Question
Problem Statement: Students have taken different tests and have scores points that are recorded in Gradebook.txt file as shown in Fig. 1. Note that students
Problem Statement:Students have taken different tests and have scores points that are recorded inGradebook.txtfile as shown in Fig. 1. Note that students may have taken different number of tests.
A program that prints students' names and their average scores as shown in Fig. 2.
Zhang: 10, 9, 10, 5, 9 Liu: 9, 10, 10 Smith: 8, 10, 10, 7, 9 Chomsky: 9, 10, 10, 8 Sharma: 10, 9, 6, 9, 8 Fig. 1: Gradebook.txt | Student Average Score ---------------------------------------- Zhang : 8.60 Liu : 9.67 Smith : 8.80 Chomsky : 9.25 Sharma : 8.40 Fig.2: Console output |
Solution Design:
You are given a class named Gradebook.java. The main() and printAverages() methods have been fully coded. You need to code the other four methods. Please refer to the comments in the code file provided for more details.
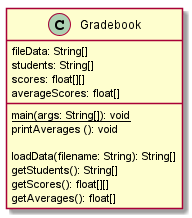
Instructions
Gradebook.java
package exam1;
import java.util.Arrays;
public class Gradebook {
String[] fileData; //stores rows of data read from data file
String[] students; //names of students
float[][] scores; //students' scores in the same order as given in data file
float[] averageScores; //each students' average score
//do not change this method
public static void main(String[] args) {
Gradebook gradebook = new Gradebook();
gradebook.fileData = gradebook.loadData("Gradebook.txt");
gradebook.students = gradebook.getStudents();
gradebook.scores = gradebook.getScores();
gradebook.averageScores = gradebook.getAverages();
gradebook.printAverages();
}
/** loadData() uses filename to read the file and
* returns an array of String, with each
* string representing one row in data file
* @param filename
* @return
*/
String[] loadData(String filename) {
// code here
return null;
}
/** getStudents() uses data in fileData array
* and extracts student's names from it. It returns
* an array of these names.
* @return
*/
String[] getStudents() {
//code here
return null;
}
/** getScores uses data stored in fileData array
* and extracts scores of each student in a 2D array of
* float numbers.
* It returns the 2D array.
* @return
*/
float[][] getScores() {
// code here
return null;
}
/** getAverages uses data stored in score[][] array
* and computes average score for each student.
* It returns an array of these averages.
* @return
*/
float[] getAverages() {
// code here
return null;
}
//do not change this method
void printAverages() {
System.out.printf("%-20s\t%s%n", "Student", "Average Score");
System.out.println("----------------------------------------");
for (int i = 0; i < fileData.length; i++) {
System.out.printf("%-20s:\t%10.2f\n", students[i] , averageScores[i] );
}
}
}
Gradebook.txt
Zhang: 10, 9, 10, 5, 9
Liu: 9, 10, 10
Smith: 8, 10, 10, 7, 9
Chomsky: 9, 10, 10, 8
Sharma: 10, 9, 6, 9, 8
Copy/import java files in package named exam1 and data file in the project folder.
Complete your code as needed
Gradebook fileData: String[] students: String[] scores: float[][] averageScores: float[] main(args: String[]): void printAverages (): void loadData(filename: String): String[] getStudents(): String[] getScores(): float[][] getAverages(): float[]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
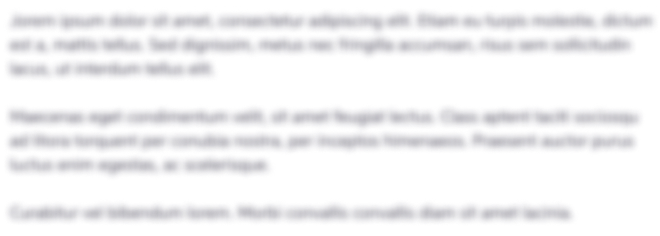
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started