Question
Problem: The town of Podunk has enrollment data for years 2005 2010 for grades K 4 in two elementary schools. The data are stored in
Problem: The town of Podunk has enrollment data for years 2005 2010 for grades K 4 in two elementary schools. The data are stored in two separate data files, School1.txt and School2.txt . Download these files from BB. Your program must: 1) read in the two data files, and store the data in 2 different and appropriate Table objects. 2) Add the enrollments for each year for each school and store in a new Table TotalEnrollment 3) Find the column sums and the row sums for TotalEnrollment 4) Write to an output file named Total.txt : The TotalEnrollment table along with the column and row sums.
In the Table class add an accessor method: get2DimArrayFromTable()method that returns the 2D array. 2) In the Table class write the following static method that will add the elements of the two tables as parameters and store in a new Table and return it. My comments are hints to write the code. (This is a static method so is not called on any object and will be called as Table.addTables(, ).) public static Table addTables (Table atable1, Table atable2) // Declare two local variables a1 and a2 to be 2Dimensional int arrays // set a1 to be the 2Dim array of atable1 // set a2 to be the 2Dim array of atable2 // declare and create a new Table object named tableSum pass appropriate parameters to the constructor of Table using length of a1 //declare a variable aSum, and set it to be 2DimArray in tableSum // add each element of a1 to a2 and store in aSum (nested for loops) //return tableSum }
f) 3) In the main method of SchoolEnrollment class: Delete any code in the main method, and do: a) declare and create three Table object variables. Use any 3 appropriate names such as: table1,table2, totalEnrollment To find out their dimensions/parameters for the Table constructor check the data in the input files School1.txt or School2.txt b) Write a method call- to store the data in School1.txt into table1 c) Write a method call- to store the data in School2.txt into table2 d) Write code using a method call to assign an appropriate value to the 3 rd table: totalEnrollment e) Write a method call to compute the row and column sums of the 3rd table and write that into an output file called Total.txt
//*********************************************************** // Table.java //*********************************************************** // Written by Edward McDowell for Computer Science 201. // modified by N. Sarawagi and A Moskol //*********************************************************** // This program reads a table of integers having rowN rows and // colN columns into a two-dimensional array. It displays the // table on the screen with row and column sums. //***********************************************************
import java.util.*; import java.io.*;
public class Table { private int[ ][ ] table; // declare table as a 2D Array private final int rowN; // number of rows private final int colN; // number of columns
public Table(int rows, int columns) { rowN = rows; colN = columns; // Create the array table = new int[rowN][colN]; } public static Table addTables (Table atable1, Table atable2){ // Declare two local variables a1 and a2 to be 2Dimensional int arrays // set a1 to be the 2Dim array of atable1 Table a1 = atable1; // set a2 to be the 2Dim array of atable2 Table a2 = atable2; // declare and create a new Table object named tableSum pass Table tableSum //appropriate parameters to the constructor of Table using length of a1 //declare a variable aSum, and set it to be 2DimArray in tableSum // add each element of a1 to a2 and store in aSum (nested for loops) //return tableSum } public int[][] get2DimArrayFromTable(){ return table; } public int [ ] rowSums () { int [ ] rowsum = new int[rowN]; // Compute the row sums. for (int row = 0; row < rowN; ++row){ // for each row: initialize the rowsum = 0; rowsum[row] = 0; //For each row: Go through each column and accumulate the sum for (int col = 0; col < colN; ++col){ rowsum[row] = rowsum[row]+table[row][col]; //rowsum[row] += table[row][col]; } } return rowsum; }
public int [ ] colSums () { int [ ] colsum = new int[colN]; // Compute the column sums. for (int col = 0; col < colN; ++col) { // for each col: initialize the colsum = 0; colsum[col] = 0; //For each clmn: Go through each row and accumulate the sum for (int row = 0; row < rowN; ++row){ colsum[col] = colsum[col]+table[row][col]; // OR colsum[col] += table[row][col]; } } return colsum; }
public void ReadTablefromFile(String fileName) throws IOException { Scanner infile; int row; int col; infile = new Scanner(new FileReader(fileName));
// Read the table one row at a time.. for (row = 0; row < rowN; ++row) for (col = 0; col < colN; ++col) table[row][col] = infile.nextInt(); infile.close(); }
public void printTableSumstoFile (String fileName) throws IOException{ PrintWriter outfile; int [ ] rowsum = rowSums(); outfile = new PrintWriter(new FileWriter(fileName)); outfile.println( ); for (int row = 0; row < rowN; ++row) { for (int col = 0; col < colN; ++col) { outfile.printf ("%4d", table[row][col]); } outfile. printf("%8d%n", rowsum[row]); } int [ ] colsum = colSums(); outfile.printf("%n"); for (int col = 0; col < colN; ++col){ outfile.printf ("%4d", colsum[col]); } outfile.printf ("%n"); outfile.close(); }
}
School1.txt School2.txt
20 35 33 42 45 16 18 22 28 30
25 30 26 36 40 22 24 17 21 25
30 27 32 28 35 24 30 22 20 20
28 34 30 34 25 23 32 28 23 18
22 39 33 38 40 25 28 33 27 24
18 28 42 37 44 19 34 30 31 27
Note: If you have done the program correctly, this is what your outputfile should have:
OutputFile: TOTAL.TXT
36 53 55 70 75 289
47 54 43 57 65 266
54 57 54 48 55 268
51 66 58 57 43 275
47 67 66 65 64 309
37 62 72 68 71 310
272 359 348 365 373
Step by Step Solution
There are 3 Steps involved in it
Step: 1
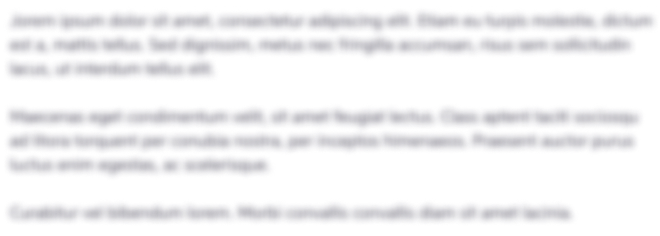
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started