Question
Problem(using Python) Very often, programs contain many functions. It can be a challenge to test and debug such programs. One way to overcome this problem
Problem(using Python)
Very often, programs contain many functions. It can be a challenge to test and debug such programs. One way to overcome this problem is to divide and conquer: test each function independently and then test the whole program.
1. The very last line in the program calls the main() function. That would mean that the entire functionality would be invoked. We dont want to do that. Either delete the call to main() or comment out the call.
2. Let us begin by testing the functionality of one of the functions.
3. Locate the get id() function. Let us test that as follows.
4. Read the documentation associated with the function. The function requires as
parameters a list and a name.
5. Create a function named test_get_id().
6. Inside the above function, create a list to satisfy the requirements of the function.
test_list = [
[id1, name 1],
[id2, name 2],
[id3, name 3],
[id4, name 4]
]
7. Let us call get_id() with test list as the first parameter and name 1 as the
second parameter. Type in the following code. result = get_id(test\_list, "name 1") print(result)
8. What should be the output corresponding to the above print statement?
9. Put in the code for getting and printing the ids for name 2 and name 4.
10. Call the function test get id() (outside the function, as the last line in the
program).
11. Verify that the output is correct. Get your instructors signature here.
12. Now develop test function for testing get names(). Verify that the function works. Show your work to your instructor. Get your instructors signature here.
13. If you have time, test the function get course id().
**************___________****************
''' This function opens the specified file and creates a list that contains every line organized as a list. It then returns the list. ''' def load(file_name): try: file = open(file_name, 'r') the_list = [] for line in file: the_list.append(line.strip(" ").split("~")) file.close() return the_list except FileNotFoundError: return None
''' The function takes in as parameters a list and a name. Each list entry is a list of strings. The first element of each list entry is the id of a student or a course. The second element of each list entry is the name of a student or a course. The function returns the id of the student or the course for the given student name or course name. ''' def get_id(a_list, name): for entry in a_list: if entry[1] == name: return entry[0] return None
''' This function takes a list as parameter. Each list entry is a list of strings. The first element of each list entry is the id of a student or a course. All the names are extracted and put in a list and the resulting list is returned. ''' def get_names(this_list): names = [] for element in this_list: names.append(element[1]) return names ''' This function takes a list and a string as parameters. The list contains one entry per section. Each section contains a section id, a corresponding course id, and the semester the section is offered. The string parameter is a section id. The function returns the course id of that section. ''' def get_course_id(sections, section_id): for entry in sections: if entry[0] == section_id: return entry[1] return None ''' This function takes a list and a string as parameters. The list contains one entry per section. Each section contains a section id, a corresponding course id, and the semester the section is offered. The string parameter is a section id. The function returns the semester name of that section. ''' def get_semester_name(sections, section_id): for entry in sections: if entry[0] == section_id: return entry[2] return None
''' This function takes a list and a section or course id as parameters. Each list entry is a list of strings. The first element of each list entry is the id of a student or a course. The second entry is the student or course name. The function returns the name of the student or the course corresponding to the student or course. ''' def get_name(this_list, some_id): for entry in this_list: if entry[0] == some_id: return entry[1] return None ''' Prints the menu ''' def print_menu(): print('Type:') print(' 1 to list all student names') print(' 2 to list all course names') print(' 3 to list course names and semesters') print(' 9 to list this menu') print(' 0 to exit') ''' Prints a list, one entry per line ''' def print_list(a_list): if a_list == None or len(a_list) == 0: print("Nothing to list") return for entry in a_list: print(entry) ''' Loads the files and accepts and processes commands. ''' def main(): students = load('students.txt') courses = load('courses.txt') sections = load('sections.txt') takes = load('takes.txt') if students == None or courses == None or sections == None or takes == None: return print_menu() command = int(input('Enter command: ')) while command != 0: if command == 1: names = get_names(students) print_list(names) elif command == 2: names = get_names(courses) print_list(names) elif command == 3: names = get_section_info(sections, courses) print_list(names) elif command == 4: student_name = input("Enter student name: ") course_info = get_students_courses(students, student_name, courses, sections, takes) print_list(course_info) elif command == 9: print_menu() command = int(input('Enter command: '))
#main() def test_get_id(): test_list = [ ['id1', 'name 1'], ['id2', 'name 2'], ['id3', 'name 3'], ['id4', 'name 4'] ] result = get_id(test_list, "name 1") print(result) result = get_id(test_list, "name 2") print(result) result = get_id(test_list, "name 4") print(result) result = get_id(test_list, "name 5") print(result)
test_get_id(
Step by Step Solution
There are 3 Steps involved in it
Step: 1
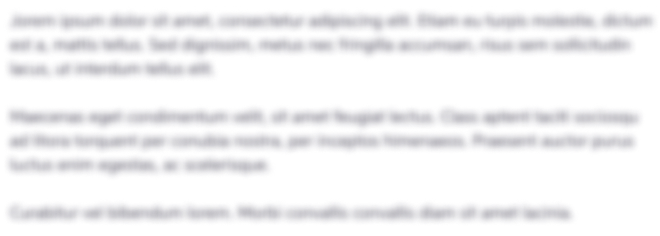
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started