Question
Processing large amounts of data can be challenging because it must fit in memory. A way of trading off is to keep the data on
Processing large amounts of data can be challenging because it must fit in memory. A way of trading off is to keep the data on disk and read it only as needed for processing, but this comes at the cost of time since disk access is slower. Write a class called IntDiskArray that abstracts reading and writing an array that is kept strictly on disk. You will support the operations as follows:
- IntDiskArray(String filename, int size). The constructor takes the name of the file and the size of the array. If the file exists then it should be opened and its size calculated (ignoring the size parameter). If the file doesnt exist, then create it and write size binary zeros to the file. Use the exists() and length() method of the File class for some of this.
- close(). Closes the array on disk.
- get(int index). Reads the data from the array at the given index. Use the seek() method for this, but remember to convert from index to byte offset. Check for bounds on the array.
- put(int index, int data). Write the data to the array at the given index. Again, use seek(). Check for bounds on the array.
A starter project and tests are provided. Paste your code for IntDiskArray as your solution here. Show a screenshot of the test results.
Replace this text with your solution.
Problem 2 Text file processing
[8 points] Binary files are convenient to computers because theyre easily machine readable. Text files are human readable, but require parsing and conversion to process in the computer.
The Java String class defines the following method to split a Java String object into multiple fragments of substrings and store them in a returned String array:
String[] split( String regularExpression)
The regularExpression argument specifies a delimiter or separator pattern. More detailed information can be found in the Java Document API (http://java.sun.com/javase/6/docs/api/). The following example uses - as a separator to split a String object:
String initialString = "1:one-2:two-3:three";
String[] fragments = initialString.split("-");
The resulting fragments array contains three Strings of 1:one, 2:two, and 3:three. One can further split these fragments if needed. For example,
String[] pair1 = fragments[0].split(":");
The pair1 array contains two String objects of 1 and one.
Given the following line in a text file:
A=Excellent B=Good C=Adequate D=Marginal E=Unacceptable
Write a method that would read this text file and print out the following:
Grade A is Excellent Grade B is Good
Grade C is Adequate
Grade D is Marginal
Grade E is Unacceptable
Step by Step Solution
There are 3 Steps involved in it
Step: 1
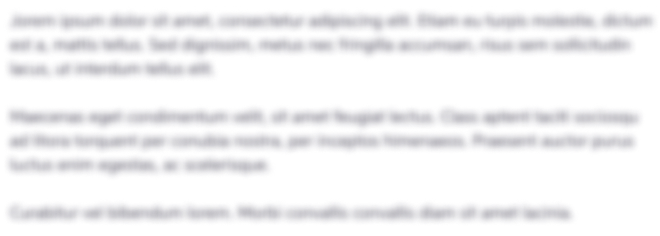
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started