Question
Program 1d: FiveCards in Java. Hello Chegg, I have been tyring to complete this lab for quite some time now but have seemed to get
Program 1d: FiveCards in Java.
Hello Chegg,
I have been tyring to complete this lab for quite some time now but have seemed to get stuck and was wodnering if I could get assistants to finsh the class. I also attached (the bottom) my own code, and was wondering if we could use it to finsh the rest of the lab.
Thank You so much! ( I always rate positively ) ..... :)
Objectives
Work with arrays that correspond to a standard poker card deck.
Use Random number generator.
Write various methods to convert index to a card suit and rank.
Assignment
Write an application called FiveCards.java that randomly selects five unique numbers from 0 to 51 which will represent individual cards in a standard 52-card four-suited poker deck of cards.
Methods
public static void main (String[] args)
Call initHand to initialize the one-dimensional "hand" of cards with 52 card deck, 5 card hand and seed 1111.
Call showHand to print the string representation of the number in the hand of cards array.
Call sameSuit to print any cards in the hand that have the same suit.
public static int[] initHand(int deckSize, int handSize, long randomGeneratorSeed)
This method returns a one-dimensional array of handSize integer values, each between 0 and deckSize.
For this program, an example of a hand array is:
Array Index | Number Value | Corresponds to String value |
---|---|---|
0 | 2 | Three of Clubs |
1 | 16 | Seven of Clubs |
2 | 21 | Nine of Diamonds |
3 | 13 | Ace of Diamonds |
4 | 46 | Eight of Spades |
This method should create a Random object seeded with randomGeneratorSeed once BEFORE any loop is entered.
Random rand = new Random(randomGeneratorSeed)
After this, rand.nextInt(deckSize) inside the loop will generate the next successive value.
Unique cards: you must handle the case where number is randomly generated that has already been used in the hand array. For example, `initHand(52, 5, 31) generates, in order, 20, 12, 20,
public static void showHand(int[] hand)
This method displays each card on one line in the order it was dealt (placed into the hand array) by calling getCardValue for each card in the array.
public static void sameSuit(int[] hand)
This method calls getCardValue to display any two or more cards having the same suit. This will be as few as two cards or as many as five. This method will require some strategy. It should be able to detect any of the following scenarios.
Two or more cards of the same suit. A hand may have more multiples of more than one suit, for example, two Hearts cards and two Clubs.
A "full house" would consist of two cards of one suit and three cards of another suit.
A "flush" would be five cards all of the same suit.
Cards should be printed in ascending rank order (sort the hand array from smallest to largest).
public static String getIdentificationString()
This method returns a string containing "Program 1, Student Name"
public static String getCardValue(int cardNumber)
This method rerturns the value of a card having the form " of ". For example, "Ace of Hearts", "Two of Diamonds", "Queen of Spades."
The rank is determined by calling getRank and suit is determined by calling getSuit.
public static String getSuit(int cardNumber)
Returns a string containing the suit of the card where
Card Value | Suit |
---|---|
0 - 12 | Clubs |
13 - 25 | Diamonds |
26 - 38 | Hearts |
39 - 51 | Spades |
public static String getRank(int cardNumber)
Returns a string containing the rank of the card where
Card Value | Rank | Card Value | Rank | Card Value | Rank | ||
---|---|---|---|---|---|---|---|
0 | Ace | 5 | Six | 10 | Jack | ||
1 | Two | 6 | Seven | 11 | Queen | ||
2 | Three | 7 | Eight | 12 | King | ||
3 | Four | 8 | Nine | ||||
4 | Five | 9 | Ten |
Notes
import java.util.Arrays to use Arrays.sort(myHandArray) if desired
import java.util.Random for random numbers
Testing
Your program should run with a variety of inputs. Test as many as you can to find unique outputs! Focus on changing the seed value. Here are some examples: 1, 8, 81, 111, 1111, 11111, 31.
// Example 1: Given the following code segment: Everyone should have this example to begin with. int[] hand1= initHand(52, 5, 1111); showHand(hand1); sameSuit(hand1); // showHand() output is: Three of Clubs Seven of Clubs Nine of Diamonds Ace of Diamonds Eight of Spades // sameSuit output is Three of Clubs Seven of Clubs Ace of Diamonds Nine of Diamonds // Example 2: Given the following code segment: int[] hand2 = initHand(52, 5, 111); showHand(hand2); sameSuit(hand2); //showHand output is Nine of Diamonds Two of Diamonds King of Diamonds Two of Clubs Ten of Clubs // SameSuit output is Two of Clubs Ten of Clubs Two of Diamonds Nine of Diamonds King of Diamonds
-----[MY OWN CODE, PLEASE USE TO FINISH THE PROBLEM, THANKS!]----
import java.util.Random; import java.util.Arrays;
public class FiveCards { public static int[] initHand(int deckSize, int handSize, long randomGeneratorSeed) { Random rand = new Random(randomGeneratorSeed); int [] cardType = new int [deckSize]; for (int i = 0; i < deckSize; i++) { cardType [i] = rand.nextInt(deckSize); } return cardType; } public static void showHand(int[] hand) { } public static String getCardValue(int cardNumber) { String toYes = "Yo"; return toYes; } public static String getSuit(int cardNumber) { String suitType = ""; if (cardNumber > 13) { suitType = "Clubs"; } if (cardNumber > 26) { suitType = "Diamonds"; } if (cardNumber > 39) { suitType ="Hearts"; } else if (cardNumber > 52) { suitType = "Spades"; } return (suitType); } public static String getRank(int cardNumber) { String rankType =""; switch (cardNumber) { case 0: rankType = "Ace"; break; case 1: rankType = "Two"; break; case 2: rankType = "Three"; break; case 3: rankType = "Four"; break; case 4: rankType = "Five"; break; case 5: rankType = "Six"; break; case 6: rankType = "Seven"; break; case 7: rankType = "Eight"; break; case 8: rankType = "nine"; break; case 9: rankType = "Ten"; break; case 10: rankType = "Jack"; break; case 11: rankType = "Queen"; break; case 12: rankType = "King"; break; } return rankType; } public static String getIdentificationString() { return "Program 1c, Eric Guevara"; } public static void main (String[] args) { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
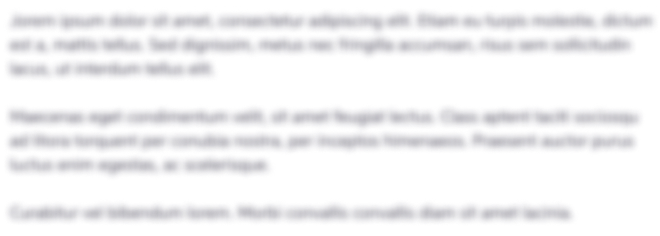
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started