Question
Program Description You will work in a team of two to write a program using Python to maintain the inventory for a company. Your program
Program Description
You will work in a team of two to write a program using Python to maintain the inventory for a
company. Your program will read and process commands from the standard input until end-of-file.
Commands consist of a single character, possibly followed by parameters.
The commands you are to implement are of the form, which one or more spaces between parameters:
A item number cost
S item number price
P
O
V type
You will maintain the data using three parallel arrays. One array is for the item descriptions, one for
the number of the item in the inventory, and the third is for the cost of the item.
1. A Add command. Read the item description, number that will be added to the inventory, and
the cost of that item. If the item already exists in the inventory, ignore the cost that was read in
and instead, add "number" to the number already in the inventory. If the item isn't already in the
inventory, then add it unless the arrays are already full. In all cases, print a message.
2. S Sell command. Read the item description, number to be sold, and the price of the item. If the
item doesn't exist in the inventory, print a message. Otherwise, if "number" is greater than or
equal to the number of that item currently in the inventory, sell all of that item in the inventory
and then delete that item from the inventory. Otherwise, reduce the inventory for that item by
"number". If items are sold, then calculate the profit from the sale and add it to the total profit.
To calculate the profit, take the number of items sold times the difference between the price and
cost.
3. P Print command. Print the inventory list and the total value of everything in the inventory.
4. O Order command. Sort the arrays by name using the Selection sort covered in class (and in the
book). There is no output for this command.
5. V Average Command. If type is 'C', then calculate and print the average cost of the inventory
items. If the type is 'I', then calculate and print the average of the number of items in the
inventory. If there are no items in the array, we'll take the average to be 0.
At the end of the program, print the total profit from all the sales.
You do not need to check for bad commands. Assume all input commands are correct. Also, assume
number, cost, and price will always be greater than 0. See the sample output for the exact wording and spacing for the output.
Requirements:
1. Declare and use the following parallel arrays.
description[MAX_ITEMS];contains strings
inventory[MAX_ITEMS]; contains integers
cost[MAX_ITEMS]; contains floats
2. You must show the floats with two decimal places.
3. You must have a Find function and use it in appropriate places. It must have the following
prototype. It must return the index where the name is found or -1 if the name isn't in the
names array. No printing is allowed in this function.
def Find(const string items[], int num, string item);
4. So that everyone's output matches the output:
a. When Adding, add it to the end of the arrays
b. When Deleting, replace the deleted data with the arrays last data. This is to replace
the "empty" cell that is created with the data from the last "good" data in the array.
5. You must use a Selection Sort for the sort. It must be the algorithm from the book, modified
for this program, not one you make up or get from the internet.
6. Both persons in the group must do their fair share of work for the program. The header block
at the top must contain each person's estimate of what percent each member contributed to the
completion of the program. The percentages must add up to one hundred. For example:
Sally Smith: Sally - 60%, J Jacobs - 40%
John Jacobs: Sally S - 50%, John - 50%
Expect to lose up to 3 points on the program if this is not done.
Students not doing their share can expect their grade lowered.
Sample Input:
V C
V I
P
S Pencil 5 1.40
O
A Pencil 10 1.16
A Pen 20 2.19
A Cheetos 10 4.18
V C
V I
P
O
P S Pencil 5 1.40
S Cheetos 15 5.00
S Dishsoap 15 3.22
P
A Pen 15 2.59
A Cheetos 20 5.64
A Dishsoap 15 2.88
A Snickers 250 1.12
A Crackers 40 3.18
A Soup 10 2.19
P
V C
V I
O
S Snickers 300 2
P
Corresponding Output:
Average cost is: $0.00
Average inventory is: 0.00
Inventory List:
Total Inventory value: $0.00
Can't sell Pencil. Not in stock.
Item Pencil added.
Item Pen added.
Item Cheetos added.
Average cost is: $2.51
Average inventory is: 13.33
Inventory List:
Pencil 10 1.16
Pen 20 2.19
Cheetos 10 4.18
Total Inventory value: $97.20
Inventory List:
Cheetos 10 4.18
Pen 20 2.19
Pencil 10 1.16
Total Inventory value: $97.20
Sold 5 of Pencil.
Only have 10 of Cheetos so all are sold. Item removed from
inventory.
Can't sell Dishsoap. Not in stock.
Inventory List:
Pencil 5 1.16
Pen 20 2.19
Total Inventory value: $49.60
15 added to item Pen.
Item Cheetos added. Item Dishsoap added.
Item Snickers added.
Item Crackers added.
Item Soup not added. No more room for items.
Inventory List:
Pencil 5 1.16
Pen 35 2.19
Cheetos 20 5.64
Dishsoap 15 2.88
Snickers 250 1.12
Crackers 40 3.18
Total Inventory value: $645.65
Average cost is: $2.69
Average inventory is: 60.83
Only have 250 of Snickers so all are sold. Item removed from
inventory.
Inventory List:
Cheetos 20 5.64
Crackers 40 3.18
Dishsoap 15 2.88
Pen 35 2.19
Pencil 5 1.16
Total Inventory value: $365.65
The Total Profit is: $229.40
Normal Termination of Program 4.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
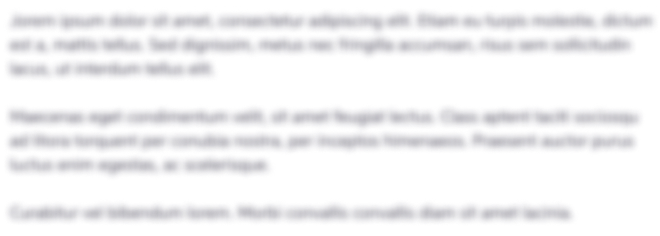
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started