Question
PROGRAM FOR C++ In this assignment, you will implement a class named bookReader which mimics the dashboard of an Electronic Bookshelf system. This dashboard allows
PROGRAM FOR C++
In this assignment, you will implement a class named bookReader which mimics the dashboard of an Electronic Bookshelf system. This dashboard allows the user to add textbook chapter titles to the bookshelf, read current chapter, move to next chapter, and move to previous chapter. This book dashboard, which can hold a book with a maximum of 10 chapters, has five operations: Log On/Off, NextChapter, PreviousChapter, ReadChapter, and AddChapter. The table below shows how each feature works.
Log On/Off | Log on to your electronic book shelf if it is off; log off if it is on. |
Next Chapter Button | If the current book chapter is not the final one in the bookshelf, move forward to the next book chapter; otherwise, the book shelf chapter stays intact. |
Previous Chapter Button | If the current book chapter is not the first one in the bookshelf, moves backward to the previous book chapter; otherwise, the bookshelf chapter stays intact. |
Read Chapter button | Displays the current book chapter title and total number of pages. |
Add Chapter button | Adds the new book chapter title to the end of the bookshelf and increments the number of pages. |
When the etext book reader is turned on the first time, it has no book chapters saved. If the etext book reader is off, pressing any button other than the On/Off button has no effect. Unlike the real etext book reader, this etext book reader receives a book chapter title and number of pages from the users input via the keyboard, reads a book by displaying the book title, chapter, and total number of pages on the screen. Furthermore, this etext book reader does not automatically read chapters saved in the etext book reader in sequence. In other words, it does not automatically read the next chapter after reading the current book chapter. To read the next chapter, the user needs to press the Next Chapter button first, then the Read button. To read the previous book chapter, the user has to press the Back Chapter button first, then the Read button.
When a button is pressed the application sends the task selected (i.e. Add, Next, Read, etc.) to the button_name, performs the proper task, then calls the display function of the class to show the result of pressing the button. Each output has three lines: The first line contains the number of book chapter titles and the number of pages loaded in the electronic textbook reader. The second line identifies which button was pressed. The third line includes information reflecting the task done.
The class bookReader below is defined based on the information described above.
#ifndef bookReader_CLASS_
#define bookReader_CLASS_
const int MAX_BOOK_CHAPTERS = 10;
const int MAX_SIZE = 15;
class bookReader
{
private:
bool logon_state; // etext book reader on/off state
string chapters[MAX_BOOK_CHAPTERS]; // holds book titles
int totalPages;
int size; // number of book chapters stored
int currentChapter; // current book chapter (from 0 to 1 < size)
char button_name[MAX_SIZE]; // holds name of the button being pressed
public:
bookReader();
void onOffPressed();
void nextChapter();
void previousChapter();
void readChapter();
void addChapter(string, int);
bool isOn();
void displayChapter();
}; // class bookReader
#endif
-
The constructor has no precondition. When a etext book reader object is created, it is off, each element in the character array or string is set to an empty string, all int values (i.e. size) set to zero, and current set to -1.
-
The onOffPressed function has no precondition. After this function is called, the etext book reader is turned off if it is on and the state of the etext book reader stays the same except it is off. On the other hand, if the etext book reader is off, it is turned on and the state of the etext book reader resumes.
-
The nextChapter function has no precondition. If the etext book reader is on and the current book chapter is not the final one, invoking this function makes the next book chapter as the current book chapter(or adds one to the member variable currentChapter). Otherwise, calling this functions has no effect.
-
The previousChapter function has no precondition. If the etext book reader is on and the current book chapter is not the first one, invoking this function makes the previous book chapter as the current book title. Otherwise, calling this functions has no effect.
-
The readChapter function has no precondition. If the etext book reader is on and at least 1 chapter has been added, invoking this function calls the display function of the class (Displays total number of chapters, the Read Chapter button is pressed, and the title of the current chapter). Otherwise, calling this functions has no effect.
-
The addPressed function has a precondition, the string passed to the function is a book chapter title and the int passed to the function is the number of pages for that chapter. If the etext book reader is on and the number of book chapters stored in the class does not exceed the maximum capacity of the array used to hold book chapter titles, the new book chapter is added to the end of the list of book chapters in the chapters array, the number of pages is added to the total pages, and the member variable size is incremented by one. Otherwise, no book title is recorded.
-
The isOn function has no precondition and returns the state of the ETextBook_Reader, either a true (on) value or a false (off) value.
-
The display function has no precondition. It shows the number of book chapters recorded, the name of the button pressed, and other related information for the task when it is invoked.
Note: When a button is pressed, its name must be copied to the member variable button_name so that the button named is displayed when the function display is called.
Write a test-driver to test the member functions of bookReader class. You need to design various test cases
header file as bookReader.h, the implementation file as bookReader.cpp and the test-driver program as testdrive.cpp.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
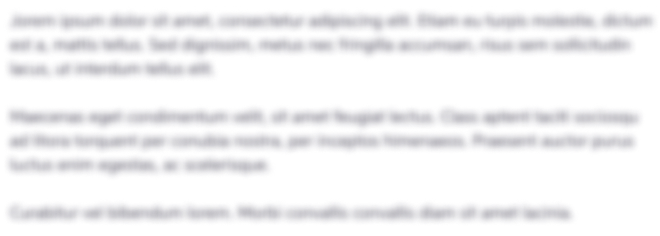
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started