Question
Program Language: C++ I Need help printing this matrix out to a output file and a data set of my own. Below are 9 files
Program Language: C++
I Need help printing this matrix out to a output file and a data set of my own. Below are 9 files file: MatrixType.cpp, Matrix Ops.cpp, MatrixOpsTest.cpp, MatrixType.h, MatrixOps.h, m1a.txt, m1b.txt, m2a.txt, m2b.txt and how it should be printed out.
How the output file should look(below):
ADD, SUBTRACT, & MULTIPLY TWO MATRICES
Programmed by xxxxx xxxx
Data Set #1
|Sp| has 5 elements
| 3.1 0.0 -4.2 |
| -5.8 0.0 0.0 |
| 0.0 2.3 0.0 |
| 0.0 0.0 9.4 |
[A] has 2 elements
[ 1.2 0.0 0.0 ]
[ 0.0 -0.6 0.0 ]
[B] has 3 elements
[ -1.2 0.0 0.0 ]
[ 0.0 -0.6 -1.0 ]
[A]+[B] has 2 elements
[ 0.0 0.0 0.0 ]
[ 0.0 -1.2 -1.0 ]
[A]-[B] has 2 elements
[ 2.4 0.0 0.0 ]
[ 0.0 0.0 1.0 ]
Dimension error. Cannot be multiplied.
[A]*[B] has 0 elements
ADD, SUBTRACT, & MULTIPLY TWO MATRICES
Programmed by xxxxx xxxx
Data Set #2
|Sp| has 5 elements
| 3.1 0.0 -4.2 |
| -5.8 0.0 0.0 |
| 0.0 2.3 0.0 |
| 0.0 0.0 9.4 |
[A] has 5 elements
[ 0.0 2.0 ]
[ 3.0 4.0 ]
[ 5.0 6.0 ]
[B] has 5 elements
[ 1.0 2.0 3.0 ]
[ 4.0 5.0 0.0 ]
Dimension error. Cannot be added.
[A]+[B] has 0 elements
Dimension error. Cannot be subtracted.
[A]-[B] has 0 elements
[A]*[B] has 8 elements
[ 8.0 10.0 0.0 ]
[ 19.0 26.0 9.0 ]
[ 29.0 40.0 15.0 ]
MatrixOps.CPP
#include
#include
#include
using namespace std;
#include "MatrixOps.h"
#include "MatrixType.h"
// class operator overload + for adding two matrices together
MatrixOps MatrixOps::operator+ (const MatrixOps & m2) const
{
float resVal1; // the value of corresponding element in matrix1.
float resVal2; // the value of corresponding element in matrix2.
float resVal3; // the value of corresponding element in matrix3.
MatrixOps sum; // instantiate a sum matrix
//Check for addition compatibility (row = row, col = col)
if(numberOfRows() != m2.numberOfRows() || numberOfCols() != m2.numberOfCols())
{
cout << "Error" << endl;
}
else //matrices are addition compatible
{
// nested loop for each corresponding element.
for(int r = 0; r < numberOfRows(); r++)
{
for(int c = 0; c < numberOfCols(); c++)
{
resVal1 = valueAt(r, c);
resVal2 = m2.valueAt(r, c);
// if val1 is NAN and val2 is a number
if(isnan(resVal1) && !isnan(resVal2))
{
resVal3 = resVal2;
}
else
{
}
sum.storeItem(r, c, resVal3);
}
}
}
return sum;
}
MatrixOpsTest.cpp
#include
#include
#include
#include
#include "MatrixOps.cpp"
// prototypes I used for the main. These are suggested, but not mandatory.
void title();
void process();
void displayMatrixOps(MatrixOps ); /// change these to pass by value, later
void displayMatrixType(MatrixType ); /// change these to pass by value, later
void heading(string, int);
void headingOps(string, int);
ifstream fin0 ("m0Sparse.txt"); // opens sparse matrix fin0
ifstream fin1 ("m1a.txt"); // opens test file matrixOpsA fin1
ifstream fin2 ("m1b.txt"); // opens test file matrixOpsB fin2
ofstream fout ("m1Out.txt"); // opens file output fout
const int SET = 1;
int main()
{
title(); // call the title function
process(); // call the process function
fin0.close(); // close input file0
fin1.close(); // close input file1
fin2.close(); // close input file2
fout.close(); // close file output
system ("pause"); // pause the output on the screen
return 0; // return all is well to the OS
}
// print the title for the program, explaining what is happening
void title()
{
cout << setw(41) << "ADD, SUBTRACT, & MULTIPLY TWO MATRICES" << " ";
/// please center the next line: Programmed by ........... (with your name)
cout << setw(36) << "Programmed by " << " ";
cout << setw(26) << "Data Set #" << SET << " ";
fout << setw(41) << "ADD, SUBTRACT, & MULTIPLY TWO MATRICES" << " ";
fout << setw(36) << "Programmed by Adam Pieroni" << " ";
fout << setw(26) << "Data Set #" << SET << " ";
}
// start the process of reading in to matrices, adding them, and displaying each
void process()
{
MatrixType m0; // instantiate sparse MatrixType
MatrixOps m1; // " " MatrixOps
MatrixOps m2; // " " MatrixOps
//m0.read(fin0);
fin0 >> m0; // read in the data into sparse matrix 0
cout << m0;
heading("|Sp|", m0.getSize()); // heading to print a short string
displayMatrixType(m0); // call display Type to pass object by value
// m1.read(fin1);
fin1 >> m1; // read in ops matrix 1
cout << m1;
heading("[A]", m1.getSize()); // heading to print a short string
displayMatrixOps(m1); // call display Ops to pass object by value
// m2.read(fin2);
fin2 >> m2; // read in ops matrix 2
cout << m2;
heading("[B]", m2.getSize()); // heading to print a short string
displayMatrixOps(m2); // call display Ops to pass object by value
MatrixOps m3 = m1 + m2; // add the matrices and assign to matrix 3
headingOps("[A]+[B]", m2.getSize()); // heading to print a short string
// displayMatrixOps(m2); // call display Ops to pass object by value
}
// displayMatrix function tests the copy constructor since matrix is passed by VALUE
void displayMatrixOps(MatrixOps m)
{
// m.print(cout); // a temporary print function
// cout << m << endl;
// fout << m << endl;
}
// displayMatrix function tests the copy constructor since matrix is passed by VALUE
void displayMatrixType(MatrixType m)
{
// m.print(cout); // a temporary print function
// cout << m << endl;
// fout << m << endl;
}
// simple heading with a variable message passed in and the $ of elements
void heading(string message, int size)
{
cout << setw(16) << message << " has " << size << " elements" << " ";
fout << setw(16) << message << " has " << size << " elements" << " ";
}
// simple heading with a variable message passed in and the $ of elements
void headingOps(string message, int size)
{
cout << setw(18) << message << " has " << size << " elements" << " ";
fout << setw(18) << message << " has " << size << " elements" << " ";
}
MatrixType.cpp
#include "MatrixType.h" // include class specifications
#include
// constructor with two default parameters
MatrixType::MatrixType(int rows, int cols)
{
myRows = rows; // set the rows
myCols = cols; // set the columns
mySize = 0; // initially, since # is unknown
}
// destructor for MatrixType
MatrixType::~MatrixType()
{
cout << "MatrixType destroyed" << endl << endl;
}
// copy constructor for MatrixType
MatrixType::MatrixType(const MatrixType& otherObject)
{
myRows = otherObject.myRows;
myCols = otherObject.myCols;
mySize = otherObject.mySize;
for (int i = 0; i < mySize; i++)
{
myMatrix[i].col = otherObject.myMatrix[i].col;
myMatrix[i].row = otherObject.myMatrix[i].row;
myMatrix[i].value = otherObject.myMatrix[i].value;
}
}
void MatrixType::setRowAndColumns(int r, int c) // client sets number of rows & cols
{
myRows = r; // assign r to number of rows
myCols = c; // assign c to number of cols
}
void MatrixType::storeItem(int r, int c, float val) // stores the item within myMatrix
{
oneItem item; // item is a local object (node)
item.row = r; // assign the r to the row number
item.col = c; // assign the c to the col number
item.value = val; // assign the val to the value
myMatrix[mySize] = item; // assign the node to the nth element
mySize++; // increment # of elements by one
}
int MatrixType::getSize() const
{
return mySize; // " " " "
}
int MatrixType::numberOfRows() const // client access to # of rows
{
return myRows; // return the private myRows
}
int MatrixType::numberOfCols() const // client access to # of cols
{
return myCols; // return the private myCols
}
float MatrixType::valueAt(int r, int c) const // returns the value at r, j if exists
{
for(int index = 0; index < mySize; index++) // linear search if r and c are present
{
// check each r and c to see if the element exists. Returns NaN if it is not present
if(myMatrix[index].row == r && myMatrix[index].col == c)
return myMatrix[index].value; // return the matrix value or NaN
}
}
// A temporary read function leading to the overloaded operator that is used.
// Do not use this function. It should be removed for the final extraction operator function
void MatrixType::read(istream & in)
{
int sigRows, sigCols; // declare local variables to be read in
int rowIn, colIn; // "
float valueIn; // "
in >> sigRows >> sigCols; // first read in significant # rows and # cols
setRowAndColumns(sigRows, sigCols); // sets sinificant rows & cols in the class
in >> rowIn >> colIn >> valueIn; // priming read 1st sparse data (prime the pump)
while (!in.eof()) // loop until data is used (end of the file)
{
storeItem(rowIn, colIn, valueIn); // stores the file data into each node
in >> rowIn >> colIn >> valueIn; // read in the remaining sparse data for each item
}
}
// A temporary print function leading to the overloaded operator that is used
// Do not use this function. It should be removed in the final insertion operator function
//void MatrixType::print(ostream & out)
//{
// out << setprecision(1) << fixed << showpoint; // formatting for the output
// for(int row = 0; row < numberOfRows(); row++)
// {
// out << setw(11) << "|";
// for(int col = 0; col < numberOfCols(); col++)
// {
// if(isnan(valueAt(row, col) )) // if the value is NaN
// {
// out << setw(6) << 0.0; // print a 0.0
// }
// else // otherwise,
// {
// out << setw(6) << valueAt(row, col); // output the actual value
// }
// }
// out << setw(4) << "|" << endl; // new line for the next row
// }
// out << endl << endl; // skip 2 lines between matrices
//
//}
ostream & operator << (ostream & out, MatrixType& matrix)
{
out << setprecision(1) << fixed << showpoint; // formatting for the output
for(int row = 0; row < matrix.numberOfRows(); row++)
{
out << setw(11) << "|";
for(int col = 0; col < matrix.numberOfCols(); col++)
{
if(isnan(matrix.valueAt(row, col) )) // if the value is NaN
{
out << setw(6) << 0.0; // print a 0.0
}
else // otherwise,
{
out << setw(6) << matrix.valueAt(row, col); // output the actual value
}
}
out << setw(4) << "|" << endl; // new line for the next row
}
out << endl << endl; // skip 2 lines between matrices
return out;
}
istream & operator >> (istream & in, MatrixType& matrix)
{
int sigRows, sigCols; // declare local variables to be read in
int rowIn, colIn; // "
float valueIn; // "
in >> sigRows >> sigCols; // first read in significant # rows and # cols
matrix.setRowAndColumns(sigRows, sigCols); // sets sinificant rows & cols in the class
in >> rowIn >> colIn >> valueIn; // priming read 1st sparse data (prime the pump)
while (!in.eof()) // loop until data is used (end of the file)
{
matrix.storeItem(rowIn, colIn, valueIn); // stores the file data into each node
in >> rowIn >> colIn >> valueIn; // read in the remaining sparse data for each item
}
return in;
}
MatrixOps.h
#ifndef MATRIX_OPS_H
#define MATRIX_OPS_H
#include
#include
using namespace std;
#include "MatrixType.cpp"
class MatrixOps : public MatrixType // this indicates public inheritance
{
// overriding (redefining) the insertion stream operator of the base class
friend ostream & operator << (ostream & outfile, const MatrixType & matrix);
public:
// No constructor necessary. MatrixOps uses MatrixType base class constructor
MatrixOps operator+ (const MatrixOps & ) const; // class overloaded + operator
MatrixOps operator- (const MatrixOps & ) const; // class overloaded - operator
MatrixOps operator* (const MatrixOps & ) const; // class overloaded * operator
};
#endif // MATRIX_OPS_H
MatrixType.h
#ifndef MATRIXTYPE_H
#define MATRIXTYPE_H
using namespace std;
struct oneItem // struct contains each array member
{
int row; // corresponds to the 2D row number
int col; // corresponds to the 2D column number
float value; // corresponds to the float value of member
};
const int MAXSIZE = 225; // maximum # of elements in the sparse array
class MatrixType
{
/// overloaded friend functions for stream operators in MatrixType
friend istream & operator >> (istream & infile, MatrixType& matrix);
friend ostream & operator << (ostream & outfile, const MatrixType & matrix);
public:
MatrixType(int r = 0, int c = 0); /// 2 default param. constructor rows & cols
~MatrixType(); /// destructor for the matrix
MatrixType(const MatrixType& otherObject); /// copy constructor to make a deep copy
void setRowAndColumns (int r, int c); // sets rowsFilled & columnsFilled to r and c
float valueAt (int r, int c) const; // yields value at row and col of myMatrix
void storeItem (int r, int c, float value); // assigns value at row, col, & changes length
int numberOfRows() const; // yields rowsFilled
int numberOfCols() const; // yields columnsFilled
int getSize() const; /// yields # of elements in sparse matrix
void print(ostream & ); /// a temporary function. Remove when << done.
void read(istream & ); /// a temporary function. Remove when >> done.
private:
oneItem myMatrix [MAXSIZE]; // complete assigned 1D array in sparse format
int myRows; // significant rows of full matrixhttp://www.jeffgold.net/TCC/CSC210/m2a.txt
int myCols; // significant columns of full matrix
int mySize; // total number of elements in the matrix
};
#endif // MATRIXTYPE_H
M1a.txt
2 30 0 1.21 1 -0.6
M1b.txt
2 30 0 -1.21 1 -0.61 2 -1.0
M2a.txt
3 20 1 2.1 0 3.1 1 4.2 0 5.2 1 6.
M2b.txt
2 30 0 1.0 1 2.0 2 3.1 0 4.1 1 5.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
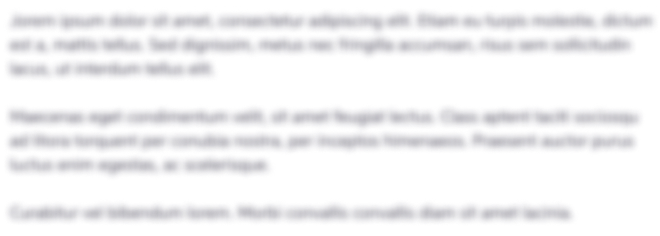
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started