Question
# Program: mlQuadratic.asm # Author: ********** # Date: mm/dd/yy # Purpose: Practice floating point calculations #---------------------------------------------------------------- # Create assembler for the Quadratic solving # #include
# Program: mlQuadratic.asm # Author: ********** # Date: mm/dd/yy # Purpose: Practice floating point calculations #---------------------------------------------------------------- # Create assembler for the Quadratic solving # #include
.data
.eqv SYS_PRINT_FLOAT 2 #float .eqv SYS_PRINT_TEXT 4 #text (zero terminated) .eqv SYS_EXIT 10 #terminate endl: .asciiz " " lblRoot1: .asciiz "Root1:\t" lblRoot2: .asciiz "Root2:\t"
a: .float 2.0 b: .float -8.0 c: .float -24.0 mytwo: .float 2.0 myfour: .float 4.0
.text .globl main main: # Get Determinate: b^2 - 4ac into $f0
#get square root jal sqrt #f0 input; f1 output
# plus root in $f0 #-b + sqrt(b^2 - 4ac) # /2a # minus root in $f1 #-b - sqrt(b^2 - 4ac) # /2a
# PRINT root1 in $f0 li $v0, SYS_PRINT_TEXT la $a0, lblRoot1 #label syscall li $v0, SYS_PRINT_FLOAT #print mov.s $f12, $f0 syscall li $v0, SYS_PRINT_TEXT la $a0, endl #endl syscall # PRINT root2 in f1 li $v0, SYS_PRINT_TEXT la $a0, lblRoot2 #label syscall li $v0, SYS_PRINT_FLOAT #print mov.s $f12, $f1 syscall li $v0, SYS_PRINT_TEXT la $a0, endl #endl syscall
#------------------------ # terminate program. li $v0, SYS_EXIT # call code for terminate syscall # system call #.end main
## =============================================================================================== ## David Krawchuk ## 11/18/2013 ## SquareRoots ## Function name : sqrt ## This function calculates square roots. The function takes one parameter in register $f0 ## and returns the calculated value in register $f1 when finished. If given a negative ## value then the function will print an error message and then return a value 0 in register $f1.
.data zero: .float 0.0 # Constant value zero one: .float 1.0 # Constant value one two: .float 2.0 # Constant value two error_statement: .asciiz " Input value is negative! " # used when input value is negative.
.globl sqrt .text sqrt:
## Negative Condition Test Block ## ## Used Registers : ## $f0 = function input ## $f31 = zero (const.) l.s $f31, zero # Load register $f31 with value zero constant for comparison to input. c.lt.s $f0, $f31 # Compare input paragmeter with zero constant; if negative set flag to 1 (1 = False). bc1t error # Break to ERROR BLOCK of function if flag value is 1; else continue to Base Calculation Block. ## End N.C.T.B.##
## Base Calculation Block ## # Used registers : # $f30 = one (const.) # $f29 = two (const.) # $f2 = calculated result # $f0 = input parameter l.s $f30, one # Set register $f30 to the float value 1.0. l.s $f29, two # Set register $f29 to the float value 2.0. add.s $f2, $f0, $f30 # Represents (input + 1) div.s $f2, $f2, $f29 # Represents (input + 1) / 2 ## End B.C.B.##
## Approximation Block ## # Used Registers : # $t0 = loop counter # $f3 = result of Xsub(i - 1) / 2 # $f2 = Xsub(i-1) # $f29 = 2 # $f4 = 2 * Xsub(i - 1) # $f5 = input / ($f4) li $t0, 1 # Set counter register $t0 to the value one; used for loop constraint.
approximation_loop: beq $t0, 10, end_approximation_loop # Break to end of loop after nine itterations of loop. # Note: first itteration performed in Base Calculation Block.
div.s $f3, $f2, $f29 # Calculates Xsub(i - 1) / 2; places results in $f3. mul.s $f4, $f29, $f2 # Calculates 2 * Xsub(i - 1) div.s $f5, $f0, $f4 # Calculates INPUT / $f4 add.s $f2, $f3, $f5 # Calculates $f3 + $f5; Xsub(i-1) / 2 + (2 * Xsub(i - 1)
addi $t0, $t0, 1 # Add one to the loop counter.
j approximation_loop # Return to the begenning of the approximation_loop.
end_approximation_loop: ## End Approximation Block ##
mov.s $f1, $f2 # Move calculated contents from appoximation loop from $f2 to return register $f1.
jr $ra # Return to caller address.
sqrt_end: ## END FUNCTION BODY ##
## ERROR BLOCK ## error: l.s $f0, zero # Set return register $f0 to value 0.
li $v0, 4 # Load instruction 4 into $v0; value 4 : print string instruction. la $a0, error_statement # Load address of string statement. syscall # Perform system call.
jr $ra # Return to caller address. error_end: ## END ERROR BLOCK ##
Step by Step Solution
There are 3 Steps involved in it
Step: 1
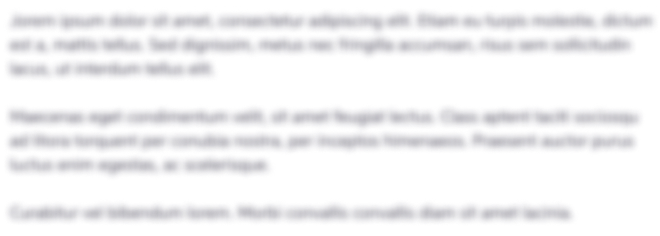
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started