Question
PROGRAM MUST COMPILE IN JBACI Modify the given code while following the guidelines to produce results without any deadlocks and correct declaration of semaphores. 1.-
PROGRAM MUST COMPILE IN JBACI
Modify the given code while following the guidelines to produce results without any deadlocks and correct declaration of semaphores.
1.- In this assignment you will implement a simulation of the interaction of user programs with the OS to execute an I/O operation on two different devices.
User programs:
User programs will communicate with DOIO (OS) to request an I/O operation. (This will simulate a system call)
User programs will give to DOIO three parameters: User id, device number (dev is a random number in the range 1 and 2 that represents device one or device two) and an address (addr is a random number in the range 1 and 20.) (addr is an integer that represents a track number in the hard drive).
User programs will pass the parameters to DOIO through three buffers of size one each (bufid, bufdev, and bufaddr).
Once the parameters are stored in the buffers, user programs executes a p(request_served) operation to wait for the completion of the I/O operation. You will need a semaphore array request_served[5].
There will be five users running concurrently and each will execute 5 I/O operations.
DOIO:
DOIO will collect and id, device(dev), and address(addr) from bufid, bufdev, and bufaddr to assemble the IORB.
DOIO will check on device number to decide which one of the two devices will get the IORB.
Once the device has been selected, DOIO will store the IORB (id and addr) into the two buffers that represent the IORQ (iorqid and iorqaddr) of the selected device. Notice that you need separate buffers (one for each device).
Device drivers (1 and 2):
Device drivers will collect an IORB (made up of id and addr) from iorqid and iorqaddr and then initiate the physical I/O operation on the hard drive it controls and wait for the I/O operation to be completed: p(operation_complete).
The device driver initiates the physical I/O operation by storing addr into a buffer of length one. The buffer name is pio (physical I/O).
When the I/O operation completes a signal is received, the Device driver will identify the User that issued the I/O request using the id, and will signal the semaphore request_served associated to the User.
Disk (1 and 2):
The Disk processes simulates the access to a track in the hard drive.
The Disk process gets the addr from pio and stores it in a variable called seek and iterates in a dummy loop from 1 to seek.
Once out of the loop, disk will execute a v on the semaphore operation_complete
Define all semaphores that you need according to the number of buffers used.
Each user will make 5 system calls to initiate I/O operations
DOIO will create 25 IORB
The sum of the I/O operations executed by drivers must add up 25. You will need a shared variable to control the total number of I/O operations because it is not known before hand the numbers of I/O operations initiated by each Device driver.
Project Direction
You will write the program C-- based on the BACI interpreter that you used in programming project 1.
Example for the print out of results:
User 1 executes system call SIO or DOIO
DOIO assembles IORB and inserts it in IORQ for user 1
User 2 executes system call SIO or DOIO
Driver initiates I/O operation for user 1
User 3 executes system call SIO or DOIO
User 4 executes system call SIO or DOIO
DOIO assembles IORB and inserts it in IORQ for user 2
Disk Completes I/O operation (disk does not know what process initiated the I/O operation)
Driver signal user 1 (operation complete)
User 1 executes system call SIO or DOIO
DOIO assembles IORB and inserts it in IORQ
And so on
//declaring constant variables const int operations = 25; const int size = 1; const int users = 5; //buffers for user program int bufid[size]; int bufaddr[size]; int bufdev[size]; int userid; int addr; int dev; //buffers for DOIO if Driver 1 int iorqid1[size]; int iorqaddr1[size]; //buffers for DOIO if Driver 2 int iorqid2[size]; int iorqaddr2[size]; //buffer for Driver 1 int daddr1[size]; int did1; int c = 0; //buffer for Driver 2 int daddr2[size]; int did2; //variable for Disk 1 int seek1 = 0; //variable for Disk 2 int seek2 = 0; //declarations of semaphores semaphore full1; semaphore full2; semaphore full3; semaphore full4; semaphore full5; binarysem mutex1; binarysem mutex2; binarysem mutex3; binarysem mutex4; binarysem mutex5; semaphore print; semaphore reqservice[5]; semaphore ioreq; semaphore reqpend1; semaphore reqpend2; semaphore physid1; semaphore physid2; semaphore opcomplete1; semaphore opcomplete2; //functions void User(int uid) { int m = 0; int i; for(i = 0; i < users; i++) { addr = random(20); dev = random(2); p(full1); p(mutex1); p(print); cout << "User " << uid << " executes system call SIO or DOIO." << endl; v(print); bufid[m] = uid; bufaddr[m] = addr; bufdev[m] = dev; m++; if(m == size){ m = 0; } v(mutex1); v(ioreq); p(reqservice[userid]); } } void DOIO() { int kid; int kaddr; int kdev; int i; int k = 0; for(i = 0; i < operations; i++) { //consumer p(ioreq); p(mutex1); kid = bufid[k]; kaddr = bufaddr[k]; kdev = bufdev[k]; p(print); cout << "DOIO assembles IORB and inserts it in IORQ." << endl; v(print); v(mutex1); v(full1); //producer if(kdev == 1) { p(full2); p(mutex2); iorqid1[k] = kid; iorqaddr1[k] = kaddr; k++; if(k == size){ k = 0; } v(mutex2); v(reqpend1); } else { p(full3); p(mutex3); iorqid2[k] = kid; iorqaddr2[k] = kaddr; k++; if(k == size){ k = 0; } v(mutex3); v(reqpend2); } } } void Driver1() { int tmpid; int tmpaddr; int i; int m = 0; for(i = 0; i < operations; i++) { did1 = iorqid1[m]; //consumer p(reqpend1); if(c == 25){ v(reqpend1); break; } p(mutex2); p(print); cout << "Driver initiates I/O operation for user " << did1 << "." << endl; v(print); tmpid = iorqid1[m]; tmpaddr = iorqaddr1[m]; m++; if(m == size){ m = 0; } v(mutex2); v(full2); c++; if(c == 25){ v(reqpend1); break; } //producer p(full4); p(mutex4); daddr1[m] = tmpaddr; p(print); cout << "Driver signal User " << did1 << " (operation complete)." << endl; v(print); v(mutex4); v(physid1); p(opcomplete1); v(reqservice[did1]); } } void Driver2() { int tmpid; int tmpaddr; int i; int m = 0; for(i = 0; i < operations; i++) { did2 = iorqid2[m]; //consumer p(reqpend2); if(c == 25){ v(reqpend2); break; } p(mutex3); p(print); cout << "Driver initiates I/O operation for user " << did2 << "." << endl; v(print); tmpid = iorqid2[m]; tmpaddr = iorqaddr2[m]; m++; if(m == size){ m = 0; } v(mutex3); v(full3); c++; if(c == 25){ v(reqpend2); break; } //producer p(full5); p(mutex5); daddr2[m] = tmpaddr; p(print); cout << "Driver signal User " << did2 << " (operation complete)." << endl; v(print); v(mutex5); v(physid2); p(opcomplete2); v(reqservice[did2]); } } void Disk1() { int i, n; int m = 0; for(i = 0; i < operations; i++) { p(physid1); p(mutex4); seek1 = daddr1[m]; p(print); cout << "Disk completes I/O operation (disk does not know what process initiated the I/O operation)." << endl; v(print); v(mutex4); v(full4); for(n = 0; n < seek1; n++) v(opcomplete1); } } void Disk2() { int i, n; int m = 0; for(i = 0; i < operations; i++) { p(physid2); p(mutex5); seek2 = daddr2[m]; p(print); cout << "Disk completes I/O operation (disk does not know what process initiated the I/O operation)." << endl; v(print); v(mutex5); v(full5); for(n = 0; n < seek2; n++) v(opcomplete2); } } main() { //declaring semaphore array int u; for(u = 0; u < users; u++) { initialsem (reqservice[u], 0); } initialsem (print, 1); initialsem (full1, 1); initialsem (full2, 5); initialsem (full3, 1); initialsem (full4, 1); initialsem (full5, 1); initialsem (mutex1, 1); initialsem (mutex2, 1); initialsem (mutex3, 1); initialsem (mutex4, 1); initialsem (mutex5, 1); initialsem (ioreq, 0); initialsem (reqpend1, 0); initialsem (reqpend2, 0); initialsem (physid1, 0); initialsem (physid2, 0); initialsem (opcomplete1, 0); initialsem (opcomplete2, 0); cobegin { User(0); User(1); User(2); User(3); User(4); DOIO(); Driver1(); Driver2(); Disk1(); Disk2(); } } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
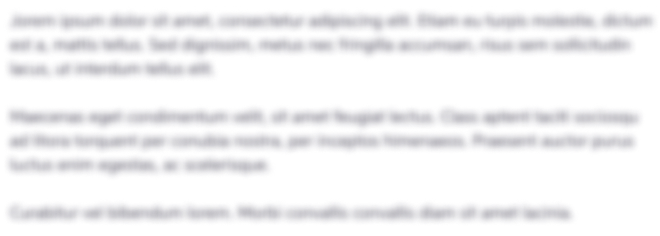
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started