Question
program must prompt the user to enter a string. The program must then test the string entered by the user to determine whether it is
program must prompt the user to enter a string. The program must then test the string entered by the user to determine whether it is a palindrome. A palindrome is a string that reads the same backwards and forwards, such as "radar", "racecar", and "able was I ere I saw elba". It is customary to ignore spaces, punctuation, and capitalization when looking for palindromes. For example, "A man, a plan, a canal. Panama!" is considered to be a palindrome.
To determine whether a string is a palindrome, you can: convert the string to lower case; remove any non-letter characters from the string; and compare the resulting string with the reverse of the same string. If they are equal, then the original string is considered to be a palindrome.
Here's the code for computing the reverse of str:
String reverse; int i; reverse = ""; for (i = str.length() - 1; i >= 0; i--) { reverse = reverse + str.charAt(i); }
As part of the program, writea static subroutine that finds the reverse of a string. The subroutine should have one parameter of type String and a return value of type String.
must also writea second subroutine that takes a String as a parameter and returns a String. This subroutine should make a new string that is a copy of the parameter string, except that all the non-letters have been omitted. The new string should be returned as the value of the subroutine. can tell that a character, ch is a lower-case letter by testing if(ch >= 'a' && ch <= 'z')
(Note that for the operation of converting str to lower case, can simply use the built-intoLowerCasesubroutine by saying:str = str.toLowerCase();)
You should writea descriptive comment before each subroutine, saying what it does.
Finally, writeamain()routine that will read in a string from the user and determine whether or not it is a palindrome. The main routine should use The program should print the string converted to lower case and stripped of any non-letter characters. Then it should print the reverse string. Finally, it should say whether the string is a palindrome. (Use the two subroutines to process the user's string.) For example, if the user's input is "Hello World!", then the output might be:
stripped: helloworld reversed: dlrowolleh This is NOT a palindrome. and if the input is "Campus motto: Bottoms up, Mac!", the output might be: stripped: campusmottobottomsupmac reversed: campusmottobottomsupmac This IS a palindrome.
i must complete the program, test, debug, and execute it. i should test two different cases, one that is a palindrome and another one that is not a palindrome. i must submit y java code file (preferably by cut and paste the code into the program dialog box). The output of program must be captured by either copying the content in the output window and pasting it into the program dialog box or by capturing the image of the screen which contains the output of java program or the output and submitting this with program as an attachment. In windows i can capture a screen shot with the Ctrl Alt and Print Screen key sequence. This image can then be pasted into a Word, WordPad, or OpenOffice document which can be submitted with program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
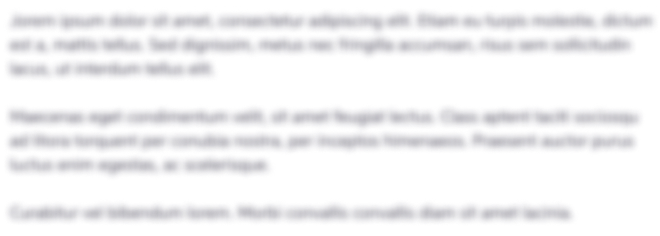
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started