Question
Program: Postfix Calculator In this program, you will write a command line calculator that can evaluate simple mathematical expressions on typed in postfix notation (also
Program: Postfix Calculator In this program, you will write a command line calculator that can evaluate simple mathematical expressions on typed in postfix notation (also called reverse polish notation, or RPN), as well as store variables for later use in other expressions. Postfix notation: In a typical mathematical expression that you are probably used to, such as 5 + 2, the operator (+) goes in-between the two operands (5 and 2). This is called "infix" notation. Postfix notation places the two operands first, and the + sign last, giving 5 2 +. One of the main benefits of postfix notation is that it is easy to evaluate using a stack. Parentheses are not needed, nor are precedence rules, since there is only one reasonable way to evaluate the expression. The algorithm to do this uses a stack of numbers, and can be stated in a few steps as follows: Given a Postfix expression such as "2 3 + x *", break it into tokens, and initialize an empty stack of numbers. Then do the following steps: 1. Process the tokens from left to right. For each token, If the token is an operand (a number or variable), push the value of the operand on the stack (of doubles in our case). For an operator or function (+, *, sin), pop the needed number of operands off the stack, compute the result, and push it back on the stack. 2. The expression's value will be on the top of the stack at the end. Error checking is also easy. The following are error conditions: 1. If at any point there are not enough operands for an operation on the stack, the postfix expression is not valid. 2. If more than one value is on the stack at the end, the expression is not valid. Memory: Your calculator must have a memory that allows it to store or modify named variables. These can easily be stored in a HashMap, or parallel ArrayLists. Program Operation: When the program starts, it should print your name and state that it is an RPN calculator. Then it should go into "command mode", showing a prompt character (in our case, >), and responding to inputs typed by the user. Example usage is given as follows. java PostfixCalc Integer postfix calculator with memory by > a = 3 5 + 1 - 7.0000 > bee = 7.0 a * 49.0000 > var { a:7.0000, bee:49.0000 } > bee sqrt 7.0000 > 3 3 3 + Error! Not enough operators. > 3 3 ^ 27.0000 > bee 49.0000 > a = 4 4.0 > d = 3.14159265 cos -1.0000 > a 3 / 1.3333 > 1 2 3 4 + + - -8.0000 > clear All variables removed > about Postfix calculator by Operators and functions: + - * / ^ sin cos tan sqrt Commands: var clear quit about > var { } > quit The calculator must accept the following input types from the user: 1. A command (quit, clear, var, about) Result: The program executes the command quit exits the program clear removes all variables var prints all the variable names and values in the calculator's memory about describes the program, lists all commands, prints your name 2. A postfix expression (example: a b + 3.1 / sqrt) Result: The value of the expression is computed and printed on the screen. ? Operands can either be numbers or variable names ? If an operand is a number, it can be positive or negative ? If a variable name is not in the memory, you must state that the expression is not valid. ? Operators and functions can be any of +, -, *, /, ^, sqrt, sin, cos, tan. ? Spaces will separate operands and operators. Thus "ab+" is not valid but "a b +" is valid. 3. An assignment (varName = postfixExpression) Example (myVar = a b + 33 *) Result: If the variable on the left does not exist, it is created. The variable on the left is then assigned the value of the right hand side. If a variable on the right hand side does not exist, it should be created and its value set to 0. Note that if the input is not a command, it must either be (1) a postfix expression, or (2) a variable name, followed by =, followed by a postfix expression. If the input is any of these three types, the program should respond properly. If the input is not valid the program must not crash. Instead, it should print an error stating that the input is not valid and continue. Things you will learn: Reading input from the keyboard Simple parsing of strings Working with stacks Evaluating postfix expressions Distinguishing between different input formats and responding to them Storing and retrieving values based on names Substituting variable names with their values Directions: 1. Write a postfix calculator as described above. 2. Make sure that your program prints your name when it begins, and that it has the appropriate block comment with your name and section at the top of the code. 3. Practice defensive programming. Your program should not crash if unknown variable names, or invalid expressions are typed in. Instead, it should print an appropriate error message and continue. 4. Make sure your program compiles and runs from the command line. 5. Make sure your program follows the class coding style, including indentation, variable naming, etc. 6. Turn in your program to the D2L dropbox for program 6, and pass it off directly to a TA or instructor.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
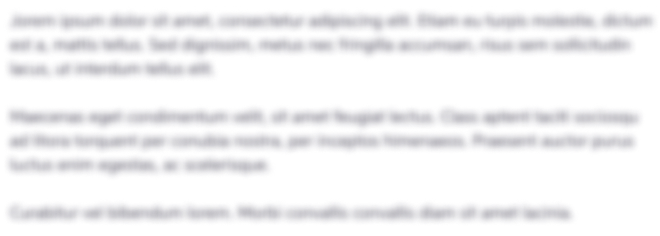
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started