Question
***Program should be in basic java. The problems refrenced to create this program are from Building Java Programs, 3rd edition. Chapter 8: Exercises #11, #12,
***Program should be in basic java. The problems refrenced to create this program are from Building Java Programs, 3rd edition. Chapter 8: Exercises #11, #12, and #13, page 568 30 points You are to incorporate all requirements of the three exercises into the basic class definition provided in the Exercise #11 description. In addition, you must check the values of deposit, withdrawal, and transfer amounts to ensure they are greater than 0. For two of the three methods, deposit and withdraw, the methods are to return a booleanvalue to indicate if the transaction was completed. For method transfer, the method is to RETurn the allowed amount transferred, refer to Exercise #13 for details for a partial transfer. In addition to the specified class methods, provide a method name() that returns a String reference to the objects account name. To start this project, create an Eclipse project and copy the two supplied source code files, Project9_BankAccount.java and BankAccount.java into the projects src folder. File BankAccount.java has the class definition shown in the text as your starting point; note that methods deposit and withdraw are incomplete! You must define all required methods in this file. You should not modify the Project9_BankAccount.java file as it provides a means to test your code. I will utilize this same test program to verify the functionality of the BankAccount class source code file you submit. Your code must work as shown with my test program! A sample Console output from this program is shown below. Code method toString() to produce the output shown for the class objects in the following Sample Console Output. Project #8 Solution Chapter 8, Exercise #11, page 568 Example #1: Account object instantiated with name only Beginning account: John Doe, $0.00, Total fees: $0.00 Depositing: $51.75 John Doe, $51.75, Total fees: $0.00 Withdrawing: $7.96 John Doe, $43.79, Total fees: $0.00 Depositing: $14.78 John Doe, $58.57, Total fees: $0.00 Depositing: $-4.59 Unable to deposit $-4.59 Withdrawing: $45.79 John Doe, $12.78, Total fees: $0.00 Withdrawing: $-3.57 Unable to withdraw $-3.57 Withdrawing: $12.78 John Doe, $0.00, Total fees: $0.00 Withdrawing: $0.01 Unable to withdraw $0.01 Example #2: Account object instantiated with name and beginning balance: $23.67 Beginning account: Sarah Jones, $23.67, Total fees: $0.00 Depositing: $51.75 Sarah Jones, $75.42, Total fees: $0.00 Withdrawing: $7.96 Sarah Jones, $67.46, Total fees: $0.00 Example #3: Account object instantiated with name, beginning balance: $34.57, and transaction fee: $2.50 Beginning account: Samuel Johnson, $34.57, Total fees: $0.00 Depositing: $51.75 Samuel Johnson, $86.32, Total fees: $0.00 Withdrawing: $7.96 Samuel Johnson, $75.86, Total fees: $2.50 Withdrawing: $75.86 Unable to withdraw $75.86 Example #4: Transfers between two accounts myAccount: Sarah Jones, $67.46, Total fees: $0.00 yourAccount: Samuel Johnson, $75.86, Total fees: $2.50 Transfering $41.38 from Sarah Jones's account to Samuel Johnson's account Source Account: Sarah Jones, $21.08, Total fees: $5.00 Amount Transferred: 41.38 Destination Account: Samuel Johnson, $117.24, Total fees: $2.50 Transfering $-8.79 from Sarah Jones's account to Samuel Johnson's account Unable to transfer $-8.79 Transfering $78.67 from Samuel Johnson's account to Sarah Jones's account Source Account: Samuel Johnson, $33.57, Total fees: $7.50 Amount Transferred: 78.67 Destination Account: Sarah Jones, $99.75, Total fees: $5.00 Transfering $94.74 from Sarah Jones's account to Samuel Johnson's account Source Account: Sarah Jones, $0.01, Total fees: $10.00 Amount Transferred: 94.74 Destination Account: Samuel Johnson, $128.31, Total fees: $7.50 Transfering $0.01 from Sarah Jones's account to Samuel Johnson's account Unable to transfer $0.01 Transfering $150.0 from Samuel Johnson's account to Sarah Jones's account Source Account: Samuel Johnson, $0.00, Total fees: $12.50 Amount Transferred: 123.31 Destination Account: Sarah Jones, $123.32, Total fees: $10.00 (spacing not completely accurate)
required docs
BankAccount.java
/****************************************************************************** * Class: BankAccount * Super Class: None ("Object" inferred) * Implements: None * * Programmer: Your Name * * Revision Date Release Comment * -------- ---------- ------------------------------------------------------ * 1.0 03/xx/2017 Initial Release * * * Class Description * ----------------- * **** You MUST provide a Class Description here **** * * * ----------------------------- Public Interface ----------------------------- * Method Description * ------------- ------------------------------------------------------------- * deposit Deposit funds to the account * withdraw Withdraw cash from the account. * transfer Transfer anount from invoking BankAccount object to another * BankAccount object * * * ------------------------- Private Data Members ----------------------------- * Data * Type Type Name Description * ------------ ------ --------------- ------------------------------------- * Non-static String name Account holder's name * Non-static double balance Current account balance * * * ------------------------- Private "Helper" Methods ------------------------- * Method Description * ------------- ------------------------------------------------------------- * ****************************************************************************** * * Imported Packages * -----------------*/ // None
public class BankAccount { // Public Interface // 1. Constructors // Add your Constructor(s) here......
// 2. Mutator Methods // A. boolean deposit(double) // Note: This method enforces the class invariant that stipulates // that a deposit amount MUST be > $0.00 public boolean deposit(double depositAmount) { boolean depositMade = false; // Insert your code here. Remember, you MUST enforce the invariant condition!!! return depositMade; } // // B. boolean withdraw(double) // Note: This method enforces the class invariant that stipulates // that the ending account balance MUST be >= $0.00 public boolean withdraw(double withdrawalAmount) { boolean withdrawalMade = false; // Insert your code here. Remember, you MUST enforce the invariant condition!!! return withdrawalMade; } // // C. double transfer(BankAccount,double) // RETurns the amount transferred: 0.0 - No transfer occurred public double transfer(double withdrawalAmount) { double amountTransfered = 0.0; // Create your method here
return amountTransfered; } // // // 3. Virtual (late binding) Methods: Extensions of class "Object" // A. toString() // Constructs Description "String" of the object String toString() { String str = ""; // Insert your code here..... return str; }
// Class Private, non-static Data Members // Private Class Data Members // 1. Variable Declarations private String name; private double balance;
} // End Class Definition: BankAccount
Project9_BankAccount.java
/****************************************************************************** * Textbook: Building Java Programs, A Back to Basics Approach, 3rd Edition * Authors: Stuart Reges and Marty Stepp * * Instructor Project Solution * * Assigned Project: 9 * Exercise: 11, 12, and 13 * * Class/File Name: Project9_BankAcount * * Revision Date Release Comment * -------- ---------- ------------------------------------------------------ * 1.0 06/12/2015 Initial Release * * * File Description * ---------------- * This file defines the the class "Project9_BankAccount", which includes all * methods, variables, and constant values. Specifically, this class contains * the Console application entry method, main(), which is a static method, * since no object of the class type is ever instantiated. * * * Program Inputs * -------------- * None * * * Program Outputs * --------------- * Device Description * --------- ----------------------------------------------------------------- * Monitor 1. Valid and invalid deposit/withdrawal amounts from a * "BankAccount" object initialized with "name" only * 2. Valid and invalid deposit/withdrawal amounts from a * "BankAccount" object initialized with "name" and start balance * only. * 3. Valid and invalid deposit/withdrawal amounts from a * "BankAccount" object initialized with "name", start balance, * and specific "transaction" fee. * 4. Various transfers of values from one "BankAccount" to another * "BankAccount" object. * * * Class Methods * ------------- * Name Description * ------------- ------------------------------------------------------------- * main OS transfers control to this method upon program execution * makeDeposit Add deposit to referenced "BankAccount" object * makeWithdrawal Withdrawal from referenced "BankAccount" object * ****************************************************************************** */ // Imported Packages
public class Project9_BankAccount { /************************************************************************** * Method: main(String[]) * * Method Description * ------------------ * This static method is the entry point to the program. * * The program tests the class "BankAccount", which allows for deposits and * withdrawals from a "BankAccount" object. The class, "BankAccount", * supports three initializing Constructors, one that takes the name of the * account only, one that takes the name and the beginning balance, and the * third that takes the name, beginning balance, and the withdrawal * transaction fee. Three objects will be defined to test all three allowed * Constructors. In addition, deposits and withdrawals will be performed to * verify the arithmetic. * * * Pre-Conditions * -------------- * None * * * ------------------------------- Arguments ------------------------------ * Type Name Description * -------- ---- -------------------------------------------------------- * String[] args Array of "String" argument(s) passed to main() when the * Console program is invoked by the User. * * * RETurn * Type Description * ------ ---------------------------------------------------------------- * void The method RETurns no value * * * Invoked Methods * --------------- * Name Description * -------------- -------------------------------------------------------- * introduction Inform User of the Project solution * makeDeposit Add deposit to referenced "BankAccount" * makeWithdrawal Withdrawal from referenced "BankAccount" * transfer Transfer amount from one account to another account * ************************************************************************** */ public static void main(String[] args) { // Constant "final" Value Declarations final double BEGINNING_BALANCE1 = 23.67; final double BEGINNING_BALANCE2 = 34.57; final double TRANSACTION_FEE = 2.50; // Create a "BankAccount" object, specifying just the account holder's // name. The beginning balance and withdrawal fee will be set to $0.0. BankAccount myAccount = new BankAccount("John Doe"); // Create arrays of deposit, withdrawal, and transfer amounts. double[] deposits = new double[] { 51.75, 14.78, -4.59 }; double[] withdrawals = new double[] { 7.96, 45.79, -3.57, 12.78, .01, 75.86 }; double[] transfers = new double[] { 41.38, -8.79, 78.67, 94.74, .01, 150.00 }; // Uninitialized Variable Declarations double deposit, withdrawal, transfer;
// Inform the User of the Project Solution introduction(); // Example #1: Account name only specified to Constructor System.out.println(" Example #1: Account object instantiated with name only"); // Display the beginning account balance System.out.println(" Beginning account:" + " " + myAccount); // Make a deposit to the account object, then "display" the object // via the overloaded "toString()" class method. deposit = deposits[0]; System.out.println(" Depositing: $" + deposit); makeDeposit(myAccount, deposit); // Now make a withdrawal withdrawal = withdrawals[0]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal); // Then another deposit deposit = deposits[1]; System.out.println(" Depositing: $" + deposit); makeDeposit(myAccount, deposit); // Then another deposit that is a negative value deposit = deposits[2]; System.out.println(" Depositing: $" + deposit); makeDeposit(myAccount, deposit); // Another withdrawal withdrawal = withdrawals[1]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal); // Another withdrawal that is a negative value withdrawal = withdrawals[2]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal); // Another withdrawal that will result in a balance of $0.00. withdrawal = withdrawals[3]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal); // Finally, a withdrawal the will exceed the current account balance withdrawal = withdrawals[4]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal);
// Example #2: Account name and beginning balance specified to the Constructor System.out.println(" Example #2: Account object instantiated with name and" + " beginning balance: $" + BEGINNING_BALANCE1); // Create a "BankAccount" object, specifying the account holder's name // and beginning balance. The withdrawal fee will be set to $0.00. // Note: This is an example of the Java feature of "garbage" collection. // The REFERENCE "account" is assigned to a new "BankAccount" class // object. Java will detect that no other reference refers to the // previous object (Name: John Doe) and will automatically return // the memory allocated for that object to the Heap Memory Manager. myAccount = new BankAccount("Sarah Jones", BEGINNING_BALANCE1); // Display the beginning account balance System.out.println(" Beginning account:" + " " + myAccount); // Make a deposit to the account object, then "display" the object // via the overloaded "toString()" class method. deposit = deposits[0]; System.out.println(" Depositing: $" + deposit); makeDeposit(myAccount, deposit); // Now make a withdrawal withdrawal = withdrawals[0]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(myAccount, withdrawal);
// Example #3: Account name, beginning balance, and transaction fee are // specified to the Constructor System.out.println(" Example #3: Account object instantiated with name," + " beginning balance: $" + BEGINNING_BALANCE2 + ", and transaction fee: $" + String.format("%.2f", TRANSACTION_FEE)); // Create a "BankAccount" object, specifying the account holder's name, // the beginning balance, and the transaction fee. BankAccount yourAccount = new BankAccount("Samuel Johnson", BEGINNING_BALANCE2, TRANSACTION_FEE); // Display the beginning account balance System.out.println(" Beginning account:" + " " + yourAccount); // Make a deposit to the account object, then "display" the object // via the overloaded "toString()" class method. deposit = deposits[0]; System.out.println(" Depositing: $" + deposit); makeDeposit(yourAccount, deposit); // Now make a withdrawal withdrawal = withdrawals[0]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(yourAccount, withdrawal); // Now make a withdrawal of the exact remaining balance // Note: The fee + withdrawal will exceed the remaining balance! withdrawal = withdrawals[5]; System.out.println(" Withdrawing: $" + withdrawal); makeWithdrawal(yourAccount, withdrawal);
// Example #4: Transfer between accounts System.out.println(" Example #4: Transfers between two accounts"); System.out.println( " myAccount: " + myAccount + " yourAccount: " + yourAccount); // Make a valid transfer from "myAccount" to "yourAccount" transfer = transfers[0]; System.out.println(" Transfering $" + transfer + " from " + myAccount.name() + "'s account to " + yourAccount.name() + "'s account"); transfer(myAccount, yourAccount, transfer); // Make an invalid transfer (negative amount) from "myAccount" to "yourAccount" transfer = transfers[1]; System.out.println(" Transfering $" + transfer + " from " + myAccount.name() + "'s account to " + yourAccount.name() + "'s account"); transfer(myAccount, yourAccount, transfer); // Make a valid transfer from "yourAccount" to "myAccount" transfer = transfers[2]; System.out.println(" Transfering $" + transfer + " from " + yourAccount.name() + "'s account to " + myAccount.name() + "'s account"); transfer(yourAccount, myAccount, transfer); // Make a second valid transfer from "yourAccount" to "myAccount" that results // in a balance of $0.01 in "myAccount" transfer = transfers[3]; System.out.println(" Transfering $" + transfer + " from " + myAccount.name() + "'s account to " + yourAccount.name() + "'s account"); transfer(myAccount, yourAccount, transfer); // Make an invalid transfer (insufficient funds) from "myAccount" to "yourAccount" transfer = transfers[4]; System.out.println(" Transfering $" + transfer + " from " + myAccount.name() + "'s account to " + yourAccount.name() + "'s account"); transfer(myAccount, yourAccount, transfer); // Make an invalid transfer (insufficient funds) from "yourAccount" to "myAccount" transfer = transfers[5]; System.out.println(" Transfering $" + transfer + " from " + yourAccount.name() + "'s account to " + myAccount.name() + "'s account"); transfer(yourAccount, myAccount, transfer); } // End static method: main(String[]) /************************************************************************** * Method: introduction() * * Method Description * ------------------ * This static method describes the Project solution to the User. * * * Pre-Conditions * -------------- * None * * * ------------------------------- Arguments ------------------------------ * *** No Arguments supplied *** * * * RETurn * Type Description * ------ ---------------------------------------------------------------- * void The method RETurns no value * * * Invoked Methods * --------------- * None * ************************************************************************** */ private static void introduction() { System.out.println(" Project #8 Solution" + " Chapter 8, Exercise #11, page 568 "); } /************************************************************************** * Method: makeDeposit(BankAccount,double) * * Method Description * ------------------ * This static method deposits the amount specified (argument) into the * specified (reference argument) "BankAccount" object. After the deposit, * the account is displayed by calling, indirectly, the overloaded * "toString()" class method. * * * Pre-Conditions * -------------- * 1. The "account" reference is not "null" * * * RETurn * Type * ------ * None * * ------------------------------- Arguments ------------------------------ * Type Name Description * ------------ -------- ------------------------------------------------ * BankAccount account Reference to a "BankAccount" object * double deposit Amount of the deposit * * * RETurn * Type Description * ------ ---------------------------------------------------------------- * void The method RETurns no value * * * Invoked Methods * --------------- * None * ************************************************************************** */ private static void makeDeposit(BankAccount account, double deposit) { // First, attempt to make the deposit if (account.deposit(deposit) ) { // A deposit amount >0 was specified, continue... // Now "display" the object to the User. By specifying the REFERENCE // to the object as an argument in the "println" method, the compiler // will generate a call to the overloaded class method, "toString()". System.out.println(" " + account); } else { // Otherwise, an invalid deposit amount was specified System.out.println(" Unable to deposit $" + String.format("%.2f", deposit)); } } /************************************************************************** * Method: makeWithdrawal(BankAccount,double) * * Method Description * ------------------ * This static method deposits the amount specified (argument) into the * specified (reference argument) "BankAccount" object. After the deposit, * the account is displayed by calling, indirectly, the overloaded * "toString()" class method. * * * Pre-Conditions * -------------- * 1. The "account" reference is not "null" * * * ------------------------------- Arguments ------------------------------ * Type Name Description * ------------ ---------- ---------------------------------------------- * BankAccount account Reference to a "BankAccount" object * double withdrawal Amount of the withdrawal * * * RETurn * Type Description * ------ ---------------------------------------------------------------- * void The method RETurns no value * * * Invoked Methods * --------------- * None * ************************************************************************** */ private static void makeWithdrawal(BankAccount account, double withdrawal) { // Attempt to make the withdrawal. The class method, "withdrawal" will // RETurn a "boolean" value; "true": Withdrawal made, // "false": Withdrawal (+ fee, if applicable) will exceed current // balance. boolean status = account.withdraw(withdrawal); if (status) { // Withdrawal was successful, display the remaining balance. System.out.println(" " + account); } else { // Otherwise, inform the User that withdrawal was NOT made. System.out.println(" Unable to withdraw $" + String.format("%.2f", withdrawal)); } // Note: A "return" statement is unnecessary for a method that "returns" // "void", but is allowed for completeness. return; } /************************************************************************** * Method: transfer(BankAccount,BankAccount,double) * * Method Description * ------------------ * This static method attempts to withdraw the secified amount from the * "source", "BankAccount" object, and if possible, deposits that amount * or part thereof, to the "destination", "BankAccount" object. * The trasfer is accomplished by invoking the "source" object's non- * static data member, "transfer", which RETurns a "bool" value, "true", * the transfer occurred. * * * Pre-Conditions * -------------- * 1. The "account" reference is not "null" * * * ------------------------------- Arguments ------------------------------ * Type Name Description * ------------ ------------ -------------------------------------------- * BankAccount source Reference to the source "BankAccount" object * BankAccount destination Reference to the destination object * double withdrawal Amount of the withdrawal * * * RETurn * Type Description * ------ ---------------------------------------------------------------- * void The method RETurns no value * * * Invoked Methods * --------------- * None * ************************************************************************** */ private static void transfer(BankAccount source, BankAccount destination, double transferAmount) { // Attempt to make the transfer. The class method, "transfer" will // RETurn a "boolean" value; "true": Transfer was made, // "false": Transfer + fee will exceed current balance of the // source account. double amountTransferred = source.transfer(destination, transferAmount); if (amountTransferred > 0.0) { // Transfer was successful, display the two accounts. System.out.printf(" Source Account: %s" + " Amount Transferred: %.2f" + " Destination Account: %s ", source, amountTransferred, destination); } else { // Otherwise, inform the User that withdrawal was NOT made. System.out.println(" Unable to transfer $" + String.format("%.2f", transferAmount)); } } } // End class definition: Project9_BankAccount
Design Considerations for Project #9
1. You must design your "BankAccount" class such that it will satisfy the requirements stated in the three text problems. The supplied Client program will invoke the required class, non-static methods described in the text and in the Project Description document (Note the additional, required method, "name()".
2. The supplied Client program creates 3 different "BankAccount" objects. You should notice that each takes different number of arguments. Therefore, you will need to supply at least 3 Constructors for the class. You are to make sure that proper values are passed to the Constructors you define, i.e. the starting balance and transaction fee must be greater than or equal to 0.0. Also note that unless supplied in a Constructor, the transaction fee is set to the specified "default" value of 0.0....hint a Class static constant (final) value.
3. For Class method "deposit" you must enforce the invariant condition that a deposit amount must be greater than 0.0. The same goes for a withdrawal and transfer amount supplied to methods "withdraw" and "transfer".
4. For a "withdraw", the transaction can be completed iff (if and only if) the specified withdraw amount PLUS the transaction fee is >= the current balance.
5. You are to keep an accumulated total of all fees charged to the account; i.e. update this amount each time the "withdraw" and "transfer" methods are called.
6. For a transfer, there is fixed, constant fee of $5.00. Note that this is a different fee than the transaction fee that applies to a withdrawal. The transfer can only occur if the current balance of the invoking "BankAccount" object exceeds $5.00. If the account balance exceeds $5.00 but is less than the requested transfer amount plus the $5.00 fee, a partial transfer can occur that leaves the invoking object's balance as exactly $0.00. Only the allowed transfer amount is "deposit"ed to the target account, the "BankAccount" object reference passed to the "transfer" method.
7. You will need to provide an overridden "toString()" method as specified in Exercise #12. In addition to the format specified in the exercise, you are to include the total accumulated fees charged, see my sample output.
8. You are free to use class private "helper" method(s) to implement your method designs. A good example would be methods(s) to enforce the invariant conditions.
Thank you!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
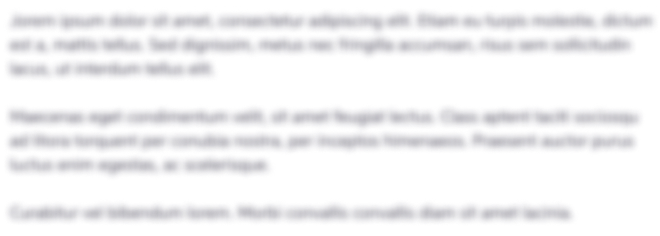
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started