Question
Program Specification: A Stack is a simple container that is initially empty and need only support three simple operations: isEmpty - reports true if the
Program Specification: A Stack is a simple container that is initially empty and need only support three simple operations:
isEmpty - reports true if the stack contains no values, otherwise reports false.
push value - adds value onto the stack.
pop - removes the most currently pushed value on the stack FIFO.
You are to extend your DynArray class to implement a stack which can hold double values and adheres to the following:
Mandatory Instance methods:
public int size() // returns the number of values which are currently on the stack
public boolean isEmpty() // returns true only if there are no values on the stack
public void push(double value) // add the specified value onto the stack
public double pop() // if the stack is not empty, // remove and returns the most currently pushd value on the stack // otherwise, // returns Double.NaN
public void stackDump() // print all of the values currenty on the stack, in the order that // they would be popd off of the stack
A Queue is a simple container that is initially empty and need only support three simple operations:
isEmpty - reports true if the queue contains no values, otherwise reports false.
que value - adds value into the queue.
deQue - removes the least currently queed value in the queue FILO.
You are to extend your DynArray class to implement a queue which can hold double values and adheres to the following:
Mandatory Instance methods:
public int size() // returns the number of values which are currently in the queue
public boolean isEmpty() // returns true only if there are no values in the queue
public void que(double value) // add the specified value into the queue
public double deQue() // if the queue is not empty, // remove and returns the least currently qued value in the queue // otherwise, / returns Double.NaN
public void queueDump() // print all of the values currenty in the queue, in the order that // they would be deQued from the queue
public class DynArray { private double[] array; private int size; private int nextIndex;
public int arraySize() { return this.size; }
public int elements() { return this.nextIndex; }
public double at(int index) { if(0 <= index && index < this.nextIndex) { return this.array[index]; } else { return Double.NaN; } }
public DynArray() { this.size = 1; this.array = new double[this.size]; this.nextIndex = 0; }
private void grow() { double[] growArray = new double[this.size * 2]; for(int i = 0; i < this.nextIndex; i++) { growArray[i] = this.array[i]; } this.array = growArray; this.size *= 2; }
private void shrink() { if(this.size/2 == this.nextIndex) { int shrink = this.size / 2; if(shrink <= 0) { shrink = 1; } double[] shrinkArray = new double[shrink]; for(int i = 0; i < this.nextIndex; i++) { shrinkArray[i] = this.array[i]; } this.array = shrinkArray; this.size = shrink; } }
public void insertAt(int index, double value) { if(0 <= index && index <= this.nextIndex) { if(this.nextIndex == this.size) { this.grow(); } for(int i = this.nextIndex; i > index; i--) { this.array[i] = this.array[i - 1]; } this.array[index] = value; this.nextIndex++; } }
public void insert(double value) { this.insertAt(this.nextIndex, value); }
public double removeAt(int index) { if(0 <= index && index < this.nextIndex) { double value = at(index); for(int i = index; i < this.nextIndex-1; i++) { this.array[i] = this.array[i + 1]; } this.shrink(); this.nextIndex--; return value; } else { return Double.NaN; } }
public double remove() { double value = at(this.nextIndex-1); this.nextIndex--; if(this.nextIndex == -1) { this.nextIndex = 0; } this.shrink(); return value; }
//accessor // prints the values of all occupied locations of the array to // the screen public void printArray() { for(int i = 0; i < this.nextIndex; i++) { System.out.println("array.at" + "(" + i + ")" + " " + this.array[i]); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
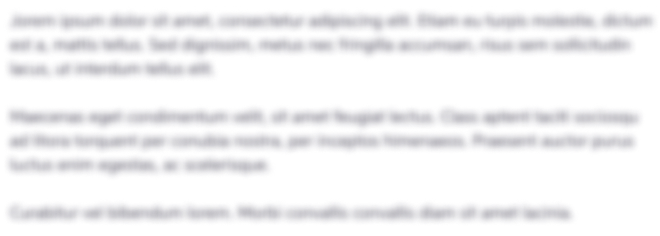
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started