Question
Program: Take another look at the Item class we created in lab 11. We will add some additional stuff to this. **ITEM CLASS** class Item_Part1
Program:
Take another look at the Item class we created in lab 11. We will add some additional stuff to this.
**ITEM CLASS**
class Item_Part1 {
/** * private variable (state of item) */ private String description; private int quantity; private double price;
/** * 3 argument constructor initializes the description, quantity and price */ public Item(String _description, int _quantity, double _price) { description = _description; quantity = _quantity; price = _price; }
/** * 2 argument constructor initializes the description and price */ public Item(String _description, double _price) { description = _description; price = _price; }
/** * accessor and modifier methods */ String getDescription() { return description; }
void setDescription(String _description) { description = _description; }
int getQuantity() { return quantity; }
void setQuantity(int _quantity) { quantity = _quantity; }
double getPrice() { return price; }
void setPrice(double _price) { price = _price; }
}// end of class Item
**ITEM CLASS PART 2**
class Item_Part2 { public static void main(String[] args) { // declare 5 element array of items Item[] itemObj = new Item[5];
//Fill the array up with the following: three Item objects using the first constructor: itemObj[0] = new Item("Spoon", 14, 1.25); itemObj[1] = new Item("Fork", 18, 1.50); itemObj[2] = new Item("Knife", 12, 2.35); //Two more objects using the second constructor: itemObj[3] = new Item("Dish", 5.95); itemObj[4] = new Item("Bowl", 4.99);
//updates itemObj[1].setPrice(1.75); itemObj[3].setQuantity(5); itemObj[4].setQuantity(8); itemObj[2].setDescription("Butter Knife");
//Using a loop and an output message, for every Item object output to the screen for (int i = 0; i < itemObj.length; i++) { System.out.println("We have " + itemObj[i].getQuantity() + " " + itemObj[i].getDescription() + "(s) at price of $" + itemObj[i].getPrice() + " each"); } } }// end of class
Start with the items created in lab 11 including three instance variables, an accessor and mutator/modifier for each, and two constructors.
Add a class constant which is an integer and represents the minimum quantity all items should have in stock( Use the value 12).
Add a Boolean method called needToOrder( ) which returns true if quantity of item is less than this minimum value.
Add toString( ) which will output this way: Item: Fork Qty: 12 Cost: $1.75
Add method called itemWorth( ) which returns current total price of the object (single object) based on the quantity on hand (qty * price)
Add method called sellSome(int) which reduces quantity of the object by the amount inputted.
Add an instance method called greaterThan( Item ) which returns a boolean based on whether the itemWorth of the calling object is worth more than the passed in object.
Add Javadoc comments to class comments before each method
Now write a better driver program to test this class.
Create an Array of 10 Items (the Inventory). Do not use a premade class such as ArrayList. We will be adding and removing Items from this Inventory. Start with an empty array. We will assume that there will always be 10 or less. When we have less than 10 we will always use only the beginning of the array, the remaining values will be null. If we delete any items in the middle of the list, you must shift them in a way to keep all the items in the beginning.
For the main program you will create a text menu that comes up on the output screen giving the user a bunch of choices. As they pick a choice, that action will be done and then the menu will repeat to the screen again. A switch statement and/or having a link to additional static methods could be useful. Your menu will do the following:
list all items
-use toString on each filled array location
list the items that need to be ordered
-process through the array until reach null
-call needToOrder on each and show the true results
-Report if no items need to be ordered
add an item to the inventory
-add it at the first null location in the array
-ask the user to give the 3 inputs and then use the constructor to create one
-Report if there is not room to add another item
Report total inventory cost
-search through entire array, and sum up the itemWorths
-report the total.
Report item that is worth the most (Use greaterThan method)
Sell some of an item
-Ask user for description, find array element and ask user to say how much is sold and update quantity.
-Report if not found should not crash
Remove an item from list
-Ask user for description, find array element
-Report if not found should not crash
-set array location to null (make sure to shift array to fix holes)
Clear list. set all array locations to null
End program
Test all of your menu choices, make sure to remove items in the middle of the list and check if the rest of the choices still work. I will test very extensively, so be prepared to do so also.
Each of these programs must be done in a separate file. Name the class Item.java, and the driver program Inventory.java. Name the class in each file these names as well. If you do not name these files correctly you will lose points.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
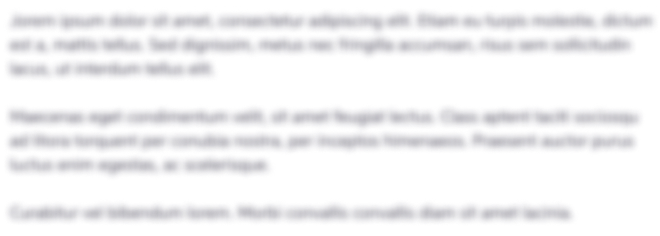
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started