Question
// Program to determine tomorrow's date #include #include struct date { int month; int day; int year; }; // Function to calculate tomorrow's date struct
// Program to determine tomorrow's date
#include
#include
struct date
{ int month; int day; int year; };
// Function to calculate tomorrow's date
struct date dateUpdate (struct date today)
{struct date tomorrow;
int numberOfDays (struct date d);
if ( today.day != numberOfDays (today) ) {
tomorrow.day = today.day + 1;
tomorrow.month = today.month;
tomorrow.year = today.year;
} else if ( today.month == 12 ) { // end of year
tomorrow.day = 1;
tomorrow.month = 1;
tomorrow.year = today.year + 1;
}
else { // end of month
tomorrow.day = 1;
tomorrow.month = today.month + 1;
tomorrow.year = today.year;
}
return tomorrow;
}
// Function to find the number of days in a month
int numberOfDays (struct date d)
{
int days;
bool isLeapYear (struct date d);
const int daysPerMonth[12] =
{ 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
if ( isLeapYear (d) && d.month == 2 )
days = 29;
else
days = daysPerMonth[d.month - 1];
return days;
}
// Function to determine if it's a leap year
bool isLeapYear (struct date d)
{
bool leapYearFlag;
if ( (d.year % 4 == 0 && d.year % 100 != 0) ||
d.year % 400 == 0 )
leapYearFlag = true; // It's a leap year
else
leapYearFlag = false; // Not a leap year
return leapYearFlag;
}
int main (void)
{
struct date dateUpdate (struct date today);
struct date thisDay, nextDay;
printf ("Enter today's date (mm dd yyyy): ");
scanf ("%i%i%i", &thisDay.month, &thisDay.day, &thisDay.year);
nextDay = dateUpdate (thisDay);
printf ("Tomorrow's date is %i/%i/%.2i. ",nextDay.month,
nextDay.day, nextDay.year % 100);
return 0;
}
Looking for someone to replace the dateUpdate() function with compound literals. Also looking for the int main (void) to be moved from the bottom of the code to the top. All I need changed is the dateUpdate() function and the int main (void) to be moved under the #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
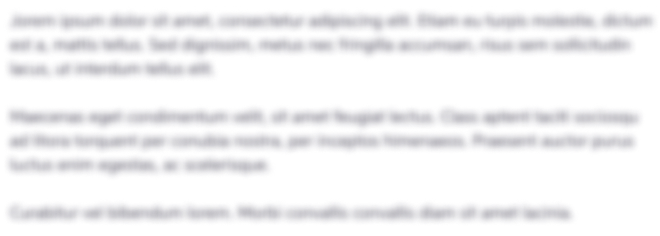
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started