Question
Program : Write a C program to read up to 20 lines from an input file students.txt into an array of struct. Use Notepad to
Program:
Write a C program to read up to 20 lines from an input file students.txt into an array of struct. Use Notepad to create a text file named students.txt with less than 20 lines. Save the file in the same location as the .c file. Each line in the file should be arranged the same way: first name, last name and a GPA as shown below:
Bobby Good 3.00
Sara Sands 3.50
Ali Bull 3.20
Abe Banks 3.40
Stacy Lee 2.70
Laila Guru 3.90
Fara Smith 2.45
Global Declarations:
Declare a struct with members to store a first name, a last name and a GPA. Assume that the first name and last name wont exceed 10 characters. Fill in the blanks:
typedef struct
{
------------------
------------------
------------------
} student_record;
Named Constant: Declare a named constant for the size of the array of struct = 20
Required function:
1)display_array()
a.Input Parameters: Array of struct and number of students stored in the array.
b.Output: void.
Task: display the array content in a tabular format along with column headers.
compute_avg()
a.Input Parameters: Array of struct and number of students stored in the array.
b.Output: float.
Task: compute the average GPA and return it as output.
int main()
{
Declare an array of struct to store up to 20 students (use the named constant)
Declare a FILE pointer: FILE * ptr;
Open the file students.txt to read from.
If the open operation failed:
Display : File open operation for reading failed.
return 1; to end the program
Read all the lines from the file into the array of struct.TIP (optional)
Declare a struct variable:
Read data from the file into this variable.
Assign this struct variable to the array at proper index.
Close the file.
Display the number of lines read from the file.
Invoke display_array to display the array content.
Invoke the function compute_avg to compute the average gpa.
Display the average GPA.
Add yourself (your first name, last name and gpa) to the array as the last student.
Invoke display_array to display the array.
Search for the unique last name Guru in this array.
If found display Gurus first name and GPA.
Display a message if search failed.
// save data to the same text file:
Open the file students.txt to save to. Use the same file pointer
Write a loop to write all the students to the text file.
Close the file.
_getch( );
return 0;
}
NOTES: These notes make sense once you have completed the program.
Each time you run the program, your data will be added to the end of the file, so you will see repeated lines of yourself. You can easily open the text file and delete yourself from the file.
Make sure there is no extra line at the end of the text file. The cursor must be at the end of the last line in the file, not at the beginning of the next line.
Output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
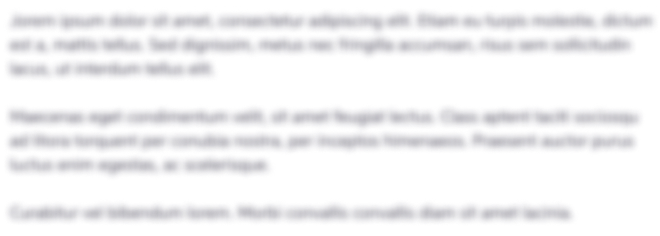
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started