Question
Programming Exercise 11 from C++ Programming Chapter 11 Design various classes and write a program to comperterize the billing system of a hospital. Design the
Programming Exercise 11 from C++ Programming Chapter 11 Design various classes and write a program to comperterize the billing system of a hospital. Design the class doctorType, inherited from the class personType, with an additional data member to store a doctor's speciality. Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
Design the class billType with data members to store a patient's ID and a patient's hospital charges, such as pharmacy charges for medicine, doctor's fee, and room charges. Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
Design the class patientType, inherited from the class personType, with additional data members to store the patient's ID, age, date of birth, attending physician's name, the date when the patient was admitted in the hospital, and the date when the patient was discharged from the hospital. (Use class dateType to store the date of birth, admit date, discharge date, and the class doctorType to store the attending physician's name.) Add appropriate constructors and member functions to initialize, access, and manipulate the data members. Write a program to test your classes. (class personType and class dateType follow)
class personType {
public:
void print() const;
void setName(string first, string last);
string getFirstName() const;
string getLastName() const;
personType(string first = "", string last = "");
private:
string firstName;
string lastName;
};
void personType::print() const {
cout << firstName << " " << lastName; }
void personType::setName(string first, string last) {
firstName = first;
lastName = last; }
string personType::getFirstName() const {
return firstName; }
string personType::getLastName() const {
return lastName; }
personType::personType(string first, string last) {
firstName = first;
lastName = last; }
class dateType {
public:
void setDate(int month, int day, int year);
int getDay() const;
int getMonth() const;
int getYear() const;
void printDate() const;
dateType(int month = 1, int day = 1, int year = 1900);
private:
int dMonth;
int dDay;
int dYear;
};
void dateType::setDate(int month, int day, int year) {
dMonth = month;
dDay = day;
dYear = year; }
int dateType::getDay() const {
return dDay; }
int dateType::getMonth() const {
return dMonth; }
int dateType::getYear() const {
return dYear; }
void dateType::printDate() const {
cout << dMonth << "-" << dDay << "-" << dYear; }
dateType::dateType(int month, int day, int year) {
dMonth = month;
dDay = day;
dYear = year; } Example Code with errors to fix follows:
#include
using namespace std;
class PersonType { public: void print() const; void SetName(string first, string last); string getFirstName() const; string getLastName() const; PersonType(string first = "", string last = "");
private: string FirstName, LastName; }; void PersonType :: print() const { cout << FirstName << " " << LastName << endl; } void PersonType :: SetName(string first, string last) { FirstName = first; LastName = last; cout << "Please Enter Your FIRST Name. "; cin >> first; cout << "Please Enter Your LAST Name. "; cin >> last; } string PersonType :: getFirstName() const { return FirstName; } string PersonType :: getLastName() const { return LastName; } PersonType :: PersonType(string first, string last) { FirstName = first; LastName = last; }
class DoctorType : public PersonType { public: void SetName(string first, string last); void SetSpeciality(string Special); string getSpeciality() const; string getFirstName() const; string getLastName() const; DoctorType(string Special = "");
private: string FirstName, LastName, Speciality; }; void DoctorType :: SetSpeciality(string Special) { Speciality = Special; cout << "Please Enter Your SPECIALITY. "; cin >> Special; } string DoctorType :: getSpeciality() const { return Speciality; } DoctorType :: DoctorType(string Special = "") { Speciality = Special; }
class DateType { public: void printDate() const; void SetDate(int Day, int Month, int Year); int getTheDay() const; int getTheMonth() const; int getTheYear() const; DateType(int Day,int Month, int Year); private: int TheDay, TheYear, TheMonth; }; void DateType :: printDate() const { cout << TheDay << " " << TheMonth << " " << TheYear << endl; } void DateType :: SetDate(int Day, int Month, int Year) { TheDay = Day; TheMonth = Month; TheYear = Year; cout << "Please Enter The Day (DD). "; cin >> Day; cout << "Please Enter The Month (MM). "; cin >> Month; cout << "Please Enter The Year (YYYY). "; cin >> Year; } int DateType :: getTheDay() const { return TheDay; } int DateType :: getTheMonth() const { return TheMonth; } int DateType :: getTheYear() const { return TheYear; } DateType::DateType(int Day, int Month, int Year) { TheDay = Day; TheMonth = Month; TheYear = Year; }
class DateOfBirthType : public DateType { public: void printDOB() const; void SetDOB(int Day, int Month, int Year); int getTheDay() const; int getTheMonth() const; int getTheYear() const;
private: int TheDay, TheYear, TheMonth; }; void DateOfBirthType :: printDOB() const { cout << "Patients Date Of Birth: " << TheDay << "/" << TheMonth << "/" << TheYear << endl; } void DateOfBirthType :: SetDOB(int Day, int Month, int Year) { TheDay = Day; TheMonth = Month; TheYear = Year;
cout << "Enter The Patients DAY Of Birth. "; cin >> Day; cout << "Enter The Patients MONTH Of Birth. "; cin >> Month; cout << "Enter The Patients YEAR Of Birth. "; cin >> Year; }
class AdmittanceDateType : public DateType { public: void printAdmittanceDate() const; void SetAdmittanceDate(int Day, int Month, int Year); int getTheDay() const; int getTheMonth() const; int getTheYear() const;
private: int TheDay, TheYear, TheMonth; }; void AdmittanceDateType :: printAdmittanceDate() const { cout << "The Patients Admittance Date: " << TheDay << " " << TheMonth << " " << TheYear << endl; } void AdmittanceDateType :: SetAdmittanceDate(int Day, int Month, int Year) { TheDay = Day; TheMonth = Month; TheYear = Year;
cout << "Enter The Patients DAY Of Admittance. "; cin >> Day; cout << "Enter The Patients MONTH Of Admittance. "; cin >> Month; cout << "Enter The Patients YEAR Of Admittance. "; cin >> Year; }
class DischargeDateType : public DateType { public: void printDischargeDate() const; void SetDischargeDate(int Day, int Month, int Year); int getTheDay() const; int getTheMonth() const; int getTheYear() const;
private: int TheDay, TheYear, TheMonth; }; void DischargeDateType :: printDischargeDate() const { cout << "The Patients Discharge Date: " << TheDay << " " << TheMonth << " " << TheYear << endl; } void DischargeDateType :: SetDischargeDate(int Day, int Month, int Year) { TheDay = Day; TheMonth = Month; TheYear = Year;
cout << "Enter The Patients DAY Of Discharge. "; cin >> Day; cout << "Enter The Patients MONTH Of Discharge. "; cin >> Month; cout << "Enter The Patients YEAR Of Discharge. "; cin >> Year; }
class PatientType : public PersonType { void print() const; void SetName(string first, string last); void SetPatientID(int ID); void SetPatientAge(int Age); int getPatientAge() const; int getPatientID() const; string getFirstName() const; string getLastName() const; PatientType(string first = "", string last = ""); PatientType(int ID = 0); PatientType(int Age = 0);
private: string FirstName, LastName; int PatientID, PatientAge; }; int PatientType::getPatientID() const { return PatientID; } int PatientType::getPatientAge() const { return PatientAge; } PatientType::PatientType(string first = "", string last = "") { FirstName = first; LastName = last; } PatientType::PatientType(int ID = 0) { PatientID = ID; } PatientType::PatientType(int Age = 0) { PatientID = Age; } int main() { int choice;
PersonType *Person = NULL;
cout << "Who would you like to input information for? "; cout << " 1 - Doctor "; cout << " 2 - Patient "; cin >> choice;
if (choice == 1) { Person = new DoctorType(); Person->SetName(); Person->SetSpeciality(); } else if (choice == 2) { Person = new PatientType(); Person->; Person->; } return 0; } Errors are:
line 68: 'DoctorType::DoctorType': redefinition of default argument: parameter 1
line 224: 'PatientType::PatientType': redefinition of default argument: parameter 1
line 224: 'PatientType::PatientType(int)': member function already defined or declared
line 238: 'PatientType::PatientType': redefinition of default argument: parameter 1
line 238: 'PatientType::PatientType': redefinition of default argument: parameter 2
line 243: 'PatientType::PatientType': redefinition of default argument: parameter 1
line 247: 'PatientType::PatientType': redefinition of default argument: parameter 1
line 248: function 'PatientType::PatientType(int)' already has a body
line 265: too few arguments in function call
line 265: 'PersonType::SetName': function does not take 0 arguments
line 266: class 'PersonType" has no member "SetSpecialty"
line 266: 'SetSpecialty': is not a member of 'PersonType'
line 270: more than one instance of "PatientType::PatientType" matches the argument list
line 270: 'PatientType::PatientType': ambiguous call to overloaded function
line 271: expected a member name
line 271: syntax error: ';'
line 272: expected a member name
line 272: 'Person': is not a member of 'PersonType'
line 272: syntax error: ';'
Step by Step Solution
There are 3 Steps involved in it
Step: 1
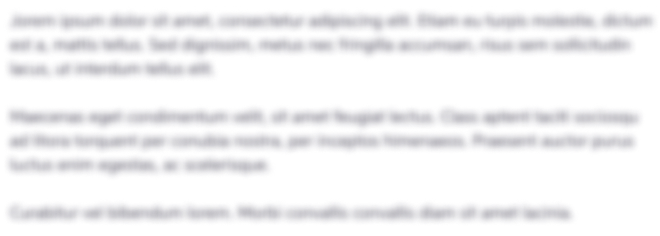
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started