Question
Programming Exercise 17-1 In C++ there are Two stacks of the same type are the same if they have the same number of elements and
Programming Exercise 17-1
In C++ there are Two stacks of the same type are the same if they have the same number of elements and their elements at the corresponding positions are the same. Overload the relational operator == for the class stackType that returns true if two stacks of the same type are the same; it returns false otherwise. Also, write the definition of the function template to overload this operator. Write a program to test the various overloaded operators and functions of class stackType.
main.cpp:
#include
#include
using namespace std;
//definition of the template class stackType
template
class stackType
{
// data memebers of the class
private :
int maxStackSize;
int stackTop;
Type *list;
// data methods of the class
public :
void initializeStack();
bool isFullStack() const;
bool isEmptyStack() const;
void push( const Type& );
void pop();
Type top() const;
stackType( int = 20 );
~stackType();
bool operator==( const stackType
}; // end template class stackType
// initialize the stack
template
void stackType
{
stackTop = 0;
} // end function initializeStack
// check for stack fullness
template
bool stackType
{
return ( stackTop == maxStackSize );
} // end function isFullStack
// check for stack empty
template
bool stackType
{
return ( stackTop == 0 );
} // end function isEmptyStack
// insert an element into stack
template
void stackType
{
if ( !isFullStack() )
{
list[ stackTop ] = newItem;
stackTop++;
} // end if
else
cout
} // end function push
// delete an element from the stack
template
void stackType
{
if ( !isEmptyStack() )
stackTop--;
else
cout
} // end function pop
// return the value of stack-top
template
Type stackType
{
assert( stackTop != 0 );
return list[ stackTop - 1 ];
} // end function top
// constructor for the class stackType
template
stackType
{
if ( stackSize
{
cout
stackSize = 10;
} // end if
else
maxStackSize = stackSize;
stackTop = 0;
list = new Type[ maxStackSize ];
} // end constructor stackType
// destructor for the class stackType
template
stackType
{
delete[] list;
} // end destructor stackType
// overload the equality operator
template
bool stackType
( const stackType
{
// check for same number of elements
if ( this->stackTop != right.stackTop )
return false;
//check for equality of elements at corresponding positions
for ( int i = 0; i
if ( this->list[ i ] != right.list[ i ] )
return false;
return true;
}
//main function
int main()
{
// let the user know about the program
cout
// create objects of type stackType
stackType
stackType
// insert elements into the stacks
cout
for ( int i = 5; i
{
s1.push( i );
s2.push( i );
} // end for
//check and print whether the stacks are equal or not
if ( s1 == s2 )
cout
else
cout
// insert one more element into the second stack
cout
s2.push( 11 );
//check and print whether the stacks are equal or not
if ( s1 == s2 )
cout
else
cout
cout
system("pause");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
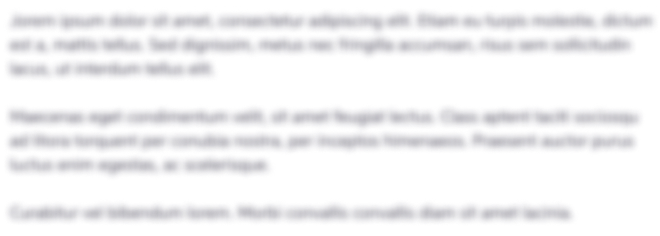
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started