Question
Programming Language: JAVA Driver.java /** * * Number System Converters * */ import java.io.*; /** * Driver Class */ public class Driver { public static
Programming Language: JAVA
Driver.java
/** * * Number System Converters * */
import java.io.*;
/** * Driver Class */ public class Driver { public static void main(String[] args) throws IOException { int choice;
PrintWriter pw = new PrintWriter(new FileWriter("csis.txt")); Decimal dec = new Decimal(pw); Binary bin = new Binary(pw); Hexadecimal hex = new Hexadecimal(pw); Menu menu = new Menu(pw);
do { menu.display(); choice = menu.getSelection(); switch (choice) { case 1 : dec.decToBin(); break; case 2 : dec.decToHex(); break; case 3 : bin.binToDec(); break; case 4 : bin.binToHex(); break; case 5 : hex.hexToDec(); break; case 6 : hex.hexToBin(); break; } } while (choice != 7); pw.close(); } }
Binary.java
import java.io.*; import java.util.Scanner;
/** * Binary Class * */ public class Binary { Scanner scan = new Scanner(System.in); private PrintWriter pw; private String binary; private String convert; /** * Constructor for Binary Class * @param PrintWriter variable */ public Binary(PrintWriter pw) { this.pw = pw; } /** * Run methods to convert binary number to decimal and * output the results * @param none * @return none */ public void binToDec() { getBin(); toDec(); outDec(); } /** * Run methods to convert binary number to hexadecimal and * output the results * @param none * @return none */ public void binToHex() { getBin(); toHex(); outHex(); } /** * Get binary number from user input * @param none * @return none */ private void getBin() { System.out.print("Please enter binary number to convert: "); binary = scan.next(); this.pw.println("Please enter binary number to convert: " + binary); } /**
I have a project, but I have to redo the problem.
* Convert binary value to decimal * @param none * @return none */ private void toDec() { int number = 0; convert = ""; for (int i=0; i 0; i--) { value = Character.getNumericValue(binary.charAt(i-1)); number += (value * Math.pow(2,mult)); mult++; } do { value = number % 16; number /= 16; if(value == 10) convert = "A" + convert; else if (value == 11) convert = "B" + convert; else if (value == 12) convert = "C" + convert; else if (value == 13) convert = "D" + convert; else if (value == 14) convert = "E" + convert; else if (value == 15) convert = "F" + convert; else convert = value + convert; } while (number != 0); } /** * Output results for binary to decimal conversion * @param none * @return none */ private void outDec() { System.out.println(binary + " to Decimal: " + convert); System.out.println(); this.pw.println(binary + " to Decimal: " + convert); this.pw.println(); } /** * Output results for binary to hexadecimal conversion * @param none * @return none */ private void outHex() { System.out.println(binary + " to Hexadecimal: " + convert); System.out.println(); this.pw.println(binary + " to Hexadecimal: " + convert); this.pw.println(); } }
Decimal.java
import java.io.*; import java.util.Scanner;
/** * Decimal Class * */ public class Decimal { Scanner scan = new Scanner(System.in); private PrintWriter pw; private int decimal; private String convert; /** * Constructor for Decimal Class * @param PrintWriter variable */ public Decimal(PrintWriter pw) { this.pw = pw; } /** * Run the methods to convert decimal number to binary and * output the results * @param none * @return none */ public void decToBin() { getDec(); toBin(); outBin(); } /** * Run the methods to convert decimal number to hexadecimal and * output the results * @param none * @return none */ public void decToHex() { getDec(); toHex(); outHex(); } /** * Get decimal value from user input * @param none * @return none */ private void getDec() { System.out.print("Please enter decimal number to convert: "); decimal = scan.nextInt(); this.pw.println("Please enter decimal number to convert: " + decimal); } /** * Convert decimal value to binary * @param none * @return none */ private void toBin() { int number = decimal; convert = ""; do { if (number % 2 == 0) convert = "0" + convert; else convert = "1" + convert; number /= 2; } while (number > 0); } /** * Convert the decimal value to hexadecimal * @param none * @return none */ private void toHex() { convert = ""; String hexTable = "0123456789ABCDEF"; int number = decimal; int index; if (number == 0) convert = "0"; while (number > 0) { index = number % 16; convert = hexTable.charAt(index) + convert; number /= 16; } } /** * Output decimal to binary conversion * @param none * @return none */ private void outBin() { System.out.println(decimal + " to Binary: " + convert); System.out.println(); this.pw.println(decimal + " to Binary: " + convert); this.pw.println(); } /** * Output decimal to hexadecimal conversion * @param none * @return none */ private void outHex() { System.out.println(decimal + " to Hexadecimal: " + convert); System.out.println(); this.pw.println(decimal + " to Hexadecimal: " + convert); this.pw.println(); } }
Hexadecimal.java
import java.io.*; import java.util.Scanner;
/** * Hexadecimal Class * */ public class Hexadecimal { Scanner scan = new Scanner(System.in); private PrintWriter pw; private String hex; private String convert; /** * Constructor for Hexadecimal Class * @param PrintWriter variable */ public Hexadecimal(PrintWriter pw) { this.pw = pw; } /** * Run methods to convert hexadecimal number to decimal and * output the results * @param none * @return none */ public void hexToDec() { getHex(); toDec(); outDec(); } /** * Run methods to convert hexadecimal number to binary and * output the results * @param none * @return none */ public void hexToBin() { getHex(); toBin(); outBin(); } /** * Input is run through a table of hex values and * returns its decimal base 10 value * @param hex digit to be examined * @return returns decimal base 10 value of hex digit */ private int hexTable(char ch) { switch (ch) { case '0': return 0; case '1': return 1; case '2': return 2; case '3': return 3; case '4': return 4; case '5': return 5; case '6': return 6; case '7': return 7; case '8': return 8; case '9': return 9; case 'a': case 'A': return 10; case 'b': case 'B': return 11; case 'c': case 'C': return 12; case 'd': case 'D': return 13; case 'e': case 'E': return 14; case 'f': case 'F': return 15; default: return 0; } } /** * Get hexadecimal value from user input * @param none * @return none */ private void getHex() { System.out.print("Please enter hexadecimal number to convert: "); hex = scan.next(); this.pw.println("Please enter hexadecimal number to convert: " + hex); } /** * Converts hexadecimal value to decimal * @param none * @return none */ private void toDec() { convert = ""; int number = 0; int index = 0; for(int i=0; i 0; i--) { value = hexTable(hex.charAt(i-1)); number += (value * Math.pow(16, mult)); mult++; } do { remainder = number % 2; number /= 2; convert = remainder + convert; } while (number != 0); } /** * Output hexadecimal to decimal conversion * @param none * @return none */ private void outDec() { System.out.println(hex + " to Decimal: " + convert); System.out.println(); this.pw.println(hex + " to Decimal: " + convert); this.pw.println(); } /** * Output hexadecimal to binary conversion * @param none * @return none */ private void outBin() { System.out.println(hex + " to Binary: " + convert); System.out.println(); this.pw.println(hex + " to Binary: " + convert); this.pw.println(); } }
Menu.java
import java.io.*; import java.util.Scanner;
/** * Menu Class * */ public class Menu { Scanner scan = new Scanner(System.in); private PrintWriter pw; private int choice; /** * Constructor for Menu Class * @param PrintWriter variable */ public Menu(PrintWriter pw) { this.pw = pw; } /** * Displays menu for the Number Systems program * @param none * @return none */ public void display() { System.out.println("Number Systems Lab Menu"); System.out.println("Convert decimal to binary - 1"); System.out.println("Convert decimal to hexadecimal - 2"); System.out.println("Convert binary to decimal - 3"); System.out.println("Convert binary to hexadecimal - 4"); System.out.println("Convert hexadecimal to decimal - 5"); System.out.println("Convert hexadecimal to binary - 6"); System.out.println("Exit Program - 7"); System.out.print("Please enter choice: "); choice = scan.nextInt(); this.pw.println("Number Systems Lab Menu"); this.pw.println("Convert decimal to binary - 1"); this.pw.println("Convert decimal to hexadecimal - 2"); this.pw.println("Convert binary to decimal - 3"); this.pw.println("Convert binary to hexadecimal - 4"); this.pw.println("Convert hexadecimal to decimal - 5"); this.pw.println("Convert hexadecimal to binary - 6"); this.pw.println("Exit Program - 7"); this.pw.println("Please enter choice: " + choice); } /** * Returns the user's menu choice * @param none * @return the user's choice */ public int getSelection() { return choice; } }
This is my code, but I have to change Binary class
This is the instruction of Binary to Hexadecimal
I know I will get same out even I don't need to follow the instruction, but the teacher wants me to follow her instruction.
This instruction use hex.setCharAt and string bin and substring and string builder.
Please, also do extra credit. It's like if user input number not 1 or 0 on binary or give a too large number, it wants the program to send out the error message instead crash.
Also, It should ask the user again to put right number and the right value.
1. Please fix my binary method to similar like instruction 1
2. Please do extra credit.
3. It's ok you guys change my codes or fix my codes.
Programming Language: JAVA
*Number Systems Lab Notes 5: Binary to Hexadecimal Conversion The binary to hex conversion is more of an algorithm that maps one value to another rather than a mathematical conversion algorithm used by the other base conversions First, you must read the 32-bit binary number (don't input spaces) into a String object, bin. Then you must create a StringBuilder object, hex, perhaps initialized to "00000000", which will hold the resulting hex conversion value The reason you need to use a StringBuilder object to hold the resulting hex value rather than a String object is because the value of a String object is immutable and cannot be changed once created. The value of a StringBuilder object can be modified after creation. Otherwise String and StringBuilder objects are prety much the same. Next you must iterate through bin, the String object, looking at 4-bits at a time. The substring function will allow you to look at 4-bits and will return the resulting 4-bits as a String: bin.substring (i, + 4) The String that the substring function returns can then be compared against a string constant holding a 4-bit value: if (bin.substring(i, i + 4).equals ("0000")) If the comparison returns true, then you can set the appropriate position in the String object, hex, to the appropriate hex digit: if (bin . substring (i, i 4).equals ("O000")) + hex.setCharAt (j, '0) else if (bin.substring (i, i 4) .equals("0001") hex.setCharAt (j, '1' All of this will take place within a for loop that initializes i and j to 0 and increments i by 4 and j by 1Step by Step Solution
There are 3 Steps involved in it
Step: 1
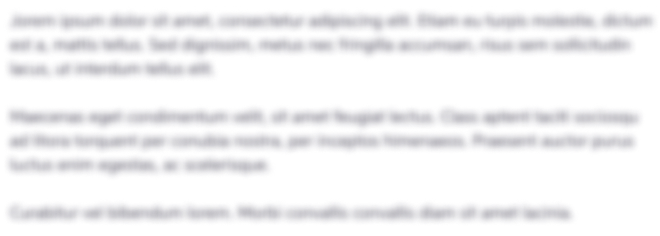
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started