Question
Programming Task Text Adventure Game, 80 points total To motivate this project, assume that you are a just-hired developer at a gaming software company. You
Programming Task Text Adventure Game, 80 points total
To motivate this project, assume that you are a just-hired developer at a gaming software company. You have been assigned the task of writing a simple text adventure game in Java. Luckily, the company has in the past released a prior version of the game. You are given several java files: two java superclasses, a java file that is a sample program that uses the two superclasses, a Utilties.java file, and a CustomPlayer.java file (that you'll modify in the last step of this programming project). Your task is to write three new versions of a game, by extending the superclasses, as was shown in lecture.
Getting Started
Download and save the following four files, which are available on the Project1 tab of the course website: Player.java, Room.java, Utilities.java, and CustomPlayer.java. Also download and save the file gameDemoSimple.java, I pasted them at the end.
The Player class is a superclass that holds information about the game's player. Do NOT modify the Player class in any way. Do NOT add code. Do NOT remove code. Leave it as-is. A player has an inventory (that is a String, with items separated by semicolons), a name, and the field roomNum, which is the ID of the room where the player currently is located within the game grid. The methods in the Player class are used to add or remove items from the player's inventory, and to retrieve the values of the private instance fields. The constructor for the Player class takes as an argument the player's name, a room number (that designates the room where the player begins the game), and a String that represents the player's inventory. Objects in the inventory are separated by semi-colons. For example, to create a new player with the name Santa, which begins the game in room 1, and which has an inventory of toys, hot chocolate, and mittens, you would write:
Player player = new Player(Santa, 1, toys;hot chocolate;mittens);
Please help.
I can't seem to get the monster room to override room and output correctly.
What I have for code:
*****************************************************************************************
// sample game demo file
public class GameDemoSimple {
public static void main(String args[]) {
// designate how many rooms are in the game final int NUM_ROOMS = 3;
// declare array of rooms Room[] gameBoard = new Room[NUM_ROOMS];
// populate the room array, using the superclass Room gameBoard[0] = new Room("First Room", "1 apple;pumpkin", 0, "e1;w2"); gameBoard[1] = new Room("Blue Room", "3 grapes;barking Germany Shephard", 1, "w0;e2"); gameBoard[2] = new Room("Green Room", "meowing kitten;", 2, "w1;e0");
// keep track of which room player is in int playerRoomNumber = 0;
// create player object, using the superclass Player Player player = new Player("Tatiana", playerRoomNumber, "bubble gum;band-aid;toothpick");
// play the game, as long as player object has field continuePlay set to true while (player.getContinuePlay() == true) { playerRoomNumber = gameBoard[playerRoomNumber].playRoom(player); } } **************************************************************************************************************
public class CustomPlayer extends Player {
public CustomPlayer(String name, int room, String inventory) { super(name, room, inventory);
} }
*******************************************************************************************************
// import statements
import java.util.StringTokenizer;
// class for a generic player public class Player {
// fields private int roomNum; private String name; private String playerInventory; private boolean continuePlay;
// constructor public Player(String name, int room, String inventory) { this.name = name; roomNum = room; continuePlay = true; playerInventory = inventory; }
// getter method to return player's inventory public String getInventory() { return playerInventory; }
// getter function to get listing of inventor public void printInventory() { StringTokenizer strT = new StringTokenizer(playerInventory, ";"); System.out.println(name + "'s items: "); int numItems = 0; while (strT.hasMoreTokens()) { System.out.println(" " + strT.nextToken()); numItems++; } System.out.println(" A total of " + numItems + " item(s)"); }
// setter function public void setContinuePlay(boolean continuePlay) { this.continuePlay = continuePlay; }
// getter function public boolean getContinuePlay() { return continuePlay; }
// getter function public String getName() { return name; }
// add an item to user's inventory public void addToInventory(String item) { playerInventory = Utilities.addToString(item, playerInventory); }
// remove item from user's inventory public void removeFromInventory(String item) { playerInventory = Utilities.removeFromList(item, playerInventory); } }
**************************************************************************************************************
// import statements
import java.util.StringTokenizer; import java.util.Scanner;
// The superclass with fields and methods // for a generic game room public class Room {
// fields private String roomObjects; private String roomName; private String roomExits; private int roomNum;
// The playRoom method can be invoked with either // a CustomPlayer object OR a Player object. They // both invoke the same method, getNextRoom public int playRoom(CustomPlayer player) { return getNextRoom(player); }
public int playRoom(Player player) { return getNextRoom(player); }
private int getNextRoom(Player player) {
// inform the player of his/her current room System.out.println("You are in the " + roomName); System.out.println("What would you like to do?");
// variables for player interaction String command; int newRoomNum = roomNum; Scanner keyboard = new Scanner(System.in); command = keyboard.nextLine();
// switch on the user's command switch (command) { case "exits": printListOfExits(); break; case "look": printObjectsInRoom(); break; case "inventory": player.printInventory(); break; case "go west": case "go east": case "go south": case "go north": newRoomNum = leaveRoom(command); break; case "quit": player.setContinuePlay(false); break; case "pick up": userPicksUp(player); break; case "drop": userDrops(player); break; case "help": Utilities.printHelp(); break; default: System.out.println("Invalid command. Type help for details."); } return newRoomNum; }
// transfer item from room to user's inventory protected void userPicksUp(Player player) { System.out.println("What would you like to pick up?"); Scanner keyboard = new Scanner(System.in); String itemToPickUp = keyboard.nextLine(); if (Utilities.isItemInContainer(itemToPickUp, roomObjects)) { player.addToInventory(itemToPickUp); roomObjects = Utilities.removeFromList(itemToPickUp, roomObjects); } else { System.out.println("That item is not in the room"); } }
// transfer item from user's inventory to room protected void userDrops(Player player) { System.out.println("What would you like to drop?"); Scanner keyboard = new Scanner(System.in); String itemToDrop = keyboard.nextLine(); if (Utilities.isItemInContainer(itemToDrop, player.getInventory())) { player.removeFromInventory(itemToDrop); roomObjects = Utilities.addToString(itemToDrop, roomObjects); } else { System.out.println("You cannot drop something that you don't have"); } }
// constructor public Room(String roomName, String objects, int roomNum, String listOfExits) { this.roomName = roomName; this.roomObjects = objects; this.roomNum = roomNum; this.roomExits = listOfExits; }
// getter function public String getRoomName() { return roomName; }
// getter function public String getRoomObjects() { return roomObjects; }
// getter function public int getRoomNum() { return roomNum; }
// add an item to room's inventory public void addToObjects(String item) { roomObjects = Utilities.addToString(item, roomObjects); }
// remove item from rooms's inventory public void removeFromObjects(String item) { roomObjects = Utilities.removeFromList(item, roomObjects); }
// determine new room number if user proceeds through an exit public int leaveRoom(String command) {
StringTokenizer exitsT = new StringTokenizer(roomExits, ";"); int exitRoom = -1;
if (command.equals("go west")) { while (exitsT.hasMoreTokens()) { String nextToken = exitsT.nextToken(); if (nextToken.substring(0, 1).equals("w")) { exitRoom = Integer.parseInt(nextToken.substring(1)); } } } else if (command.equals("go east")) { while (exitsT.hasMoreTokens()) { String nextToken = exitsT.nextToken(); if (nextToken.substring(0, 1).equals("e")) { exitRoom = Integer.parseInt(nextToken.substring(1)); } } } else if (command.equals("go north")) { while (exitsT.hasMoreTokens()) { String nextToken = exitsT.nextToken(); if (nextToken.substring(0, 1).equals("n")) { exitRoom = Integer.parseInt(nextToken.substring(1)); } } } else if (command.equals("go south")) { while (exitsT.hasMoreTokens()) { String nextToken = exitsT.nextToken(); if (nextToken.substring(0, 1).equals("s")) { exitRoom = Integer.parseInt(nextToken.substring(1)); } }
} if (exitRoom == -1) { System.out.println("That exit is not available"); return roomNum; } else { return exitRoom; } }
// getter function to list items in room public void printObjectsInRoom() {
StringTokenizer strT = new StringTokenizer(roomObjects, ";"); System.out.println("Items in " + roomName); int numItems = 0; while (strT.hasMoreTokens()) { System.out.println(" " + strT.nextToken()); numItems++; } System.out.println(" A total of " + numItems + " item(s)"); }
// getter function to get list of exits public void printListOfExits() {
StringTokenizer exitsT = new StringTokenizer(roomExits, ";"); while (exitsT.hasMoreTokens()) { String nextToken = exitsT.nextToken(); if (nextToken.substring(0, 1).equals("n")) { System.out.println("There is an exit to the north"); } else if (nextToken.substring(0, 1).equals("e")) { System.out.println("There is an exit to the east"); } else if (nextToken.substring(0, 1).equals("s")) { System.out.println("There is an exit to the south"); } else if (nextToken.substring(0, 1).equals("w")) { System.out.println("There is an exit to the west"); } } } }
*****************************************************************************************************
import java.util.StringTokenizer;
public class Utilities {
public static String addToString(String item, String list) { StringBuilder listSB = new StringBuilder(list); listSB.append(";" + item); return listSB.toString(); }
public static boolean isItemInContainer(String item, String container) { StringTokenizer strT = new StringTokenizer(container, ";"); while (strT.hasMoreTokens()) { String nextToken = strT.nextToken(); if (nextToken.equals(item)) { return true; } } return false; }
public static String removeFromList(String item, String list) { String newList = ""; StringTokenizer listT = new StringTokenizer(list, ";"); while (listT.hasMoreTokens()) { String nextToken = listT.nextToken(); if (!nextToken.equals(item)) { newList += nextToken + ";"; } } return newList; }
public static void printHelp() { System.out.println("The commands that you can use: "); System.out.println(" exits - will print out all exits from current room"); System.out.println(" look - will display all (visible) items in the room"); System.out.println(" inventory - list the items that you are carrying"); System.out.println(" go dir - exit the room, where dir is east, west, north or south"); System.out.println(" quit - quit the game"); System.out.println(" pick up - pick up an item (another question will display)"); System.out.println(" drop - drop an item (another question will display)"); System.out.println(" help - this menu."); } }
End of code***********
The UML diagram of the Player class is the following Plaver - name String String oolean + Player (name : String, room int, inventory : String) + printInventor0 void + getContinuePla0: boolean +etName0 String + addToInventory(item String): void item : String) : void The Room class is a superclass that holds information about a room in the game. Do NOT modify the Room class in any wav. Do NOT add code. Do NOT remove code. Leave it as-is. The field roomNum is the ID of the room, and xoomName is the name of the room. The field roomQbiects is of type String, and holds a string representation of the items that are in the room, separated by a semicolon. The field roomExits, of type String, contains information about which of the cardinal points in the room (n for north, s for south, e for east, and w for west) lead to which other rooms in the game If a room has more than one exit, the exits in the roomExits string are separated by semicolons. The UML diagram of the Room class is shown below. As an example, to create a room object whose name is MeetingRm, has a room number ID of 3, contains chairs, a tables, and a professor, and that has arn exit on the north end leading to room 2 and an exit to the east leading to room 1, you would write Room oomnew Room ("MeetingRm", "chairsitableuprefesse", 3, "n2;e1"); The UML of the Room class is shown in the following diagram The UML diagram of the Player class is the following Plaver - name String String oolean + Player (name : String, room int, inventory : String) + printInventor0 void + getContinuePla0: boolean +etName0 String + addToInventory(item String): void item : String) : void The Room class is a superclass that holds information about a room in the game. Do NOT modify the Room class in any wav. Do NOT add code. Do NOT remove code. Leave it as-is. The field roomNum is the ID of the room, and xoomName is the name of the room. The field roomQbiects is of type String, and holds a string representation of the items that are in the room, separated by a semicolon. The field roomExits, of type String, contains information about which of the cardinal points in the room (n for north, s for south, e for east, and w for west) lead to which other rooms in the game If a room has more than one exit, the exits in the roomExits string are separated by semicolons. The UML diagram of the Room class is shown below. As an example, to create a room object whose name is MeetingRm, has a room number ID of 3, contains chairs, a tables, and a professor, and that has arn exit on the north end leading to room 2 and an exit to the east leading to room 1, you would write Room oomnew Room ("MeetingRm", "chairsitableuprefesse", 3, "n2;e1"); The UML of the Room class is shown in the following diagramStep by Step Solution
There are 3 Steps involved in it
Step: 1
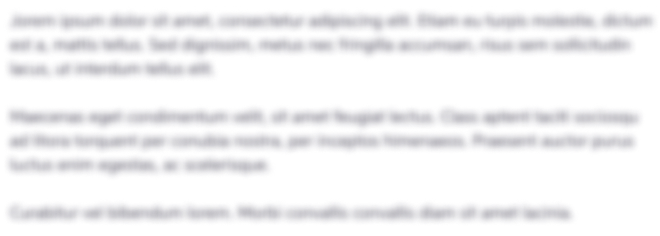
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started