Question
//********************************************************** // Proj4Test.cpp // An interactive test program for Programming Assignment 4 //********************************************************** #include // Provides toupper #include #include #include NumberType.h using namespace std; //
//**********************************************************
// Proj4Test.cpp
// An interactive test program for Programming Assignment 4
//**********************************************************
#include
#include
#include
#include "NumberType.h"
using namespace std;
// PROTOTYPES for functions used by this test program:
void printMenu();
// Post: A menu of choices for this program has been written to cout.
char getUserCommand();
// Post: The user has been prompted to enter a one character command.
// The next character has been read (skipping blanks and newline characters),
// and this character has been returned.
void skipToEnd();
// Post: Input has been read up to and including an end-of-line
// marker, and this input has been discarded.
int main()
{
int decNum;
char binNum[MAX_NUM_OF_BITS];
char choice; // a command character entered by the user
NumberType theNum;
do
{
printMenu( );
choice = toupper(getUserCommand( ));
switch (choice)
{
case '1':
cout << "Please enter a nonnegative decimal integer number: ";
cin >> decNum;
theNum.setDecNum(decNum);
cout << "The corresponding binary representation is: ";
theNum.getBinNum(binNum);
cout << binNum << endl;
break;
case '2':
cout << "Please enter a binary integer number: ";
cin >> binNum;
theNum.setBinNum(binNum);
cout << "The corresponding decimal representation is: ";
decNum = theNum.getDecNum();
cout << decNum << endl;
break;
case 'Q': cout << "Bye!" << endl;
break;
default: cout << choice << " is invalid." << endl;
}
}
while ((choice != 'Q'));
return 0;
}
void printMenu( )
// Library facilities used: iostream.h
{
cout << endl; // Print blank line before the menu
cout << "%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%" << endl;
cout << " DECIMAL-BINARY CONVERSION " << endl;
cout << "The following choices are available: " << endl;
cout << " 1 Convert a decimal number to binary" << endl;
cout << " 2 Convert a binary number to decimal" << endl;
cout << " Q Quit this test program" << endl;
cout << "%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%" << endl << endl;
}
char getUserCommand( )
// Library facilities used: iostream
{
char command;
cout << "Enter choice: ";
cin >> command; // Input of characters skips blanks and newline character
skipToEnd( );
return command;
}
void skipToEnd( )
{
while (cin && cin.peek( ) != ' ')
cin.ignore( );
cin.ignore( );
}
******************
HEADER
#ifndef NUMBERTYPE_H #define NUMBERTYPE_H #include
const int MAX_NUM_OF_BITS = 50; // Maximum number of bits allowed // for binary representation
class NumberType { public: // Constructor NumberType(int a = 0); // Post: num = a; binRep contains the corresponding // binary number bit sequence.
void setDecNum(int a); // Post: num = a; binRep contains the corresponding // binary number bit sequence. void setBinNum(char binNum[]); // Post: binRep contains the same bit sequence as bitNum; // num contains the corresponding decimal value.
int getDecNum(); // Return the value of num. void getBinNum(char binNum[]); // Post: bitNum contains the same bit sequence as bitRep.
protected: int num; // nonnegative decimal integer char binRep[MAX_NUM_OF_BITS]; // the corresponding binary // representation for num; we use a C-string to // represent the bit sequence
private: void dec2Bin(); // Decimal to binary conversion (use a LinkedStackType stack // in its implementation). // Post: binRep contains the bit sequence corresponding to // the decimal value num. void bin2Dec(); // Binary to decimal conversion. // Post: num contains the decimal value corresponding to // the binary representation bitRep. };
#endif
In this programming assignment, you will implement a new class called NumberType, using a header file NumberType.h (the members are specified and brief descriptions of operations are also given for you). You are to write the implementation file NumberType.cpp for the NumberType class.
You are to implement and test the following operations for the NumberType class. Since the operations are only briefly described in the header file NumberType.h, you may add more details to the precondition/postcondition contract of the member functions.
A constructor.
Two set functions.
Two get functions.
A decimal-to-binary conversion function.
A binary-to-decimal conversion function.
The NumberType class objects are used to represent nonnegative integers in both decimal and binary representations
Additional Requirements and Hints
The Protected Member Variables
Carefully read the class definition in NumberType.h. The NumberType class has a protected integer member num to store the nonnegative decimal integer number. It also has a protected C-string member binRep to store the binary representation corresponding to num. Here we use C-string to store binRep instead of using int such that binRep can accommodate possibly long binary bit sequences. At any time, num and binRep always correspond to each other in the sense that they are different representations of the same number using different bases.
The dec2Bin Function
Use the method of successive division (see the supplementary reading on positional-number-system conversions posted on Blackboard) to write this function. Use a stack (you may use array-based or linked-list-based stacks discussed in Lecture Handout 13) to facilitate the process of storing and retrieving the remainders. Do not use any available C++ built-in function which directly achieves such a conversion.
The bin2Dec Function
Write this function to implement the weighted sum formula for binary-to-decimal conversion (see the supplementary reading on positional-number-system conversions posted on Blackboard). Do not use any available C++ built-in function which directly achieves such a conversion.
Implement and Test Small Pieces
You may start by implementing what you can, using one or several member functions together with a simple test driver client program to test the functionality of the functions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
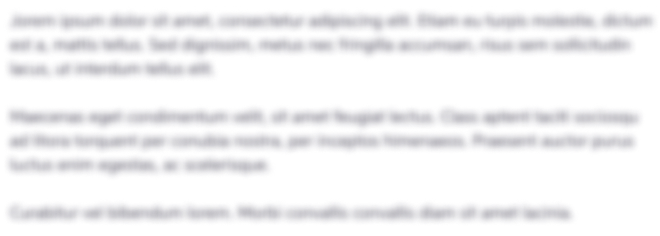
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started