Question
Project 08 Description For this lab you will write a Java program that manipulates numbers. The program will ask the user to enter a number
Project 08 Description
For this lab you will write a Java program that manipulates numbers. The program will ask the user to enter a number (or a negative number to quit) and then convert that non-negative number from base 10 into base 2 (binary) and display the result. The program will loop until the user enters a negative number to end the program. For this assignment you must start with the following "skeleton" of Java code. Import this into your Eclipse workspace and fill in the methods as directed. Feel free to add any methods you find useful, but make sure that you add comments indicating what they do following the form of the rest of the comments in the code. Project08.java ( in the end)
Binary Numbers and Decimal Numbers
You should be somewhat familiar with numbers in different "bases" from your earlier math classes. As a quick refresher, we can represent numbers by using different "bases". The "base" of a number system tells you how many different digits are used when representing values in that system. We call the standard system that we use every day the "base 10" system or "decimal system" because there are 10 digits used to represent numbers (0, 1, 2, 3, 4, 5, 6, 7, 8, and 9 - decimal because "deci" is the Latin prefix meaing "10"). In a "base 2" or "binary" system we use only two digits - "0" and "1". The binary number system has a special place in computing because it is very easy to build logic gates using voltages that are either "high" or "low" - representing "1" and "0" (or "true" and "false", or "on" and "off"). It would be much more difficult - and much more error-prone - to try to use logic gates that represent more than just the two simple values. Fortunately we can easily convert between bases. The choice of base 10 as our "standard" base is fairly arbitrary (and likely comes from the fact that we have 10 fingers - which are also known as "digits" - on each hand) and there are simple algorithms to convert back and forth between bases. For this project you will be coding one of them. Binary representation can be best explained through an analogy to decimal representation. Consider the number 1234. When we look at this number and break down what the individual digits represent, we know through many years of education what it means. It means that we have 1 "thousand" plus 2 "hundreds" plus 3 "tens" plus 4 "ones". To put it another way, each position in the string of digits (known as a "place") represents 10 raised to a power. From right to left we have 1, 10, 100, and 1000 - or to better see the pattern - 100, 101, 102, and 103. Binary representation works exactly the same way, except instead of 10 we use 2 and instead of using 10 digits we use only 2 of them. So we might have a number like 1101. Here this means that we have 1 "eight" plus 1 "four" plus 0 "twos" plus 1 "one". Or more formally 1*23 + 1*22 + 0*21 + 1*20. The total here, represented as a decimal, would be 13 - so the string "1101", read as a binary number, represents the same value as the string "13" read as a decimal number does. Each place in a binary number holds a value for a power of two, just as each place in a decimal number holds a value for a power of 10. Wikipedia has much more on the topic of binary numbers if you are interested.
Converting from Decimal to Binary
One algorithm for converting from a decimal number to a binary number is:
Start with an empty String to contain your binary value
While your decimal number is not zero
Get the remainder of what your current decimal number would be when divided by 2. Prepend this result to the front of your String.
Divide your decimal number by 2 and repeat.
So for example, the number 13 could be converted to a binary value as follows
Start with an empty String: ""
The number 13 != 0
13 % 2 = 1
Prepend 1 to the front of our String: "1"+"" = "1"
13 / 2 = 6 (Remember - integer division here, no fractions)
The number 6 != 0
6 % 2 = 0
Prepend 0 to the front of our String "0" + "1" = "01"
6 / 2 = 3
The number 3 != 0
3 % 2 = 1
Prepend 1 to the front of our String "1" + "01" = "101"
3 / 2 = 1
The number 1 != 0
1 % 2 = 1
Prepend 1 to the front of our String "1" + "101" = "1101"
1 / 2 = 0
The number 0 == 0
Our final result is: "1101"
Verify your result above by converting it back - 1*23 + 1*22 + 0*21 + 1*20 = 8 + 4 + 0 + 1 = 13.
Project 08 Sample Output
This is a sample transcript of what your program should do. Items in bold are user input and should not be put on the screen by your program. Converting from Decimal to Binary Enter an integer value (negative value to quit): 1984 The decimal value 1984 is 11111000000 in binary. Enter an integer value (negative value to quit): 10 The decimal value 10 is 1010 in binary. Enter an integer value (negative value to quit): 4000 The decimal value 4000 is 111110100000 in binary. Enter an integer value (negative value to quit): 123s12 ERROR - value must be non-negative and contain only digits Enter an integer value (negative value to quit): 0 The decimal value 0 is 0 in binary. Enter an integer value (negative value to quit): -1 Goodbye Note that your output depends on the choices made by the user. You are also required to check that the user has entered an actual numeric value and report an error message if they do not (see the description of methods in the project skeleton for details on this).
/** * Project08.java * * A program that converts decimal numbers into binary numbers. * Used to practice breaking code up into methods. * * @author YOUR NAME HERE */ package osu.cse1223; import java.util.Scanner; public class Project08{ public static void main(String[] args) { // TODO: Fill in the body } /** * Given a Scanner as input, prompt the user to enter an integer value. Use * the function checkForValidDecimal below to make sure that the value * entered is actually a decimal value and not junk. Then return the value * entered by the user as an integer to the calling method. * * @param input * A scanner to take user input from * @return a valid integer value read from the user */ public static int promptForDecimal(Scanner input) { System.out.print("Enter an integer value (negative value to quit): "); String val = input.nextLine(); while (!checkForValidDecimal(val)) { System.out.println( "ERROR - value must be non-negative and contain only digits"); System.out.print( "Enter an integer value (negative value to quite): "); val = input.nextLine(); } return Integer.parseInt(val); } /** * Given a String as input, return true if the String represents a valid * integer value (i.e. contains only digits, with possibly a '-' character * in the first position indicating a negative value). Returns false if the * String does not represent an integer value. * * Note that you must NOT use Exception Handling to implement this. If you * search on the net for solutions, that is what you will find but you * should not use it here - you can solve this using only what we have * discussed in class. * * Note too that your code does not need to worry about the number * represented being too large or too small (more than Integer.MAX_VALUE or * less than Integer.MIN_VALUE. Just worry about the constraints given above * for now (but for a challenge, try to figure out how to do it using only * what you have learned so far in class - it is not a simple problem to * solve.) * * @param value * A String value that may contain an integer input * @return true if the String value contains an integer input, false * otherwise */ public static boolean checkForValidDecimal(String value) { // TODO: Fill in the body } /** * Given an integer value, return a String that is the binary representation * of that value. Your implementation must use the algorithm described in * the Project 8 write-up. Other algorithms will receive no credit. * * @param value * An integer value to convert to binary * @return A String containing the binary representation of value */ public static String decimalToBinary(int value) { // TODO: Fill in the body } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
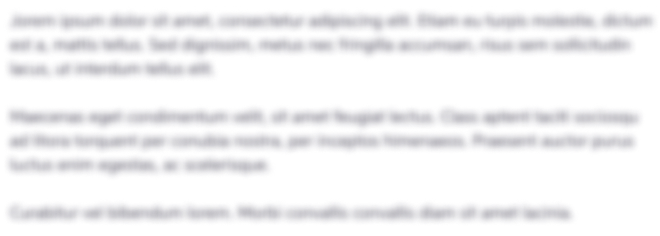
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started