Question
Project 10 This is C++. Please upload all code files as text. Write the definition of a class, swimmingPool, to model the properties of a
Project 10 This is C++. Please upload all code files as text.
Write the definition of a class, swimmingPool, to model the properties of a swimming pool as an abstract data type.The pool object will be represented by the following properties: length, width, and depth of the pool (notice that our model represents a swimming pool object as a simple rectangular box). We will also include as properties the rate (in gallons per minunte) at which water can fill the pool and the rate (in gallons per minute) at which water can be drained from the pool. In addition to constructors and getters and setters for the private instance variables, we also want the pool object to be able to provide the following information: the current amount of water in the pool, the amount of water needed to fill an empty or partially filled pool and the amount of time needed to completely or partially fill or empty the pool. We also will include modifier functions to add or drain water for a specific amount of time. We want to give the pool object complete control over its data (i.e., the amount of water it holds) so the only way to change the amount of water in the pool will be through these modifier functions. In order to maintain this control, our pool object will follow the following rules:
The amount of water currently in the pool is stored in a private instance variable, amountOfWaterInPool.
The amount of water in the pool cannot be < 0 or > than the capacity of the pool as determined by its length, width, and depth.
If the pool is drained below 0, set the amount of water to 0
If the pool is filled over capacity, set the amount of water to the maximum and display an error message.
The only way to add water to the pool is to fill it for a specified period of time at a specified flow rate.
The only way to remove water from the pool is to drain it for a specified period of time at a specified flow rate.
These rules are sometimes referred to as the class invariant. It is the responsibility of the class builder to ensure that the class invariant is not violated. In addition, these rules are the guidelines the programmer uses to determine the preconditions for the modifier functions. If the class builder takes care to ensure that the invariant is maintained, then he/she can guarantee that the postconditions for the member functions will be met. It is the responsibility of the class user to follow the preconditions when requesting modifications to the private instance variables. This division of responsibility is sometimes called the precondition - postcondition contract. The class builder, however, should never assume that the class user will follow the preconditions, so the class builder has the ultimate responsibility to prevent violations of the class invariant and can refuse to assign invalid values to the private instance variables.
To help with the design of the class, a UML diagram is provided:
swimmingPool class |
- length: double - width: double - depth: double - amountOfWaterInPool: double - flowRateIn: double - flowRateOut: double |
+ getLength(): const double + getWidth(): const double + getDepth(): const double + getFlowRateIn(): const double + getFlowRateOut(): const double + setLength(double): void + setWidth(double): void + setDepth(double): void + setFlowRateIn(double): void + setFlowRateOut(double): void + print(): const void + getAmountOfWaterInPool(): const double + getPoolTotalCapacity(): const double + getTimeToFillPool(): const double + getTimeToDrainPool(): const double + getAmountNeededToFill(): const double + addWater(double, double): void + drainWater(double, double): void + swimmingPool(double, double, double, double, double) |
The member function getPoolTotalCapacity() returns the maximum volume of water the pool can hold. Use the constant 7.48 gallons per cubic foot to calculate this value. The constructor is set up to assign default values for the length, width, depth, flow rate in and flow rate out. It should also initialize the amount of water in the pool to 0.
Using the UML diagram develop the header for this class. The header must contain comments for each member function describing the input to the function, any preconditions that exist on the input, and the postconditions for each function. Develop the class implementation file taking care to detect and handle precondition violations. Design a test program that performs the following actions:
Create a pool object.
Display the properties of the pool (print).
Display the total capacity of the pool
Display the amount of water needed to fill the pool completely.
Display the amount of time needed to fill the pool completely.
Fill the pool to capacity and display the current water amount.
Display the amount of time needed to drain the pool completely.
Drain half the water from the pool and display its current water amount.
Display the amount of time required to fill the pool.
Add water for 3 hours and display the current water amount.
Fill the pool over capacity and verify that you get an error message.
Turn in:
swmmingPool.h
swimmingPool.cpp
swimmingPoolTest.cpp
One or more screen shots showing the results of your testing.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
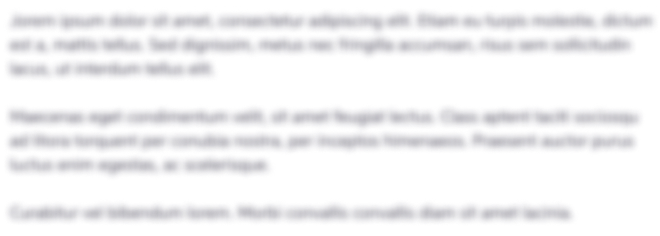
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started