Question
Project 2 - BattleCode Part 1 Introduction You will build a turn-based battle game called BattleCode. The characters in the game are called Heroes. Heroes
Project 2 - BattleCode Part 1
Introduction
You will build a turn-based battle game called BattleCode. The characters in the game are called Heroes. Heroes have four traits: strength, agility, health, and stamina. These factors, and some luck, will ultimately decide the outcome of a battle. In this assignment you will only build part of the larger game - more to come later
Game Play
Setup
The game starts by initializing Heroes. Heroes have 4 properties: strength, agility, stamina, and health. By default, Health is set to 50 and stamina is set to 25, but strength and agility must be initialized by following the instructions below. Strength helps with attacking and agility helps with blocking.
Hero Initialization
Roll one 12-sided die, one 10-sided die, and one 8-sided die. Assign the highest value to strength. Set agility to the sum of the other two values.
Rounds and Moves
A round consists of each player selecting a move and calculating the resulting damage. Each player must select a move for their hero. Hero objects can attack or defend. Each move requires stamina. If the hero cannot afford the stamina cost, it must rest.
Once both players select a move damage is calculated.
Attacking and resting provide no defense. If you are attacked in these states, you receive full damage
resting adds health, which mitigates damage, but it is not the same as blocking
Blocking provides defense. If a hero is attacked when blocking, damages could be averted
If the block value is greater than or equal to the attack value, the attack is dodged
Otherwise the difference between the attack and the block is the damage.
You will need to build the following classes:
Die Class
The die class should have the following fields and methods.
a field called value that stores the face value of the die
a field called sides that stores the number of side on a die
a method to get the sides
a method to get the value
a roll method that sets the value using random number generation and the number of sides on the die (Roll is the only way to change the value of the die)
a single constructor that the number of sides as input (once the number of sides is set, it does not change)
Hero Class
The Hero is a data class. It contains all the information and behaviors of a Hero. It uses the Die class to complete some actions
A hero has the following fields:
a string for the Hero name
integers to represent each of the following: health, stamina, strength, and agility
public static final integers to represent the stamina cost of attacking (6), the stamina cost of defending (4), the default values for health (50), stamina (25), strength (5), and agility (5) if none are specified.
A hero should have two constructors:
one that takes just a string representing the name
one that takes a string for the name and two integers, one for strength and one for agility
all other fields should be initialized to the default values using the final variables stated above
A hero performs the following actions:
getName - return the string name
setName- assign a value to the string name
getHealth - return the int health
setStrength - assign a value to strength, cannot be negative
getStrength - return strength
setAgility - assign a value to agility, cannot be negative
getAgility - return the int agility
setStamina - assign a value to stamina, cannot be negative
getStamina - return the int stamina
setHealth - assign a value to the int health
addHealth - add an int value to health, the value added cannot be negative
removeHealth - subtract an int value from health, the value subtracted cannot be negative
printStats - print the hero name, strength, agility, stamina, and health to the screen
defend - returns the int value calculated by the table below - if stamina is less than move cost, opponent must rest and zero is returned
attack - returns the int value calculated by the table below - if stamina is less than move cost, opponent must rest and zero is returned
rest - returns void, adds to health and stamina based on table below, should be private and triggered by attack/defend
Move | Action | Calculation | Stamina Cost |
---|---|---|---|
Attack | User selected, attacks opponent | Roll a 20-sided die. The attack value is the strength plus the roll. If the Hero cannot meet the stamina cost, it must rest, and the attack value is zero | 6 |
Defend | User selected, defends from opponent attack | Roll a 20-sided die. The defend value is the agility plus the die roll. If the Hero cannot meet the stamina cost, it must rest, and the attack value is zero | 4 |
Rest | triggered when stamina is depleted | When a player tries to attack/defend but is out of stamina, roll a 6 sided, an 8-sided die, and a 10-sided die. Add the highest value to health. Add the sum of the other two to stamina. | 0 |
BattleManager Class
A battleManager is a management class. It handles all the interactions between hero objects. It also uses the Die class. A battle manager needs the following methods:
setupHero - Takes a string, the name of the hero, as a parameter. The method uses the initialization instructions above to create and initialize a hero object. The method returns the hero object that was created.
round - takes two hero objects, returns void. Allows user to select moves, performs the moves, and applies damage. print any info you would like upon completion.
You may have any other private methods you like
Testing Classes
Write a class to test everything:
Create a new BattleManager
Use the BattleManager to setup 2 new Heroes.
Print the stats of each hero
Call the round method using the heroes as input paramters
Print the stats of each hero
Call the round method using the heroes as inputparamters
Print the stats of each hero
Compare the health of the two heroes. Print the name of the hero with the most health and declare them the winner.
Instructions
Create a new NetBeans project called Projec2_XXXXXX, where XXXXXX is your student ID
Define the classes and test your program as stated above
Be sure your code complies with the style and documentation guide
Be sure your classes are encapsulated.
Comments required.
Code Needed In Java Please.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
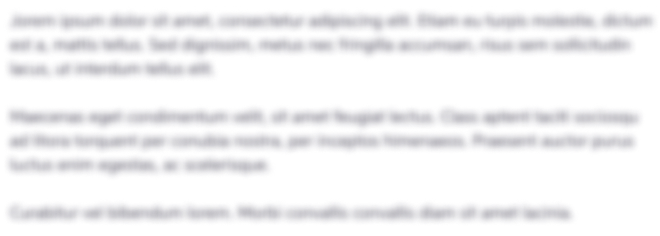
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started