Question
Project 3 Parking Deck Ticketing System Objectives: Use if, switch, and loop statements to solve a problem. Use input and output statements to model a
Project 3 Parking Deck Ticketing System
Objectives:
Use if, switch, and loop statements to solve a problem.
Use input and output statements to model a real world application
Incorporate functions to divide the program into smaller segments
Instructions:
Your task is to write a program that simulates a parking meter within the parking deck. The program will start by reading in the time a car arrives in the parking deck. It will then ask you what time the car left the parking deck. You should then calculate the time that has passed between issuing the time ticket and the time the car left. Lastly, you will use this information to determine how much the person needs to pay upon leaving the deck. The rates are in the table below. Please read through all of the notes below!
You should write a single function for each of the following things:
Reading in the arrival times and departure times
Calculate the passage of time
Determine how much the player needs to pay
Print out the receipt for the user. Including their arrival and departure times, time in the deck and how much they owe.
Notes:
Time should be handled in the 24 hour format for simplicity. In addition, you should put the data on a file with the hours and the minutes as two separate variables separated by a space. Please have the hours ranging from 0 to 23, and minutes ranging from 0 to 59 with a blank in between hours and minutes. See the second table below for the data.
You may assume that the parking deck is open 24/7 for simplicity. You do not need to worry about times the deck closes and such for this project.
If the drive has a special parking pass, please enter the time of 99 for the hour and 99 for the minutes when entering the deck. This should be used as a code for the system to know that this person has a special parking pass.
If the drive has lost their ticket, please input 55 for the hour and 55 for the minutes when exiting the deck. This will prompt the system to handle the payments without a ticketing stub.
Please make sure that the output is attractive and informative. It should include, but is not limited to: the time the ticket was issued the time the ticket was entered back into the machine (time the drive exited the deck.) You should also include the amount of time that transpired while the driver was in the deck and the amount of money due to pay back. Please use proper formatting techniques for output (fixed and set precision, remember we are talking about money.)
Rate Table:
Time in Parking Deck | Rate in Dollars ($) |
Less than or equal to 30 minutes | 3.00 |
> 30 Minutes <= 1 Hour) | 5.00 |
>1 Hour <= 2 Hours ) | 10.00 |
>2 Hours <= 3 Hours ) | 15.00 |
>3 Hours <= 4 Hours ) | 30.00 |
Each half hour over four hours <= 12 hours | 30.00 + 5.00 per additional half hour |
>12 Hours <= 24 Hours ) | Error prints out, see notes above. |
Lost ticket | 110.00 |
Special Parking Pass | 5.00 |
Running:
Please run the following sets of data from an input file:
| Entrance Hour | Entrance Minute | Exit Hour | Exit Minute |
Run 1 | 00 | 00 | 00 | 30 |
Run 2 | 05 | 45 | 07 | 00 |
Run 3 | 06 | 32 | 09 | 54 |
Run 4 | 09 | 15 | 12 | 15 |
Run 5 | 09 | 32 | 14 | 35 |
Run 6 | 08 | 00 | 10 | 30 |
Run 7 | 08 | 45 | 55 | 55 |
Run 8 | 99 | 99 | 99 | 99 |
Submitting:
Please include this document at the front of your project folder. This should be followed by the algorithm for your program. Next please include your source code, followed by all of your output. Lastly, please include your academic honesty promise. Please put all of these documents in a zipped folder and submit to D2L.
Extra Credit:
What happens if you were to arrive at 5:00 PM and leave at 4:00 AM? Would you program still run this correctly? Make sure that you can account for this sort of issue.
More Information on Project 3
If the ticket is lost (code 99), charge the patron $110.00. If the patron has a parking pass (code 55), the charge is $5.00. If the ticket shows more than 12 hours in the parking deck, print ERROR in the column where the charge usually goes. If you do the extra credit (in at 17:00 hours (5PM)and out at 4:00 (4AM)), the charge will be $100.00 for 11 hours. Present the answers in a table with column headings of entry hours & min (could be 99), exit hours & min (could be 99 or 55), total minutes in the parking deck (leave blank for codes of 99 and 55), and total cost of parking.
Answers are: 3.00, 10.00, 30.00, 15.00, 45.00, 15.00, 110.00, 5.00, ERROR, and 100.00(extra credit).
This is what I have so far. I need help in C++
#include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
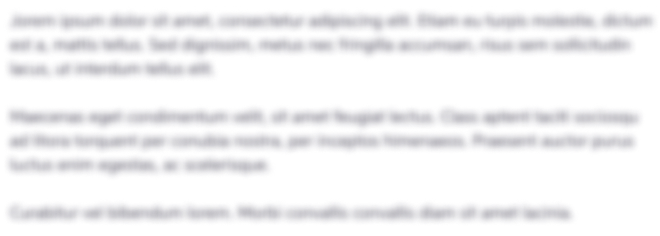
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started