Question
project 8 Change the program to use classes for temperature, wind, and weather measurement. Please tell me where the changes have been made. Thank you
project 8
Change the program to use classes for temperature, wind, and weather measurement.
Please tell me where the changes have been made. Thank you
C++ code:
EdwardMagruderWeatherStation.cpp
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
#include "weather.h"
using namespace std;
string DisplayMenu(string station_name)
{
string str, temp;
do
{
cout << "*******************WEATHER STATION: " << station_name\
<< " *******************" << endl << endl;
cout << "I. Input a complete weather reading." << endl;
cout << "P. Print the current weather." << " ";
cout << "H. Print the weather history (from most recent to oldest)." << endl;
cout << "E. Exit the program." << " ";
cout << "Enter your choice: " << endl;
cin >> str;
temp = str;
for (std::string::size_type i = 0; i < str.length(); ++i)
temp[i] = toupper(str[i]);
str = temp;
} while (!(str == "I" || str == "P" || str == "H" || str == "E"));
return str;
}
/*
double getTemperature()
{
double temp;
string temp_string;
stringstream converter;
cout << "Enter the temperature: ";
cin >> temp_string;
converter << temp_string;
converter >> temp;
return temp;
}
double getWindSpeed()
{
double temp;
string temp_string;
stringstream converter;
//this loop will be iterated continuously untill user enters windspeed which is greater than zero
do
{
cout << "Enter Wind speed(>=0): ";
cin >> temp_string;
converter << temp_string;
converter >> temp;
if (temp <= 0)
cout << "Wind speed should be always greater or equal to 0(zero)";
} while (temp < 0);
return temp;
}
string getWindDirection()
{
string temp_string, temp;
do {
cout << "Enter the Wind Direction (North,South,East,West): ";
cin >> temp_string;
temp = temp_string;
for (std::string::size_type i = 0; i < temp_string.length(); ++i)
temp[i] = toupper(temp_string[i]);
} while (!(temp == "NORTH" || temp == "SOUTH" || temp == "EAST" || temp == "WEST"));
temp_string = temp;
return temp_string;
};
void printWeather(Weather_Station ws)
{
cout << "Station Name " << ws.name << endl;
cout << "Temperature " << ws.temperatureMeasure.temperature << endl;
cout << "Wind Direction " << ws.windMeasure.windDirection << endl;
cout << "Wind Speed " << ws.windMeasure.windspeed << endl;
cout << endl;
}
*/
int main()
{
//Have the user provide a name for the weather station upon entry.
weather_t * history;
int historySize;
string station_name, input_choice;
int histCount = 0;
cout << "Enter the name of Weather Station: ";
getline(cin, station_name);
do {
cout << "Please enter how many records you want" << " ";
cin >> historySize;
if (historySize <= 0)
cout << "Input record count should always be greater than 0(zero)" << " ";
} while (historySize <= 0);
history = new weather_t[historySize];
while (1)
{
//Control loop to perform various actions
input_choice = DisplayMenu(station_name);
if (input_choice == "I")
{
/*
// get the details
int valid_wind_direction = 0, valid_wind_speed = 0;
myWeather_Details.temperatureMeasure.temperature = getTemperature(); // get temperature
myWeather_Details.windMeasure.windDirection = getWindDirection(); //get wind direction
myWeather_Details.windMeasure.windspeed = getWindSpeed(); //get wind direction
if (myWeather_Details.windMeasure.windspeed >= 0)
{
valid_wind_speed = 1;
}
if ((myWeather_Details.windMeasure.windDirection == "NORTH") ||
(myWeather_Details.windMeasure.windDirection == "SOUTH") ||
(myWeather_Details.windMeasure.windDirection == "EAST") ||
(myWeather_Details.windMeasure.windDirection == "WEST"))
{
valid_wind_direction = 1;
}
//store the details
if (valid_wind_direction && valid_wind_speed)
myStation.push_back(myWeather_Details);
*/
for (int i = historySize - 1; i > 0; i--) {
history[i] = history[i - 1];
}
history[0] = getWeather();
histCount++;
}
else if (input_choice == "P")
{
cout << "*************Printing Current Weather*************" << endl;
if (histCount > 0) {
printWeather(history[0]);
}
else {
cout << "no data has been entered" << " ";
}
}
else if (input_choice == "H")
{
cout << "*************Printing Current Weather*************" << endl;
if (histCount == 0)
cout << "no data to output" << " ";
//this loop will be iterated continuously untill user gives the input count more than 0 and it is not greater than available record count in vector
int max = histCount > historySize ? historySize : histCount;
for (int i = 0; i < max; i++) {
printWeather(history[i]);
}
}
else if (input_choice == "E")
{
exit(0);
}
}
return 0;
}
temperature.cpp
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
#include "temperature.h"
using namespace std;
temperature_t getTemperature()
{
temperature_t temp;
string temp_string;
stringstream converter;
cout << "Enter the temperature: ";
cin >> temp_string;
converter << temp_string;
converter >> temp.temperature;
return temp;
}
weather.cpp
#include "stdafx.h"
#include "temperature.h"
#include
#include
#include "weather.h"
void printWeather(weather_t ws)
{
//cout << "Station Name " << ws.name << " ";
cout << "Temperature " << ws.temperature.temperature << " ";
cout << "Wind Direction " << ws.wind.direction << " ";
cout << "Wind Speed " << ws.wind.speed << " ";
cout << " ";
}
weather_t getWeather() {
weather_t result;
result.wind = getWind();
result.temperature = getTemperature();
return result;
}
wind.cpp
#include "stdafx.h"
#include "wind.h"
#include
#include
#include
#include
#include
#include
using namespace std;
double getWindSpeed()
{
double temp = -1;
string temp_string;
stringstream converter;
//this loop will be iterated continuously untill user enters windspeed which is greater than zero
do
{
cout << "Enter Wind speed(>=0): ";
cin >> temp_string;
stringstream(temp_string) >> temp;
if (temp < 0)
cout << "Wind speed should be always greater or equal to 0(zero)";
} while (temp < 0);
return temp;
}
string getWindDirection()
{
string temp_string, temp;
do {
cout << "Enter the Wind Direction (North,South,East,West): ";
cin >> temp_string;
temp = temp_string;
for (std::string::size_type i = 0; i < temp_string.length(); ++i)
temp[i] = toupper(temp_string[i]);
} while (!(temp == "NORTH" || temp == "SOUTH" || temp == "EAST" || temp =="WEST"));
temp_string = temp;
return temp_string;
};
wind_t getWind() {
wind_t result;
result.speed = getWindSpeed();
result.direction = getWindDirection();
return result;
}
temperature.h
#pragma once
struct temperature_t {
int temperature;
};
temperature_t getTemperature();
weather.h
#pragma once
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
#include "wind.h"
#include "temperature.h"
using namespace std;
struct weather_t {
wind_t wind;
temperature_t temperature;
};
weather_t getWeather();
void printWeather(weather_t ws);
wind.h
#include
#pragma once
struct wind_t {
double speed;
std::string direction;
};
wind_t getWind();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
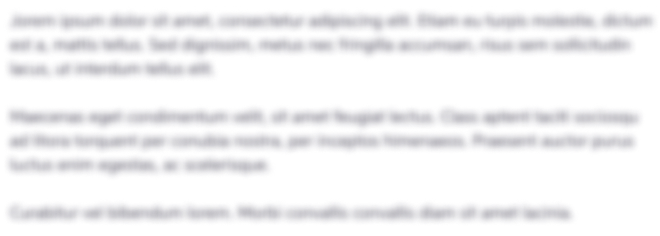
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started