Question
Project Management Program, python File: project.py File: project_management.py Read the instructions, then enter a time estimate in your module docstring. The instructions for this exercise
Project Management Program, python
File: project.py File: project_management.py
Read the instructions, then enter a time estimate in your module docstring.
The instructions for this exercise are intentionally not step-by-step. We want you to use what you've learned about good program and class design, iterative development, version control, clean code, etc. to determine the best approach.
Save the projects.txt data file to your prac folder. This file contains the data for this exercise delimited by tabs. The first line is a header, explaining the fields for each project.
Write a program in project_management.py to load and save a data file and use a list of Project objects.
Load projects from the data file when the program starts and save them to it when the user quits.
Your program should contain a menu with the following options:
Load projects (Prompt the user for a filename to load projects from and load them)
Save projects (Prompt the user for a filename to save projects to and save them)
Display projects (Display two groups: incomplete projects; completed projects, both sorted by priority)
Filter projects by date (Ask the user for a date and display only projects that start after that date, sorted by date)
Add new project (Ask the user for the inputs and add a new project to memory)
Update project (Choose a project, then modify the completion % and/or priority - leave blank to retain existing values)
Expectations:
Commit your work as you go (iterative development)
Some of this could be considered relatively "hard" - do the easy bits first, then add more as you go
Use the datetime module for the project start date
Write your class such that you are able to sort/compare Project objects based on priority order
Think about writing utility/helper methods in your class and main program. Think of our examples like is_vintage for Guitar and what you might use for a Project.
Follow good design principles like SRP and DRY. Notice that there's two kinds of loading and write one function to handle both. Same for saving.
Write good clean code (no pylint warnings) with good naming and design (as always!)
Here are two suggestions to leave until last (iterative development):
Error checking. Do no error checking to start with.
Date formatting. Just use a string until most everything else works, then, here are some suggestions.
The following code reads a string from user input, converts it to a date object (using the strptime method from the datetime type), prints the day of the week (%A) and then prints the date as a string:
import datetime date_string = input("Date (d/m/yyyy): ") # e.g., "30/9/2022" date = datetime.datetime.strptime(date_string, "%d/%m/%Y").date() print(f"That day is/was {date.strftime('%A')}") print(date.strftime("%d/%m/%Y"))
Remember to enter your actual time in your module docstring. Are you getting better at estimating?
Is this exercise too big? Will it take too long? Probably. Not every student can do every task in every subject. Some things are challenging and that's OK.
Be systematic, thoughtful, use the patterns you've learned, copy from previous examples... be efficient. Don't waste time reinventing new/different ways to do "standard" things like menus. Follow the patterns.
Sample output
- (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> d Incomplete projects: Organise Pantry, start: 20/07/2022, priority 1, estimate: $25.00, completion: 55% Build Car Park, start: 12/09/2021, priority 2, estimate: $600000.00, completion: 95% Mow Lawn, start: 31/10/2022, priority 3, estimate: $3.00, completion: 0% Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 0% Completed projects: Read 7 Habits Book, start: 13/12/2021, priority 6, estimate: $99.00, completion: 100% - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> u 0 Build Car Park, start: 12/09/2021, priority 2, estimate: $600000.00, completion: 95% 1 Mow Lawn, start: 31/10/2022, priority 3, estimate: $3.00, completion: 0% 2 Organise Pantry, start: 20/07/2022, priority 1, estimate: $25.00, completion: 55% 3 Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 0% 4 Read 7 Habits Book, start: 13/12/2021, priority 6, estimate: $99.00, completion: 100% Project choice: 3 Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 0% New Percentage: 20 New Priority: - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> a Let's add a new project Name: Practical 7 Start date (dd/mm/yy): 1/11/2022 Priority: 1 Cost estimate: $0 Percent complete: 32 - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> f Show projects that start after date (dd/mm/yy): 20/7/2022 Organise Pantry, start: 20/07/2022, priority 1, estimate: $25.00, completion: 55% Mow Lawn, start: 31/10/2022, priority 3, estimate: $3.00, completion: 0% Practical 7, start: 01/11/2022, priority 1, estimate: $0.00, completion: 32% Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 20% - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> u 0 Build Car Park, start: 12/09/2021, priority 2, estimate: $600000.00, completion: 95% 1 Mow Lawn, start: 31/10/2022, priority 3, estimate: $3.00, completion: 0% 2 Organise Pantry, start: 20/07/2022, priority 1, estimate: $25.00, completion: 55% 3 Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 20% 4 Read 7 Habits Book, start: 13/12/2021, priority 6, estimate: $99.00, completion: 100% 5 Practical 7, start: 01/11/2022, priority 1, estimate: $0.00, completion: 32% Project choice: 0 Build Car Park, start: 12/09/2021, priority 2, estimate: $600000.00, completion: 95% New Percentage: 100 New Priority: 3 - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> d Incomplete projects: Organise Pantry, start: 20/07/2022, priority 1, estimate: $25.00, completion: 55% Practical 7, start: 01/11/2022, priority 1, estimate: $0.00, completion: 32% Mow Lawn, start: 31/10/2022, priority 3, estimate: $3.00, completion: 0% Record Music Video, start: 01/12/2022, priority 9, estimate: $250000.00, completion: 20% Completed projects: Build Car Park, start: 12/09/2021, priority 3, estimate: $600000.00, completion: 100% Read 7 Habits Book, start: 13/12/2021, priority 6, estimate: $99.00, completion: 100% - (L)oad projects - (S)ave projects - (D)isplay projects - (F)ilter projects by date - (A)dd new project - (U)pdate project - (Q)uit >>> q Thank you for using custom-built project management software.
Name Start Date Priority Cost Estimate Completion Percentage Build Car Park 12/09/2021 2 600000.0 95 Mow Lawn 31/10/2022 3 3.0 0 Organise Pantry 20/07/2022 1 25.0 55 Record Music Video 01/12/2022 9 250000.0 0 Read 7 Habits Book 13/12/2021 6 99.0 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
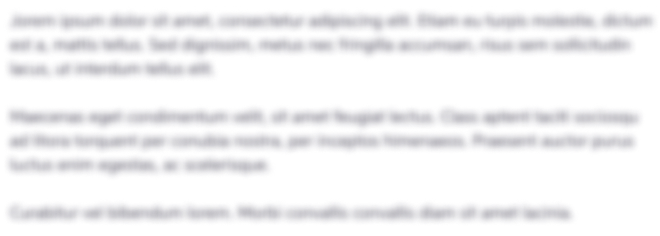
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started