Question
PROJECT Simple Java GUI: Salary Calculator Objective To type a simple Java program, execute ( run ) the program for some particular values, observe the
PROJECT Simple Java GUI: Salary Calculator
Objective To type a simple Java program, execute ( run ) the program for some particular values, observe the output and then modify the program.
PROJECT DESCRIPTION
Design a program that uses Java Graphical User Interface ( GUI ) components such as frames, text boxes, labels, checkboxes, radio buttons and click buttons.
The initial starter code for your program is given in Figure 1 .
First run and test the starter code, which primarily has Java GUI elements and has you enter a number into each of two textboxes ( input ) and then clicking a button to observe the sum of the numbers that appears within another textbox ( output ) .
After you test the original starter code and verify its functionality, you will then modify the program according to the instructions that follow.
Your completed program will perform the following tasks
enter a current salary into the appropriate text field
enter a decimal salary rate of increase into the appropriate text field
select the checkbox, if required
click the [ COMPUTE ] button
observe the new salary (with bonus if applicable )
Basically, your program is outlined in the given starter code statements shown within Figure 1 , which follows. Review the starter code to understand the mechanisms of the interactions between the two classes. Perform any modifications according to this projects instructions.
Type, compile and run the basic Java program that is shown in Figure 1 , which follows. Then compile and run your program, observe the output then modify the program.
Information About This Project
GUI objects in Java include these elements.
frames and containers to enclose other GUI objects
buttons to perform an event
check boxes for mutually inclusive selections
labels to display information
radio buttons for mutually exclusive selections
textboxes to enter or display data
Steps to Complete This Project
STEP 1 Open NetBeans or Eclipse or Similar Java - Based Editor
Open NetBeans or Eclipse or Similar Java - Based Editor and create a Java project with the following details:
For Project Name include: SimpleGUI For the Main Class include: SimpleGUI
In your Code window for this class, shown below, copy the program code shown in Figure 1 below, in the appropriate places, except substitute your own name in place of Sammy Student. PROJECT Simple Java GUI: Salary Calculator
Figure 1 Source Code for the Simple GUI Program
import java.awt.*; import java.awt.event.*; import javax.swing.*; //Sammy Student public class SimpleGUI extends JFrame implements ActionListener { private static final long serialVersionUID = 1L; JLabel l1, l2, l3; JButton b1; JTextField t1, t2, t3; SimpleGUI() { l1 = new JLabel(" INPUT 1"); l2 = new JLabel(" INPUT 2"); l3 = new JLabel(" OUTPUT"); b1 = new JButton("BUTTON 1"); t1 = new JTextField(10); t2 = new JTextField(10); t3 = new JTextField(10); add(l1); add(t1); add(l2); add(t2); add(l3); add(t3); add(b1); b1.addActionListener(this); setSize(500,300); setLayout(new GridLayout(4,2)); setTitle("Simple Java GUI"); }
public void actionPerformed(ActionEvent ae) { float a, b, c; if(ae.getSource() == b1) { a = Float.parseFloat(t1.getText()); b = Float.parseFloat(t2.getText()); c = a + b; t3.setText(String.valueOf(c)); } } PROJECT Simple Java GUI: Salary Calculator
Figure 1 Source Code for the Simple GUI Program ( continued )
public static void main(String args[]) { SimpleGUI a = new SimpleGUI(); a.setVisible(true); a.setLocation(200, 200); } }
STEP 2 Build, Compile and Run the Program From the menu select [ Run ] and click [ Run Project ] to run your app.
STEP 3 Test the Program Once you have successfully compiled your program, review the graphical user interface that appears, as shown in Figure 2 . This represents a sample run of the program.
Figure 2 Java Swing Interface
With the program running enter valid values in the input text boxes that appear. Click the button and observe the output.
PROJECT Simple Java GUI: Salary Calculator
STEP 4 Modify the Program For your first modification of the program you are to a new click button element to your graphical user interface. The purpose of this new button is to allow the user to exit the application.
To do this, perform the following tasks:
Declare a new button by supplementing the JButton declaration statement to include a new button named b2 .
JButton b1, b2;
In an appropriate location in your program code, instantiate the new button, named b2 .
b2 = new JButton("EXIT");
In an appropriate location in your program code and directly below the line of code
add(b1);
place the new button on your GUI grid by writing this statement:
add(b2);
In an appropriate location in your program code and directly below the line of code
b1.addActionListener(this);
add an action to the new button by writing this statement:
b2.addActionListener(e -> System.exit(0));
Test your modified program and the operation of the [ EXIT ] button.
STEP 5 Modify Again the Program You will now expand your programs layout by including a new row of GUI elements.
To accomplish this, perform these tasks:
In the class definition and before the constructor method, declare a new JLabel named l1 and also declare a new JCheckBox element.
JCheckBox check1;
In the class constructor, instantiate these two new GUI elements as follows:
l4 = new JLabel(" "); check1 = new JCheckBox("click to select"); In the class constructor, set the initial state of the textbox.
check1.setSelected(true); PROJECT Simple Java GUI: Salary Calculator
In the class constructor, place the new checkbox and label to appear between text field 3 and button 1 .
add(check1); add(l4);
Now change the settings of the GridLayout() to accommodate five rows and two columns instead of the existing four rows and two columns.
Finally, add a skeletal if / else block directly below the existing if block that appears in the actionPerformed(ActionEvent ae) method. // verify the checkbox state if (check1.isSelected()) { // perform a task ... } else { // perform a different task ... }
Test your modified program and the operation of the program.
STEP 6 Alter the Program
Now change the GUI application such that the screen will appear as follows:
PROJECT Simple Java GUI: Salary Calculator
Then alter your program code such that when a current salary and percent rate is entered into the respective text fields a new salary is then computed, with a bonus amount added to the new salary if the checkbox is selected. You can test your program with these two sample data scenarios:
Scenario I ( bonus pay NOT included )
Current Salary $ 30,000 Percent Rate 5 %
Bonus Pay NO
New Salary $ 31,500 Scenario II ( bonus pay included )
Current Salary $ 30,000 Percent Rate 5 %
Bonus Pay YES ( $ 500 )
New Salary $ 32,000 STEP 7 Supplement the Graphical User Interface
Finally, supplement the GUI interface such that it would appear such as the following.
To accomplish this, follow these steps:
Include a new JLabel named l5 .
Include a new JTextField named t4 .
Instantiate the new JLabel component.
l5 = new JLabel(" Difference");
PROJECT Simple Java GUI: Salary Calculator
Instantiate the new JTextField component. t4 = new JTextField(10);
Add the JLabel component to the interface. add(l5);
Add the JTextField component to the interface. add(t4);
Remember to adjust the grid layout to adjust for the new components that were added to the interface.
Modify now the program code statements associated with the [ Compute ] button such that the difference of the Current Salary and the New Salary is displayed in the interface, as shown below.
STEP 8 Submit Your Project
Once you have determined that your modified program is correctly displaying the required information, complete the submission process as follows:
Open MS Word and type a heading for a new document that includes your full name, course number, lab number and date.
Within the document, paste a sufficient number of screen snapshots of your modified program in action. Multiple snapshots will be required to show the program is fully functional for different input values. Label the snapshots of your modified run with a reasonable description.
After your snapshot, paste in your finished source code as well copied in from your Java editor.
Submit your MS Word document to Blackboard when complete.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
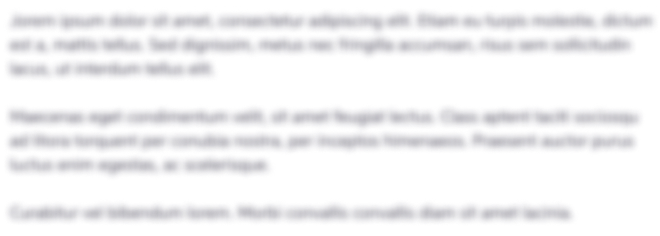
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started