Question
Project Specification: Simulation of a Small Airport Simulation of a Small Airport Simulation is the use of one system to imitate the behavior of another
Project Specification: Simulation of a Small Airport
Simulation of a Small Airport Simulation is the use of one system to imitate the behavior of another system. Computer simulations use the steps of a program to imitate the behavior of a system under study.
In a computer simulation, the objects being studied are usually represented as data, often as classes whose methods describe behavior of the objects and whose instance variables describe the properties of the objects. Most importantly, actions being studied are represented as methods of the classes, and the rules describing these actions are translated into computer algorithms. By changing the values of the data or by modifying these algorithms we can observe changes in the computer simulation, then we can draw worthwhile conclusions concerning the behavior of the actual system.
This project is a simulation of an airport. As a specific example, we consider a small but a busy airport with only one runway. The simulation will run for a certain number of units of time. In each time unit, one plane can land or one plane can take off, but not both. Planes arrive ready to land or take off at random times, so at any given moment of time, the runway may be idle or a plane may be landing or taking off, and there may be several planes waiting either to land or take off. Since there is only a single runway, we will not use a separate ATC (air traffic control) class which might normally register arriving and departing flights for a large airport with multiple terminals and runways. The runway class controls all air traffic in this project.
Throughout the simulation we keep track of statistics so that we can have a good understanding of how efficiently the runway is used. In this simulation we need the following three classes:
class Plane
class Runway
a class to represent the AirportSimulation
3 class AirportSimulation
We begin with the simulation class that has the static main method. We need to think what statistics we are going to keep and what input data we need. We need to process the requests of arriving planes, and departing planes. For this we need to know at what rates the planes are arriving.
Only one plane can be served in any unit of time. So either a plane can land or a plane can takeoff not both.
Since waiting in the air might lead to disasters, arrivals have higher priority than the departing planes.
Only when the landing list is empty, is a departing plane allowed to takeoff.
Planes cant be queued for too long while waiting to land. This means there is a capacity limit on the landing list. When this list is full arriving planes must be directed to another airport.
The simulation of the airport should keep track of how long a landing or departing plane waits in the line before landing or departing.
It should also keep track of how many arriving flights are directed to another airport.
The simulation should keep track of the number of planes that requested landing and the number that are accepted, and the number that are landed.
The departing list doesnt have to have a limit. All requests for departures will be accepted and served in the order in which they requested the service.
At the end of simulation, you need to find the total number of planes served, the average rate of planes wanting to land per unit time, the average rate of planes wanting to depart per unit time, the average wait in the landing line, and the average wait in the departing line in time units.
3.1 Input Data required from a user of the simulation This will be read as input in the main method before the simulation begins.
1. endTime in time units.
2. landing list size. Keep this size small.
3. arrivalRate, departureRate. These two variables are doubles. The sum of these two rates shouldnt exceed 1.0 or this will saturate the runway. You must validate this to make sure that the values you entered are acceptable.
3.2 Methods
1. The simulation class needs the main method and other required methods.
2. The simulation has one principal loop that begins at time 0, and ends at time endTime. Divide this time into 1 unit intervals. You plan for at least 1000 time intervals.
(a) Generate the number of arriving planes in each interval. I have typed a method called poisson(double rate) which gives you the number of arriving planes. (Note: there might be more than one plane that arrives in any interval. The Poisson distribution is a statistical distribution that simulates this possibility. The given method uses the Poisson distribution, but you should simply view it as returning a number here the number of arriving planes.) Use a loop to add the arriving planes to ArrayList landing as long as landing hasnt reached its limit. Adjust relevant statistics about arriving planes.
(b) Excess arriving planes should be refused and directed to a different airport and statistics should be kept.
(c) Also get a number of departing planes from the method poisson(double rate) using departingRate as the parameter. Add the departing planes to the ArrayList takeoff in each unit time interval. Once again dont forget to keep statistics.
(d) Next check if there is a plane waiting to land, if so allow it to land and update the stats.
(e) If there is no plane to land, check if there is any plane to takeoff and allow it to takeoff and keep stats.
(f) Otherwise leave the runway idle in that time interval and keep track of the number of idle minutes by incrementing the variable idleTime. At the end of simulation, you are going to print the % of time the runway stayed idle.
(g) That ends the one iteration of the time loop.
(h) After all the iterations, you have to call the shutdown method of the Runway class and print out all the statistics that you have collected and close the input Scanner object.
(i) This ends the main method.
3. In addition to the main method you need two additional methods.
4. validate(double arrivalRate, double departureRate)
5. poisson(double rate) which will give the number of planes arriving and the number that want to depart.
6. This should end the Simulation class. Here is code you should use for the Poisson distribution method:
public static int poisson(double rate) {
double limit = Math.exp(-rate);
double product = Math.random();
double count = 0; while (product > limit) {
count++; product *= Math.random();
}
return count;
}
4 class Runway
4.1 Instance variables
ArrayList landing;
ArrayList takeoff;
int listLimit; //The limit of the landing list.
4.2 Methods
1. Runway(int limit) //Constructor with one parameter which represents size of the landing list
2. boolean canLand(Plane current) // returns true if it can enter the landing list otherwise false.
3. void addPlane(Plane current) //Add the current plane to the landing list
4. void addTakeoff(plane current)// Add the current plane to the takeoff list;
5. void activityLand(int time) //let the first plane in the list land and then remove it after the plane lands.
6. void activityTakeoff(int time)// Let the first plane in the takeoff list takeoff.
7. void runIdle(int time) //If there is no plane to land or takeoff, let the runway idle.
5 class Plane
5.1 Instance variables
1. int flightNumber //
2. int clockStart //Time the plane enters the Runway system
3. int status (null = 0, arriving = 1, departing = 2)
5.2 Methods
1. Plane() (Default Constructor)
2. Plane(int fltNumber, int time, int status) (Constructor)
3. void refuse() //If the landing ArrayList reaches a predetermined size the plane gets directed to a different airport.
4. void land(int time) //Arriving flight lands and once it lands it will be removed from the list.
5. void fly(int time) //Departing flight takes off, and it is deleted from the takeoff list.
6. int started() //returns the time at which a plane joined the appropriate Runway ArrayList.
7. The Plane methods output what is happening. For example, the method refuse() checks the status of the plane, if it is arriving, then it checks that ArrayList landing hasnt reached the preset limit. If it has, it simply outputs that the plane is redirected.
8. Think about what messages landing and departing planes should output and print that info.
9. Both arriving and departing planes must find how long they had to wait in line before landing or departing and print that information.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
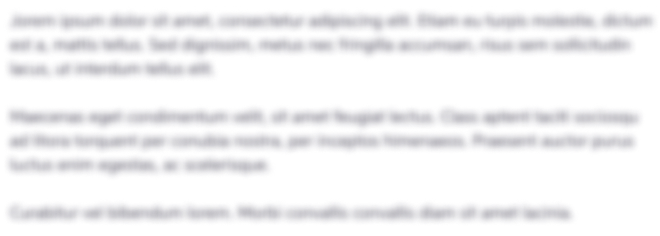
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started