Question
Provided code(solve under multiplyTerm method): package assignments2018.a2template; import java.math.BigInteger; public class Polynomial { private SLinkedList polynomial; public int size() { return polynomial.size(); } private Polynomial(SLinkedList
Provided code(solve under multiplyTerm method):
package assignments2018.a2template;
import java.math.BigInteger;
public class Polynomial { private SLinkedList
/**** ADD CODE HERE ****/ return null; } //TODO: multiply this polynomial by a given term. private void multiplyTerm(Term t) { /**** ADD CODE HERE ****/ } //TODO: multiply two polynomials public static Polynomial multiply(Polynomial p1, Polynomial p2) { /**** ADD CODE HERE ****/ return null; } //TODO: evaluate this polynomial. // Hint: The time complexity of eval() must be order O(m), // where m is the largest degree of the polynomial. Notice // that the function SLinkedList.get(index) method is O(m), // so if your eval() method were to call the get(index) // method m times then your eval method would be O(m^2). // Instead, use a Java enhanced for loop to iterate through // the terms of an SLinkedList.
public BigInteger eval(BigInteger x) { /**** ADD CODE HERE ****/ return new BigInteger("0"); } // Checks if this polynomial is same as the polynomial in the argument public boolean checkEqual(Polynomial p) { if (polynomial == null || p.polynomial == null || size() != p.size()) return false; int index = 0; for (Term term0 : polynomial) { Term term1 = p.getTerm(index); if (term0.getExponent() != term1.getExponent() || term0.getCoefficient().compareTo(term1.getCoefficient()) != 0 || term1 == term0) return false; index++; } return true; } // This method blindly adds a term to the end of LinkedList polynomial. // Avoid using this method in your implementation as it is only used for testing. public void addTermLast(Term t) { polynomial.addLast(t); } // This is used for testing multiplyTerm public void multiplyTermTest(Term t) { multiplyTerm(t); } @Override public String toString() { if (polynomial.size() == 0) return "0"; return polynomial.toString(); } }
5. Polynomial.multiplyTerm (15 points) This is a private helper method used by the multiply function. The polynomial object multiplies each of its terms by an argument term and updates itself. The runtime of this method should be O(n)Step by Step Solution
There are 3 Steps involved in it
Step: 1
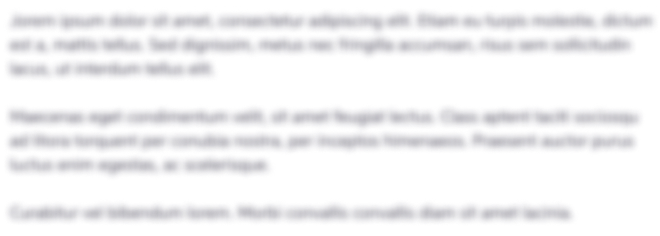
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started