Question
public abstract class Creature { public abstract int getIntLevel(); } public class HumanBeing{ private int intLevel; private String name; private int age; public HumanBeing(int intLevel,
public abstract class Creature { public abstract int getIntLevel(); }
public class HumanBeing{ private int intLevel; private String name; private int age;
public HumanBeing(int intLevel, String name, int age) { this.intLevel = intLevel; this.name = name; this.age = age; }
public void setIntLevel(int intLevel) { this.intLevel = intLevel; }
public int getIntLevel() { return intLevel; }
public String getName() { return name; }
public int getAge() { return age; } }
public class Male extends HumanBeing {
private int height; private int weight;
public Male(String name, int age, int height, int weight, int intLevel) throws notHumanBeingException { super(intLevel, name, age); this.height = height; this.weight = weight; }
public int getHeight() { return height; }
public int getWeight() { return weight; }
public void calcIntLevel() { if (this.getAge() > 40) { this.setIntLevel(5); } else if (this.getAge() < 5) { this.setIntLevel(1); } else if (this.weight >= 150 && this.height > 70) { this.setIntLevel(5); } else if (this.weight < 149 && this.weight > 120 && this.height > 70) { this.setIntLevel(4); } else if (this.weight < 119 && this.weight > 50) { this.setIntLevel(3); } else if (this.weight < 149 && this.weight > 120 && this.height < 69 && this.height > 55) { this.setIntLevel(2); } else { this.setIntLevel(3); } } }
public class Female extends HumanBeing {
private String lastDegree;
public Female( String name, int age, String lastDegree, int intLevel) throws notHumanBeingException{ super(intLevel, name, age); this.lastDegree = lastDegree; }
public String getLastDegree() { return lastDegree; }
public void calcIntLevel() { if (this.getLastDegree() == "Ph.D.") { this.setIntLevel(5); } else if (this.getLastDegree() == "MS") { this.setIntLevel(4); } else if (this.getLastDegree() == "BS") { this.setIntLevel(3); } else if (this.getLastDegree() == "AA") { this.setIntLevel(2); } else { this.setIntLevel(1); } }
}
public class notHumanBeingException extends Exception{ public notHumanBeingException(){ } public notHumanBeingException(String message){ super(message); } }
import java.io.FileNotFoundException; import java.io.FileReader; import java.io.PrintWriter; import java.util.Scanner; import javax.swing.JOptionPane; import java.util.ArrayList;
public class CreatureTester {
public static void main(String[] args) throws notHumanBeingException, FileNotFoundException {
String inf = JOptionPane.showInputDialog("Input file?"); // Input file is src/
ArrayList
while (in.hasNextLine()) { String line = in.nextLine(); String[] split = line.split("\\s+");
switch (split[0].toUpperCase().charAt(0)) { case 'M': { try { Male male = new Male(split[1], Integer.parseInt(split[2]), Integer.parseInt(split[3]), Integer.parseInt(split[4]), Integer.parseInt(split[5])); if (male.getWeight() <= 400 && male.getHeight() <= 100) { list.add(male); System.out.println(male); } else ; } catch (notHumanBeingException e) { System.out.println("This is not a valid Male"); } break; } case 'F': { try { Female female = new Female(split[1], Integer.parseInt(split[2]), split[3], Integer.parseInt(split[4])); if (female.getAge() < 110) { list.add(female); } else ; } catch (notHumanBeingException e) { System.out.println("This is not a valid female"); } break; } }
outFile.println(line + " ");
}
outFile.close (); } }
There are many different types of Creatures, and talking about the intelligence level of a Creature makes perfect sense. HumanBeing is a Creature, each possessing, in addition to the intelligence level, a name and age. Male is a HumanBeing, and so is Female. Animal is another Creature which possesses some Intelligence Level, and so is Bird.
In this assignment, you are required to implement a Creature as an abstract class containing an abstract method getIntLevel; a HumanBeing class described as above; a Male class and a Female class. For each male, we wish to keep track of his height and weight, whereas for every female, we wish to keep track of her last degree. Male and Female classes have all required accessor methods to return the values of their attributes. Both, Male and Female classes allow users to calculate intelligence level defined (absurdly) as follows:
Male: (Calculation proceeds in the order given here)
If age > 40, then IntLevel = 5
If age is < 5 years, then IntLevel = 1
If weight >= 150 pounds and height is > 70 inches then IntLevel = 5
If weight is between 120 and 149 pounds, and height > 70 inches, then IntLevel = 4
If weight is between 50 and 119 pounds, then IntLevel = 3
If weight is between 120 and 149 pounds, and height is between 55 and 69 inches,
then IntLevel = 2
In all other cases, the IntLevel = 3.
Female:
If lastDegree = "Ph.D.", then IntLevel = 5
If lastDegree = "MS", then IntLevel = 4
If last Degree = "BS", then IntLevel = 3
If last Degree = "AA", then IntLevel = 2
In all other cases, IntLevel = 1.
When a Male or Female object is created, the constructor checks if this is indeed a "valid" person. A "valid" male is someone whose weight is less than 400 pounds and height is less than 100 inches. A "valid" female is someone whose age is less than 110 years. If the user tries to create an "invalid" person, the class constructors throw an exception called notHumanBeingException to the calling program. The calling program rejects that person from consideration. In case the person was "valid", the constructor calculates the person's Intlevel and initializes it. Obviously, HumanBeing must provide a method that allows setting of one's IntLevel.
The test program will do the following:
From an input file (as always, get the name of the file from the user), read the basic information about Human Beings. The first character identifies what type of Human Being this person is - M or F. Information in the input file is in the following order:
Male: name, age, height (in inches), weight (in pounds).
Female: name, age, last degree.
Create an ArrayList of all persons, an array of males, and an array of females from this given data. That is, each person will be included in an ArrayList and one array.
Output unsorted ArrayList of persons.
Sort the ArrayList based on names, using "sort" method of the Collections interface.
Output the sorted ArrayList of persons.
Output unsorted list of Males.
Sort the array of males based on their heights using Arrays.sort (array_of_males, comparator) call.
Output the sorted array of males.
Output the unsorted list of females.
Sort this array based on age using Arrays.sort (array_of_females) call. Note that no comparator is passed in this call. Do whatever you need to do to achieve this.
Output the sorted list of females.
The output should be sent to an output file
My code is above but ive gotten stuck on the part that checks if the male or female is valid.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
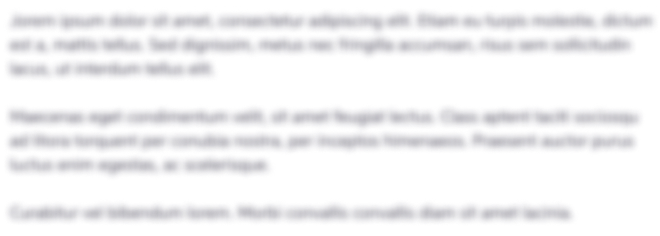
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started