Question
public class ArrayBoundedStack implements StackInterface { protected final int DEFCAP = 100; // default capacity protected T[] elements; // holds stack elements protected int topIndex
public class ArrayBoundedStack
public ArrayBoundedStack() { elements = (T[]) new Object[DEFCAP]; }
public ArrayBoundedStack(int maxSize) { elements = (T[]) new Object[maxSize]; }
public void push(T element) // Throws StackOverflowException if this stack is full, // otherwise places element at the top of this stack. { if (isFull()) throw new StackOverflowException("Push attempted on a full stack."); else { topIndex++; elements[topIndex] = element; } }
public void pop() // Throws StackUnderflowException if this stack is empty, // otherwise removes top element from this stack. { if (isEmpty()) throw new StackUnderflowException("Pop attempted on an empty stack."); else { elements[topIndex] = null; topIndex--; } }
public T top() // Throws StackUnderflowException if this stack is empty, // otherwise returns top element of this stack. { T topOfStack = null; if (isEmpty()) throw new StackUnderflowException("Top attempted on an empty stack."); else topOfStack = elements[topIndex]; return topOfStack; }
public boolean isEmpty() // Returns true if this stack is empty, otherwise returns false. { return (topIndex == -1); }
public boolean isFull() // Returns true if this stack is full, otherwise returns false. { return (topIndex == (elements.length - 1)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
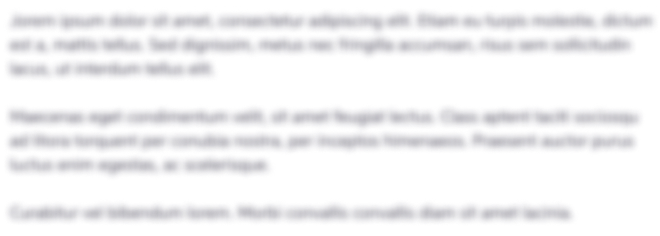
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started