Question
public class ArrayProblems { /* Given an array of integers nums, sort the array in ascending order. Example: Input: nums = [5,2,3,1] Output: [1,2,3,5] Example:
public class ArrayProblems {
/* Given an array of integers nums, sort the array in ascending order.
Example: Input: nums = [5,2,3,1] Output: [1,2,3,5] Example: Input: nums = [5,1,1,2,0,0] Output: [0,0,1,1,2,5] */ public static int[] sortArray(int[] nums) { //TODO:finish this method. //TODO: Modify this line to return correct data. return null;
}
/* * Find the kth largest element in an unsorted array. * Note that it is the kth largest element in the sorted order, not the kth distinct element. Example1: Input: [3,2,1,5,6,4] and k = 2 Output: 5 Example2: Input: [3,2,3,1,2,4,5,5,6] and k = 4 Output: 4 */ public static int findKthLargest(int[] nums, int k) { //TODO:finish this method. //TODO: Modify this line to return correct data. return 0; } }
-------------------------------------------------------------------------------------------
class ArrayProblemsTest {
@BeforeEach void setUp() throws Exception { }
/* sortArray CASE 1: Input: nums = [5,2,3,1] Output: [1,2,3,5] */ @Test void testSortArray1() { int[] nums= {5,4,3,1}; int[] expected = {1,3,4,5}; assertArrayEquals(expected, ArrayProblems.sortArray(nums)); }
/* sortArray CASE 2: Input: nums = [5,1,1,2,0,0] Output: [0,0,1,1,2,5] */ @Test void testSortArray2() { fail("Not yet implemented"); // TODO }
/* findKthLargest test case1: Input: [3,2,1,5,6,4] and k = 2 Output: 5 */ @Test void testFindKthLargest1() { fail("Not yet implemented"); // TODO }
/* findKthLargest test case1: Input: [3,2,3,1,2,4,5,5,6] and k = 4 Output: 4 */ @Test void testFindKthLargest2() { fail("Not yet implemented"); // TODO }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
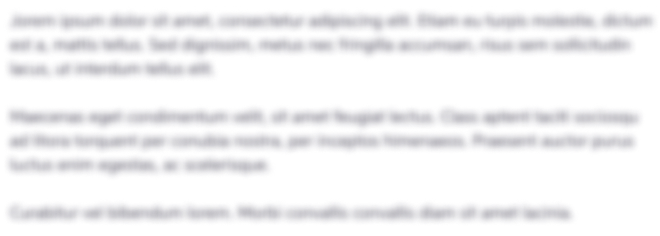
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started