Question
public class driver { public static void main(String[] args) { ////////////////////////////////////////////////// //Q0: Make sure that you are able to run the driver class and see
public class driver {
public static void main(String[] args) {
//////////////////////////////////////////////////
//Q0: Make sure that you are able to run the driver class and see the Vending Machine in action
VendingMachine vm = new VendingMachine(5, 1, "Water");
// Enter the sufficient change
vm.Vend();
// enter insufficient change
vm.Vend();
// Q1: Make this VM vend Pepsi for you by changing above code
//////////////////////////////////////////////////
//Q6: test out your canadian vending machine by editing the code above!
}
}
******************************************************************************************************************* ////////////////////////////////////
//Write a class that extends the Vending Machine class to a vending machine that only vends Pepsi
public class pepsi_VendingMachine extends VendingMachine {
// Q:1 how would tweak the parent's constructor so that it can only vend pepsi? fill it in below
private String product;
public pepsi_VendingMachine( , )
{
}
//Q2: write the getters and setters using the parent class' getters and setters.
// Hint: use "super" key word to get the parent class' constructors
// Hint: In eclipse, type "get" and then press ctr+tab ;)
// see if that works for "set" :P
// Q3:Complete the following class method to print how many bottles this vending machine would have
public int count_bottles(){
int count = 0;
// write your code here
// hint: look at status method
return count;
}
// Q4: Write a method to clear all the VendingMachine money at the end of the day.
}
************************************************************************************************************
public class Canadian_VendingMachine {
// This class is an extension of VendingMachines in Canada, in a US embassy.
// Q5: Now, the original Vending Machine was made in US and all the currency was set to USD.
// But in Canada, we need to convert USD to CAD.
// %(a)One USD = 1.31 CAD, with conversion rate. write a getter and setter for it
private double conv;
public double getConv(){
// code here
}
public void setConv(double rate){
}
// Here is what should happen:
//1. Price is displayed in USD and CAD both
//2. Customer gets a choice which currency to pay in
//3. Customer gets the change money in the currency he chose for
//4. The vending machine should always store the money in USD
// For this to happen, first ask customer which CurrencyIn they would like to pay.
// 5(c) Hence, override the currencyIn method
public String currencyIn(){
//5(b)) this requires some getters! Write them first!
// correct the following line
System.out.println("The price of a "+type+" is "+price+" USD");
System.out.println("which is "+getConv+ "CAD");
System.out.println("How would you like to pay? USD or CAD? Please enter your response");
String curr = "USD";
// ask user for the response using scanner
// Store it in curr
return curr;
}
// Now, you will have to override the pay() method from parent
public double pay() {
String curr = this.currencyIn();
// 5(d)getMoneyFromCustomer() is a super class method. So edit the following line to call that
double customerMoney = ?.getMoneyFromCustomer();
// get the price per bottle in the given country's currency.
// 5(e) edit the following line with getPrice, and exchange rate, and call exchangePrice from parent
double unitprice = ?.exchangePrice(curr, ?, this.conv);
// 5(f) getChangeis a super class method. So edit the following line to call that
double change = ?.getChange(unitprice, customerMoney);
// if customer added insufficient money, the change would be the amount customer paid to, and in that case we don't want to wend anything
if (change - customerMoney != 0.0){
// yes, customer did give sufficient money
//5(g)the price here should be in USD
?.addVendingMachineMoney(?.price);
return change;
}
return -1;
}
} ***********************************************************************************************
import java.util.Arrays; import java.util.*;
public class VendingMachine { /////////////////////////////////////////////////////////////////////////// private String type; private String [] stuff; private double machineMoney; private double price; private int capacity = 0; /////////////////////////////////////////////////////////////////////////// // getters public int getCapacity() { return capacity; } public double getMachineMoney() { return machineMoney; } public double getPrice() { return price; } public String[] getStuff() { return stuff; } public String getType() { return type; } /////////////////////////////////////////////////////////////////////////// //setters protected void setCapacity(int capacity) { this.capacity = capacity; } protected void setMachineMoney(double machineMoney) { this.machineMoney = machineMoney; } protected void setPrice(double price) { this.price = price; } public void setStuff(String[] stuff) { this.stuff = stuff; } public void setType(String type) { this.type = type; } /////////////////////////////////////////////////////////////////////////// public VendingMachine(int x, double p, String type) { this.type = type; stuff = new String [x]; Arrays.fill(stuff,this.type); price = p; }
/////////////////////////////////////////////////////////////////////// public int status() { int stuff_at = -1; for (int i = 0; i < stuff.length; i++) { if(stuff[i]!=null) { stuff_at = i; } } return stuff_at; }
/// The following methods are methods to help a machine transaction public String currencyIn(){ System.out.println("The price of a "+type+" is "+price+" USD"); String curr = "USD"; return curr; } public double getMoneyFromCustomer(){ Scanner s = new Scanner(System.in); System.out.println("Please enter your money"); double customerMoney = s.nextDouble(); return customerMoney; } public double getChange(double unitprice, double customerMoney){ double change = customerMoney; if(customerMoney public void Vend() { String product = null; int at = this.status(); /////////////////////////////////////////////////////////////////////// if (at>=0) { double change = this.pay(); if (change >=0) { System.out.println("Your change is "+change); product = stuff[at]; stuff[at] = null; System.out.println(" Here is your one "+product); System.out.println("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~"); System.out.println("Have a great day!"); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
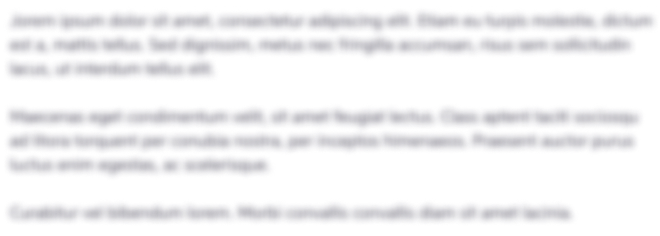
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started