Answered step by step
Verified Expert Solution
Question
1 Approved Answer
public class DVD implements Comparable { private String title; private int year; private int duration; public DVD () { this (,0,0); } public DVD (String
public class DVD implements Comparable { private String title; private int year; private int duration; public DVD () { this ("",0,0); } public DVD (String newTitle, int y, int minutes) { title = newTitle; year = y; duration = minutes; } public int compareTo(Object obj) { if (obj instanceof DVD) { DVD aDVD = (DVD)obj; return title.compareTo(aDVD.title); } return 0; } public String getTitle() { return title; } public int getDuration() { return duration; } public int getYear() { return year; } public void setTitle(String t) { title = t; } public void setDuration(int d) { duration = d; } public void setYear(int y) { year = y; } public String toString() { return ("DVD (" + year + "): \"" + title + "\" with length: " + duration + " minutes"); } public static void main(String args[]) { System.out.println(DVD.parseFrom("Rush Hour 2,2001,118")); } }
import javafx.scene.control.Button; import javafx.scene.layout.Pane; public class DVDButtonPane extends Pane { private Button addButton, deleteButton, statsButton; public Button getAddButton() { return addButton; } public Button getDeleteButton() { return deleteButton; } public Button getStatsButton() { return statsButton; } public DVDButtonPane() { Pane innerPane = new Pane(); // Create the buttons addButton = new Button("Add"); addButton.setStyle("-fx-font: 12 arial; -fx-base: rgb(0,100,0); -fx-text-fill: rgb(255,255,255);"); addButton.relocate(0, 0); addButton.setPrefSize(90,30); deleteButton = new Button("Delete"); deleteButton.setStyle("-fx-font: 12 arial; -fx-base: rgb(200,0,0); -fx-text-fill: rgb(255,255,255);"); deleteButton.relocate(95, 0); deleteButton.setPrefSize(90,30); statsButton = new Button("Stats"); statsButton.setStyle("-fx-font: 12 arial;"); statsButton.relocate(210, 0); statsButton.setPrefSize(90,30); // Add all three buttons to the pane innerPane.getChildren().addAll(addButton, deleteButton, statsButton); getChildren().addAll(innerPane);//, titleLabel); } }
public class DVDCollection { public static final int MAX_DVDS = 100; private DVD[] dvds; private int dvdCount; public DVDCollection() { dvds = new DVD[MAX_DVDS]; } public DVD[] getDvds() { return dvds; } public DVD[] getDVDList() { DVD[] list = new DVD[dvdCount]; for (int i=0; i
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp1 extends Application { private DVDCollection model; public DVDCollectionApp1() { model = DVDCollection.example1(); } public void start(Stage primaryStage) { Pane aPane = new Pane(); // Create the view DVDCollectionAppView1 view = new DVDCollectionAppView1(); aPane.getChildren().add(view); primaryStage.setTitle("My DVD Collection"); primaryStage.setResizable(false); primaryStage.setScene(new Scene(aPane)); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.layout.Pane; public class DVDCollectionAppView1 extends Pane { private ListViewtList; private ListView yList, lList; private DVDButtonPane buttonPane; public ListView getTitleList() { return tList; } public ListView getYearList() { return yList; } public ListView getLengthList() { return lList; } public DVDButtonPane getButtonPane() { return buttonPane; } public DVDCollectionAppView1() { // Create the labels Label label1 = new Label("Title"); label1.relocate(10, 10); Label label2 = new Label("Year"); label2.relocate(220, 10); Label label3 = new Label("Length"); label3.relocate(290, 10); // Create the lists tList = new ListView (); tList.relocate(10, 40); tList.setPrefSize(200,150); yList = new ListView (); yList.relocate(220, 40); yList.setPrefSize(60,150); lList = new ListView (); lList.relocate(290, 40); lList.setPrefSize(60,150); // Create the button pane buttonPane = new DVDButtonPane(); buttonPane.relocate(30, 200); buttonPane.setPrefSize(305,30); // Add all the components to the Pane getChildren().addAll(label1, label2, label3, tList, yList, lList, buttonPane); setPrefSize(348, 228); } }
import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.control.TextField; import javafx.scene.layout.Pane; public class DVDCollectionAppView2 extends Pane { private ListViewtList; private TextField tField, yField, lField; private DVDButtonPane buttonPane; public ListView getTitleList() { return tList; } public TextField getTitleField() { return tField; } public TextField getYearField() { return yField; } public TextField getLengthField() { return lField; } public DVDButtonPane getButtonPane() { return buttonPane; } public DVDCollectionAppView2() { // Create the labels Label label1 = new Label("DVDs"); label1.relocate(10, 10); Label label2 = new Label("Title"); label2.relocate(10, 202); Label label3 = new Label("Year"); label3.relocate(10, 242); Label label4 = new Label("Length"); label4.relocate(120, 242); // Create the TextFields tField = new TextField(); tField.relocate(50, 200); tField.setPrefSize(500,30); yField = new TextField(); yField.relocate(50, 240); yField.setPrefSize(55,30); lField = new TextField(); lField.relocate(180, 240); lField.setPrefSize(45,30); // Create the lists tList = new ListView (); tList.relocate(10, 40); tList.setPrefSize(540,150); // Create the buttons buttonPane = new DVDButtonPane(); buttonPane.relocate(250, 240); buttonPane.setPrefSize(305,30); // Add all the components to the Pane getChildren().addAll(label1, label2, label3, label4, tField, yField, lField, tList, buttonPane); setPrefSize(548, 268); } }
import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.layout.Pane; public class DVDCollectionAppView3 extends Pane { private ListViewThe tutorial code contains two different Pane classes that represent three different views of a DVDCollection These classes are called DVDCollectionAppView1 and DVDCollectionAppView2 DVDs Title Length Title Add Delete Stats Year Length Add Stats DVDCollectionAppView1 DVDCollectionAppView2 There is also an application called DVDCollectionApp1 which is used as the controller for the application. That is, it represents the main application onto which the view is attached A. Run the DVDCollectionApp1 class and ensure that the window contains the first view above B. Change the line that creates the view to create a DVDCollectionAppView2 view instead of a DVDCollectionAppView1 view C. Re-run the code and ensure that it looks like the 2nd view above Note that we will keep changing which view we have in the application throughout the tutorial D. Rewrite the line so that it creates a DVDCollectionAppView1 view again. Notice how we can simply swap various views in and out of our application with this simple one- line change. This is one advantage of separating the view from the controller in our MVC strategy Z) Now we will insert the model into the mix as our third part of the MVC. The model is a DVDCollection objectdList; private DVDButtonPane buttonPane; public ListView getDVDList() { return dList; } public DVDButtonPane getButtonPane() { return buttonPane; } public DVDCollectionAppView3() { // Create the labels Label label1 = new Label("Title, Year, Length (min.)"); label1.relocate(10, 10); // Create the lists dList = new ListView (); dList.relocate(10, 40); dList.setPrefSize(300,250); // Create the buttons buttonPane = new DVDButtonPane(); buttonPane.relocate(10, 300); buttonPane.setPrefSize(305,30); // Add all the components to the Pane getChildren().addAll(label1, dList, buttonPane); setPrefSize(310, 330); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
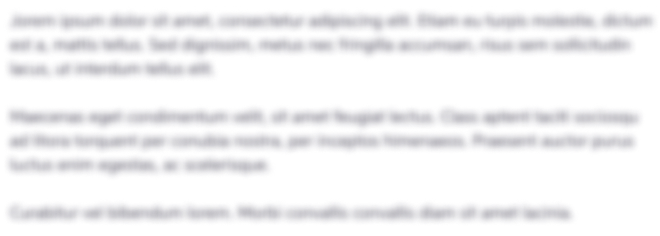
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started