Question
////////////////////////////////////////////////////////////// public class EPProfile { /* Attributes */ private String First; private String Last; private int dob; // YYYYMMDD private int medConditions; private int firstDose;
////////////////////////////////////////////////////////////// public class EPProfile {
/* Attributes */ private String First; private String Last; private int dob; // YYYYMMDD private int medConditions; private int firstDose; private int secondDose; private boolean secondAdministered; /* Constructors */ public EPProfile() {} public EPProfile(String First, String Last, int dob, int medConditions, int firstDose, int secondDose, boolean secondAdministered) { this.First = First; this.Last = Last; this.dob = dob; this.medConditions = medConditions; this.firstDose = firstDose; this.secondDose = secondDose; this.secondAdministered = secondAdministered; } public EPProfile(EPProfile P) { this.First = P.getFirst(); this.Last = P.getLast(); this.dob = P.getDob(); this.medConditions = P.getMedConditions(); this.firstDose = P.getFirstDose(); this.secondDose = P.getSecondDose(); this.secondAdministered = P.getSecondAdministered(); }
/* Getters and Setters */ /** * @return the first */ public String getFirst() { return First; }
/** * @param first the first to set */ public void setFirst(String first) { First = first; }
/** * @return the last */ public String getLast() { return Last; }
/** * @param last the last to set */ public void setLast(String last) { Last = last; }
/** * @return the dob */ public int getDob() { return dob; }
/** * @param dob the dob to set */ public void setDob(int dob) { this.dob = dob; }
/** * @return the medConditions */ public int getMedConditions() { return medConditions; }
/** * @param medConditions the medConditions to set */ public void setMedConditions(int medConditions) { this.medConditions = medConditions; }
/** * @return the firstDose */ public int getFirstDose() { return firstDose; }
/** * @param firstDose the firstDose to set */ public void setFirstDose(int firstDose) { this.firstDose = firstDose; }
/** * @return the secondDose */ public int getSecondDose() { return secondDose; }
/** * @param secondDose the secondDose to set */ public void setSecondDose(int secondDose) { this.secondDose = secondDose; }
/** * @return the secondAdministered */ public boolean getSecondAdministered() { return secondAdministered; }
/** * @param secondAdministered the secondAdministered to set */ public void setSecondAdministered(boolean secondAdministered) { this.secondAdministered = secondAdministered; } /* Other methods */ public String toString() { String output = Last + "' " + First + " "; output += "Date of birth: " + dob + " "; output += "Number of medical conditions: " + medConditions + " "; output += "Date of first dose: " + firstDose + " "; if (secondAdministered) output += "Date of second dose: " + secondDose + " "; else output += "Second dose pending, to be administered: " + secondDose + " "; return output; } public void print() { System.out.println(toString()); } }
///////////////////////////////////////////////////////////////////////////
import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; public class EPProfileDB { /* Attributes **********************************************************/ private EPProfile[] ProfileDB; private int size; /* Constructors ********************************************************/ public EPProfileDB() {} public EPProfileDB(int size) { this.size = size; ProfileDB = new EPProfile[size]; } public EPProfileDB(EPProfile[] DB) { size = DB.length; ProfileDB = new EPProfile[size]; for (int i=0; i ProfileDB[i] = DB[i]; } } /* TO DO 1: * Write a constructor that takes a file name (String) as only parameter * and reads the file information into an instance of an EPProfile with correct size and array of profiles. * The size if the first information read in the file (first line). * The EPProfile objects that go in the the ProfileDB are read from the file, one by one as follows: * Last-name First-name * DOB * Number of medical conditions * Date of first dose (YYYYMMDD) * Date of second dose (YYYYMMDD) * Second administered (as yeso) * We give you starter code for this method, as available below (you have to complete it). */ public EPProfileDB(String filename) throws FileNotFoundException, IOException { FileReader fr = new FileReader(filename); BufferedReader textReader = new BufferedReader(fr); /* We read the size of the DB as an integer on the first line of the file */ if (textReader.ready()) { size = Integer.valueOf(textReader.readLine()); ProfileDB = new EPProfile[size]; } else{ textReader.close(); } for(int i=0; i /* TO DO 2: * This method prints all profiles in the ProfileDB, one by one */ public void print() { // COMPLETE CODE HERE } /* TO DO 3: * This method moves all profiles of already twice vaccinated people * at the end of the DB. * It returns the number of people who have already been vaccinated twice. */ public int moveDoneToEnd() { // COMPLETE CODE HERE return 0; // dummy return, just to ensure that code compiles: please modify }
/////////////////////////////////////////////////////////////////////////////////////////////////
import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.io.PrintWriter; import java.util.Random; public class EPVaccination { /* You will now use the methods you designed to handle patient profiles for vaccination */ public static void main(String[] args) throws FileNotFoundException, IOException { String filename = "/Users/username/myDB.txt"; // please change this path to where your file is EPProfileDB EPDB1 = new EPProfileDB(filename); EPDB1.print(); EPDB1.moveDoneToEnd(); EPDB1.print(); double[] stats = EPDB1.compareAgeMCRankings(); System.out.println("max Change = " + stats[0] + "; " + "min Change = " + stats[1] + "; " + "average Change = " + stats[2] + "."); } }
//////////////////////////////////////////////////////////////////////////////
7 Lara Eduardo 19980110 5 20210505 20210707 True Jimenes Paco 20000808 1 20210208 0 False Mendez Juanito 19890110 7 20210420 20220520 True Alvarez Ricardo 19970102 10 20200517 20211012 True Mota Jorge 19950505 4 20200707 20200801 True Rodriguez Meny 19670228 5 20210228 20210320 True Salaz Julian 19500505 8 20210405 0 False
1. The 4th constructor that builds an instance of an EPProfile based on the content of a file. 2. Method print, which prints all profiles in the ProfileDB, one by one sequentially. o Note: be careful to print out the content of the EPProfile objects, not their addresses. 3. Method moveDoneToEnd, which moves all profiles of ProfileDB of patients who have already received their second dose to the end of the array. o As a result of applying this method, ProfileDB is organized as follows: all profiles waiting for their second dose at the beginning of the order (in no other particular order) and all profiles who had already received their second dose at the end of the array (in no other particular order). . This method returns an int that represents the number of people who have already been vaccinated twiceStep by Step Solution
There are 3 Steps involved in it
Step: 1
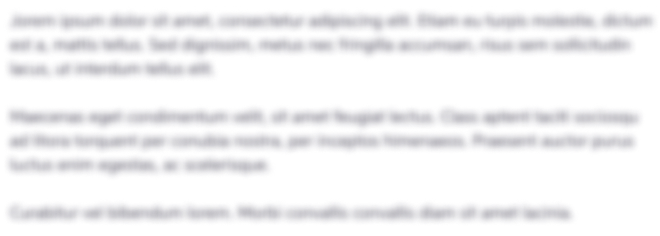
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started