Question
public class LinkedList { // Defined Node class private class Node { private Object Data = null; private Node Next = null; public Node() {
public class LinkedList {
// Defined Node class
private class Node { private Object Data = null; private Node Next = null; public Node() { Data = null; Next = null; } public Node(Object element) { Data = element; } public Node(Object o, Node n) { Data = o; Next = n; } public void setNext(Node n) { Next = n; } public Node getNext() { return Next; } public Object getElement() { return Data; } public void setElement(Object element) { Data = element; } }
// Internal data for LinkedList
private Node head = null;
private Node current = null;
private int size = 0;
// LinkedList constructor
public LinkedList() { head = null; current = head; }
// Move the current position forward one position
public void forward() { current = current.Next; }
// Move the current position backward one position
public void backward() { Node temp = head; while(temp.Next != current) { temp = temp.Next; } current = temp; }
// Get current object's data element
public Object currentData() { return current.Data; }
// Add object to the first of the list
public void addFirst(Object o) { Node newNode = new Node(o); newNode.Next = head; head = newNode; size ++; }
// resetCurrent at the first position
public void resetCurrent() { current = head; }
// Add object to the last of the list
public void addLast(Object o) { Node newNode = new Node(o); if(head == null) { head = newNode; size ++; return; } newNode.Next = null; Node last = head; while (last.Next != null) last = last.Next; last.Next = newNode; size ++; }
// Add an object o before the current position
public void insertBefore(Object o) { Node newNode = new Node(o); Node temp = head, beforeNode=null; while(temp!=null && temp.Next!=newNode){ beforeNode = temp; temp = temp.Next; } }
// Add an object o after the current position
public void insertAfter(Object o) { Node newNode = new Node(o, null); newNode.Next = current.Next; current.Next = newNode; size ++; }
// Get first object
public Object getFirst() { return head.Data; }
// Get last object
public Object getLast() { Node temp = head; while(temp != null) temp = temp.Next; return temp.Data; }
// Remove the first object
public Object removeFirst(){ Node temp = head; head = head.Next; size --; return temp.Data; }
// Remove the last object
public Object removeLast() { Node temp = head; while(temp.Next != null) temp = temp.Next; Node p = temp.Next; temp.Next = null; size --; return p.Data; }
// Remove object o from the list
public void remove(Object o) { Node temp = head, prev = null; // If head node itself holds the key to be deleted if (temp != null && temp.Data == o) { head = temp.Next; // Changed head return; } // Search for the key to be deleted, keep track of the // previous node as we need to change temp.next while (temp != null && temp.Data != o) { prev = temp; temp = temp.Next; } // If key was not present in linked list if (temp == null) return; // Unlink the node from linked list prev.Next = temp.Next; size --; }
// Returns the number of elements on the list
public int size() { return size; }
// Is the list emptied?
public boolean isEmpty() { return size == 0 ? true : false; }
// Display a content of a list
public String toString() { String r = "( HEAD -> "; // Node l = head.getNext(); Node l = head; while (l != null) { r = r + l.getElement() + " -> " ; l = l.getNext(); } return r + " )"; }
public static void main(String args[]) { LinkedList lst = new LinkedList(); // creat instances for testing CsusStudent instance1 = new CsusStudent("John Doe 1", 1, "1 Somewhere", "916-555-1211", "johndoe1@somewhere.com"); CsusStudent instance2 = new CsusStudent("John Doe 2", 2, "2 Somewhere", "916-555-1212", "johndoe2@somewhere.com"); CsusStudent instance3 = new CsusStudent("John Doe 3", 3, "3 Somewhere", "916-555-1213", "johndoe3@somewhere.com"); CsusStudent instance4 = new CsusStudent("John Doe 4", 4, "4 Somewhere", "916-555-1214", "johndoe4@somewhere.com"); CsusStudent instance5 = new CsusStudent("John Doe 5", 5, "5 Somewhere", "916-555-1215", "johndoe5@somewhere.com"); CsusStudent instance6 = new CsusStudent("John Doe 6", 6, "6 Somewhere", "916-555-1216", "johndoe6@somewhere.com"); CsusStudent instance7 = new CsusStudent("John Doe 7", 7, "7 Somewhere", "916-555-1217", "johndoe7@somewhere.com"); CsusStudent instance8 = new CsusStudent("John Doe 8", 8, "8 Somewhere", "916-555-1218", "johndoe8@somewhere.com"); CsusStudent instance9 = new CsusStudent("John Doe 9", 9, "9 Somewhere", "916-555-1219", "johndoe9@somewhere.com"); // begin adding instance1 to the list // test forward and backward for single entry // now add instance2 and instance3 via addFirst and instance4, instance5, instance6 via addLast // move current forward a few times // insert instance 9 after // remove instance9 // print out the first and last entries System.out.println("Show the first entry and last entry:"); // print out the whole list System.out.println("Show the whole list:"); // remove entries starting from the last entry System.out.println("Check for the content of the emptied list"); }
}
-----------------------------------------------------------------------------
public class CsusStudent { private String studentName; private int studentId; private String studentAddress; private String studentPhone; private String studentEmail;
public void CsusStudent(String name, int id, String address, String phone, String email) { // TODO Auto-generated constructor stub name = name; id = id; address = address; phone = phone; email = email; } }
Getting errors LinkedList.java:343: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance1 = new CsusStudent("John Doe 1", 1, "1 Somewhere", "916-555-1211", "johndoe1@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:345: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance2 = new CsusStudent("John Doe 2", 2, "2 Somewhere", "916-555-1212", "johndoe2@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:347: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance3 = new CsusStudent("John Doe 3", 3, "3 Somewhere", "916-555-1213", "johndoe3@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:349: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance4 = new CsusStudent("John Doe 4", 4, "4 Somewhere", "916-555-1214", "johndoe4@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:351: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance5 = new CsusStudent("John Doe 5", 5, "5 Somewhere", "916-555-1215", "johndoe5@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:353: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance6 = new CsusStudent("John Doe 6", 6, "6 Somewhere", "916-555-1216", "johndoe6@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:355: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance7 = new CsusStudent("John Doe 7", 7, "7 Somewhere", "916-555-1217", "johndoe7@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:357: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance8 = new CsusStudent("John Doe 8", 8, "8 Somewhere", "916-555-1218", "johndoe8@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length LinkedList.java:359: error: constructor CsusStudent in class CsusStudent cannot be applied to given types; CsusStudent instance9 = new CsusStudent("John Doe 9", 9, "9 Somewhere", "916-555-1219", "johndoe9@somewhere.com"); ^ required: no arguments found: String,int,String,String,String reason: actual and formal argument lists differ in length 9 errors
Step by Step Solution
There are 3 Steps involved in it
Step: 1
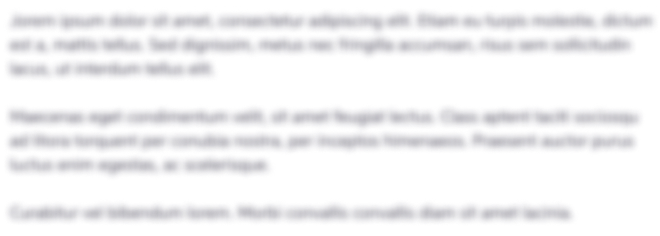
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started