Question
public class Matrix { private int nRow, nCol; private double[] data; // must store as linear array unless 0 //getter for NRow - do not
public class Matrix
{
private int nRow, nCol;
private double[] data; // must store as linear array unless 0
//getter for NRow - do not modify
public int getNRow()
{
return nRow;
}
//getter for NCol - do not modify
public int getNCol()
{
return nCol;
}
/**
* set nRow to r if r is not negative, otherwise set nRow to 0
* @param r: value to be assigned to nRow
*/
public void setNRow(int r)
{
if(r < 0)
nRow = 0;
else
nRow = r;//to be completed
}
/**
* set nCol to c if c is not negative, otherwise set nCol to 0
* @param c: value to be assigned to nCol
*/
public void setNCol(int c)
{
if(c < 0)
nCol = 0;
else
nCol = c;//to be completed
}
/**
*
* @return true if both nRow and nCol are zero, false otherwise
*/
public boolean isEmpty()
{
if(nRow == 0 && nCol ==0)
return true;
else
return false; //to be completed
}
/**
* DO NOT MODIFY
* if arr is null, instance array data should become null,
* otherwise if arr.length is not equal to nRow * nCol,
* set nRow, nCol to 0 and data to null,
* otherwise instantiate instance array data to be of the
* same size as arr. then copy each item of arr into data.
* IMPORTANT: do not re-declare data!
*
* @param arr
*/
public void setData(double[] arr) {
if(arr == null)
data = null;
else {
if(nRow*nCol != arr.length) {
nRow = 0;
nCol = 0;
data = null;
return;
}
data = new double[arr.length];
for(int i=0; i < arr.length; i++) {
data[i] = arr[i];
}
}
}
/**
* Default constructor.
* instance variables nRow and nCol should be set to 0 using the setters.
* the data member data should be set to null
*/
public Matrix()
{
setNRow(0);
setNCol(0);
data = null;//to be completed
}
/**
* Constructor a matrix which has r rows and c columns.
* data member nRow and nCol should be set accordingly using setters.
* AFTER THAT, instance array data should be instantiated to size nRow*nCol
* @param r: number of rows
* @param c: number of columns
*/
public Matrix(int r, int c)
{
setNRow(r);
setNCol(c);
data = new double [r*c];//to be completed
}
/**
*
* @return true if the matrix is a square matrix (a matrix
* for which number of rows and number of columns is the same)
*/
public boolean isSquare() {
if(data.length == nCol * nRow)
return true;
else
return false; //to be completed
}
/**
*
* @param other
* @return true if calling object and parameter object have the same
* dimensions (they both have the same number of rows compared to each
* other, and the same number of columns compared to each other), false otherwise
*/
public boolean sameDimensions(Matrix other)
{
if(nRow == other.nRow)
if(nCol == other.nCol)
return true;
return false; //to be completed
}
/**
* @param r
* @return true if r is a valid row number, false otherwise
* only row numbers 0 to nRow-1 (inclusive on both sides) are valid
*/
public boolean isValidRowNumber(int r) {
if(r >= 0 && r return true; } return false; //to be completed } /** * @param c * @return true if c is a valid column number, false otherwise * only column numbers 0 to nCol-1 (inclusive on both sides) are valid */ public boolean isValidColumnNumber(int c) { if(c >= 0 && c return true; } return false; //to be completed } /** * The constructor must first check that r * c is equal to the length of array d. * if this requirement does not meet, member nRow and nCol should be set to 0 and * data should be set to null. * if the requirement is met, nRow should be set to r and nCol should be set to c. * d should be copied into data using setData(double[] arr) method * @param r: number of rows * @param c: number of columns * @param d: array d to populate data */ public Matrix(int r, int c, double[] d) { //to be completed } /** * The method must check that r and c are valid row numbers and column numbers * respectively. Note that row and column numbers begin with 0. * It should return 0 if r or c is out of range of the dimension of the matrix * @param r * @param c * @return an element of the Matrix at row r and column c */ public double get(int r, int c) { return 0; //to be completed } /** * The method must check that r and c are valid row numbers and column numbers * respectively. Note that row and column numbers begin with 0. * It should return 0 if r or c is out of range of the dimension of the matrix * @param r * @param c * @return an element of the Matrix at row r and column c */ public double get(int r, int c) { return 0; //to be completed }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
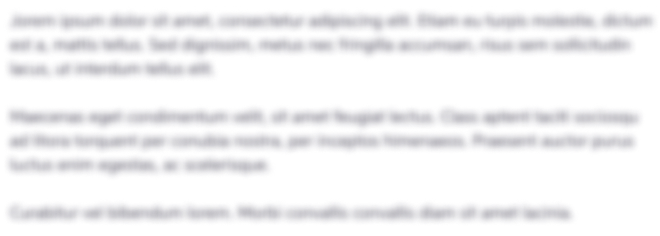
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started