Question
public class MaxHeap
public class MaxHeapextends Comparable super T>> implements HeapInterface { private T[] backingArray; private int size; // Do not add any more instance variables. Do not change the declaration // of the instance variables above. /** * Creates a Heap with an initial capacity of {@code INITIAL_CAPACITY} * for the backing array. * * Use the constant field in the interface. Do not use magic numbers! */ public MaxHeap() { backingArray = (T[]) new Comparable[INITIAL_CAPACITY]; }
/** * Creates a properly ordered heap from a set of initial values. * * The initial array before the Build Heap algorithm takes place should * contain the data in the same order as it appears in the ArrayList. * * The {@code backingArray} should have capacity 2n + 1 where n is the * number of data in the passed in ArrayList (not INITIAL_CAPACITY from * the interface). Index 0 should remain empty, indices 1 to n should * contain the data in proper order, and the rest of the indices should * be empty. * * @param data a list of data to initialize the heap with * @throws IllegalArgumentException if data or any element in data is null */ public buildHeap(ArrayListdata) { if (data == null) { throw new IllegalArgumentException("Cannot insert null data into" + " data structure."); } backingArray = (T[]) new Comparable[INITIAL_CAPACITY * 2 + 1]; int len = data.size(); for (int i = len / 2; i > 0; i--) { upHeap(); } for (int i = 0; i < data.size(); i++) { backingArray[i + 1] = data.get(i); } }
...
I got this error messages
1.
Checking if MaxHeap(ArrayList
2.
Checking if HeapBuild works... (correct organization of heap) Initial: [ null 25 10 30 35 40 53 20 2 99 3 232 222 89 null null null null null null null null null null null null null ] Expected: [ null 232 99 222 35 40 89 20 2 25 3 10 53 30 null null null null null null null null null null null null null ] Actual: [ null 25 10 30 35 40 53 20 2 99 3 232 222 89 null null null null null null null null null null null null null null null null null null null ]
public interface HeapInterfaceextends Comparable super T>> { public static final int INITIAL_CAPACITY = 16; /** * Adds an item to the heap. If the backing array is full and you're trying * to add a new item, then double its capacity. The data passed in will not * be in the heap. Therefore, there will be no duplicates. * * @throws IllegalArgumentException if the item is null * @param item the item to be added to the heap */ public void add(T item); /** * Removes and returns the first item of the heap. Null out all elements not * existing in the heap after this operation. Do not decrease the capacity * of the backing array. * * @throws java.util.NoSuchElementException if the heap is empty * @return the item removed */ public T remove(); /** * Returns if the heap is empty or not. * @return a boolean representing if the heap is empty */ public boolean isEmpty(); /** * Returns the size of the heap. * @return the size of the heap */ public int size(); /** * Clears the heap and returns array to starting capacity. */ public void clear(); /** * Used for grading purposes only. * * DO NOT USE OR EDIT THIS METHOD! * * @return the backing array */ public Comparable[] getBackingArray(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
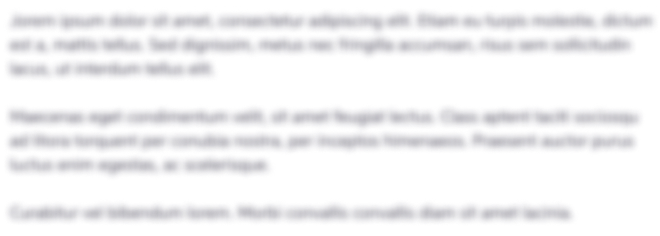
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started