Question
public class MilitaryCargo extends Airplane{ /** *The carrying capacity for missiles */ private int missilePayload; /** * The constructor. * *Takes as input a single
public class MilitaryCargo extends Airplane{
/**
*The carrying capacity for missiles
*/
private int missilePayload;
/**
* The constructor.
*
*Takes as input a single argument, of type int
*@param payload ariplanes carrying capacity for missiles
*/
public MilitaryCargo(int payload){
this.missilePayload = payload;
}
/**
*This getter method retrieves the instance field
*missilePayload
*@return carrying capacity for missiles
*/
public int getMissilePayload(){
return missilePayload;
}
}
public class Seaplane extends Airplane{
private boolean landOnWater;
private double waterDepth;
public Seaplane(double waterDepth){
this.waterDepth = waterDepth;
ableToLand();
}
public boolean getLandOnWater(){
return landOnWater;
}
private void ableToLand(){
if(waterDepth >= 20){
landOnWater = true;
}else{
landOnWater = false;
}
}
}
public class Airplane{
/**The span of the airplane wing*/
private double wingspan;
/**The number of engines on the plane*/
private int numEngines;
/**Is the airplane meant for the military*/
private boolean isForMilitary;
/**
*The constructor.
*
*No argument constructor which sets a default value
*of 50 for the field wingspan.
*/
public Airplane(){
wingspan = 50;
}
/**
*The constructor.
*
*Takes as input a single argument, of type double.
*@param wingspan to set the wingspan of the airplane
*/
public Airplane(double wingspan){
this.wingspan = wingspan;
}
/**
*This getter method retrieves the value of the numEngines
*instance field.
*@return number of engines on the airplane
*/
public int getNumEngines(){
return numEngines;
}
/**
* This setter method sets the value of the numEngines
* instance field.
*
* @param num for the number of engines of the airplane
*/
public void setNumEngines(int num){
numEngines = num;
}
/**
*This getter method retrieves the value of the wingspan
*instance field.
*@return the span of the airplanes wing
*/
public double getWingspan(){
return wingspan;
}
/**
*This getter method retrieves the value of the isForMilitary
*instance field.
*@return wheher the airplane is meant for the military
*/
public boolean getIsForMilitary(){
return isForMilitary;
}
/**
*This method prints out information about the airplane.
*/
protected void info(){
System.out.println("Basic Airplane");
}}
Assume that you are a developer, and you've been given the following UML diagrams, for a parent class (Airplane), and two subclasses (Military Cargo and Seaplane) that both extend the Airplane class. The composite UML diagram can be seen below. It indicates that Military Cargo and Seaplane are derived from Airplane class. Airplane wingspan : double - numEngines : int - for Military : boolean + Airplane + Airplane(wingspan : double) + getNumEngines : int + setNumEngines(num : int) : void + getWingspan (: double + getIsForMilitary : boolean # info() : void Seaplane MilitaryCargo - missilePayload : int landOn Water : boolean - waterDepth : double + MilitaryCargo(payload : int) + getMissilePayload(): int + Seaplane(waterDepth : double) + getLandOn Water(): boolean - ableToLand : void II. Inheritance Explore the UML diagram one more time to get familiar with the classes and methods. Write a new java class named Airport, it should only have the main method. Inside of the main method do the following: Create an object of type MilitaryCargo with the reference variable airplanel. The constructor of the Military Cargo class requires an int value for the field missilePayload, it should be 15. Set the number of engines for airplanel to be 8. Create an object of type Seaplane with the reference variable airplane2. The constructor of the Seaplane class requires a double value for the field waterDepth, it should be 30.0. Set the number of engines for airplane2 to be 2. Create an object of type Airplane with the reference variable airplane3. Use the constructor of the Airplane class which requires a double value for the field wingspan, it should be 100.0. Set the number of engines for airplane3 to be 4. Create an array of type Airplane, named airplanes. Place airplanel, airplane2, and airplane3 into the airplanes array. Using a for loop print out the reference to each airplane, and how many engines it has. The for loop should go through the airplanes array. Use the getter method to retrieve the value of the field numEngines. Check if airplane2 is able to land on water by calling the method getLandOnWater(). Print out if the plane can land or if it should look for a spot where the water is deeperStep by Step Solution
There are 3 Steps involved in it
Step: 1
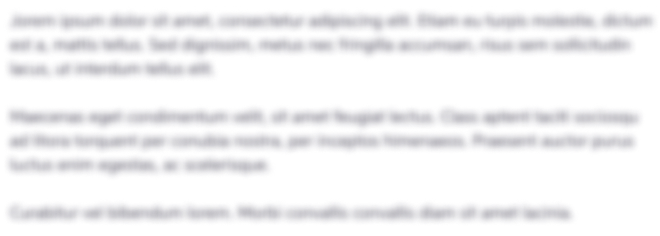
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started