Question
public class myArrayList{ private Object[] buffer; private int currentSize; public myArrayList(){ final int INITIAL_SIZE = 10; buffer = new Object[INITIAL_SIZE]; currentSize=0; } public int size(){
public class myArrayList{
private Object[] buffer;
private int currentSize;
public myArrayList(){
final int INITIAL_SIZE = 10;
buffer = new Object[INITIAL_SIZE];
currentSize=0;
}
public int size(){
return currentSize;
}
private void checkBounds(int n){
if(n < 0 || n>= currentSize){
throw new IndexOutOfBoundsException();
}
}
public Object get(int pos){
checkBounds(pos);
return buffer[pos];
}
public void set(int pos, Object element){
checkBounds(pos);
buffer[pos] = element;
}
public boolean addLast(Object newElement){
growBufferIfNecessary();
currentSize++;
buffer[currentSize - 1] = newElement;
return true;
}
private void growBufferIfNecessary(){
if(currentSize== buffer.length){
Object[] newBuffer = new Object[2 * buffer.length];
for(int i=0; i < buffer.length; i++){
newBuffer[i] = buffer[i];
}
buffer = newBuffer;
}
}
public Object remove(int pos){
checkBounds(pos);
Object removed = buffer[pos];
for(int i = pos + 1; i < currentSize; i++){
buffer[i-1] = buffer[i];
}
currentSize--;
return removed;
}
public static void main(String[] args){
myArrayList list = new myArrayList();
System.out.println(list.size());
list.addLast("King");
list.addLast("King6");
list.addLast("King6");
list.addLast("King4");
list.addLast("King2");
list.addLast("King");
list.addLast("Larry");
list.add(0, "Juliet");
list.remove(1);
for(int i=0; i< list.size(); i++){
System.out.println(list.get(i));
}
//System.out.println(list.get(6));
System.out.println(list.size());
//list.remove(1);
//System.out.println(list.size());
}
}
public class myArrayList{
private Object[] buffer;
private int currentSize;
public myArrayList(){
final int INITIAL_SIZE = 10;
buffer = new Object[INITIAL_SIZE];
currentSize=0;
}
public int size(){
return currentSize;
}
private void checkBounds(int n){
if(n < 0 || n>= currentSize){
throw new IndexOutOfBoundsException();
}
}
public Object get(int pos){
checkBounds(pos);
return buffer[pos];
}
public void set(int pos, Object element){
checkBounds(pos);
buffer[pos] = element;
}
public boolean addLast(Object newElement){
growBufferIfNecessary();
currentSize++;
buffer[currentSize - 1] = newElement;
return true;
}
private void growBufferIfNecessary(){
if(currentSize== buffer.length){
Object[] newBuffer = new Object[2 * buffer.length];
for(int i=0; i < buffer.length; i++){
newBuffer[i] = buffer[i];
}
buffer = newBuffer;
}
}
public Object remove(int pos){
checkBounds(pos);
Object removed = buffer[pos];
for(int i = pos + 1; i < currentSize; i++){
buffer[i-1] = buffer[i];
}
currentSize--;
return removed;
}
public static void main(String[] args){
myArrayList list = new myArrayList();
System.out.println(list.size());
list.addLast("King");
list.addLast("King6");
list.addLast("King6");
list.addLast("King4");
list.addLast("King2");
list.addLast("King");
list.addLast("Larry");
list.add(0, "Juliet");
list.remove(1);
for(int i=0; i< list.size(); i++){
System.out.println(list.get(i));
}
//System.out.println(list.get(6));
System.out.println(list.size());
//list.remove(1);
//System.out.println(list.size());
}
}
error code:
list.add(0, "Juliet");
^
symbol: method add(int,String)
location: variable list of type myArrayList
1 error
I need to define the method?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
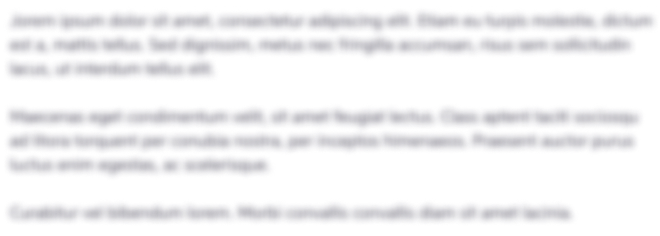
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started