Question
public class Person { // Every person needs, minimally, a name and an age. private String name; private int age; // We can build a
public class Person { // Every person needs, minimally, a name and an age. private String name; private int age;
// We can build a person with a specific name and age with this constructor. public Person(String name, int age) { this.name = name; this.age = age; }
// Since the fields for name and age are private, we need getters and // setters if we want people to be able to change and access them. public String getName() { return this.name; }
public int getAge() { return age; } public void setName(String name){ this.name = name; } /* YOUR CODE HERE * Create setters for name and age somewhere below this comment. * Make sure they are called "setName" and "setAge". * What should they return? What should their parameters be? */ public String toString() { return String.format("%s is %d years old.", name, age); } //NOTE: this main will not run in zyLabs, but you can use this for testing in an IDE public static void main(String[] args) { Person jim = new Person("Jim", 22); System.out.println(jim.toString()); jim.setName("John"); jim.setAge(30); System.out.println(jim.toString()); } }
public class Employee extends Person{ private int id; private Date hireDate; public Employee(Name name, int age, int id, Date hireDate){ super(name,age); // constructor for parent class, Person this.id = id; this.hireDate = hireDate; } public Employee(String first, String last, int age, int id, int year, int month, int dom){ super(new Name(first,last),age); // constructor for parent class, Person this.id = id; this.hireDate = new Date(year,month,dom); } public String toString() { // getName() and getAge() are the getters created in parent class Person return this.getName().toString() + "(" + this.getAge() + ") #"+ this.id + ", hired on "+ this.hireDate.toString(); } public static void main(String[] args) { // create 2 employee objects using the two constructors for class Employee Employee employee1 = new Employee( new Name("Alex","Kean"),23,1001,new Date(2018,2,17)); Employee employee2 = new Employee("Rose","Pike", 18, 1002, 2019, 11, 23); // print the two employees System.out.println(employee1); // toString method invoked automatically System.out.println(employee2); // toString method invoked automatically } }
// Just like an Employee is a specific kind of Person, a Manager is a specific // kind of Employee. public class Manager extends Employee { /* YOUR CODE HERE * At the moment, this constructor is causing errors. * What's wrong with it? Fix it by adding some code. */ public Manager(String name, int age, long salary, String employer) { /* ... fix me! */ }
/* YOUR CODE HERE * This function will properly compile, but it's not very helpful. * We should be putting the name of the person, their company, their age, * etc. instead of just blank spaces and zeros. Fix this toString by * putting relevant information into the string. */ public String toString() { return " " + " is a manager at " + " " + " who is " + 0 + " and makes $" + getSalary() + " a year."; } //NOTE: this main will not run in zyLabs, but you can use this for testing in an IDE public static void main(String[] args) { Manager m = new Manager("Liang", 41, 4_000_000_000L, "Microsoft"); // Perhaps it's unusual that anyone is being paid a salary of four // billion dollars a year, but it's just an example. System.out.println(m.toString()); }
}
import java.util.ArrayList;
public class Polymorphism { public static void main(String[] args) { ArrayList
Person jennifer = new Person("Jennifer", 40); Person michael = new Employee("Michael", 56, 370_000, "Dunder Mifflin"); Person david = new Manager("David", 39, 13_000_000, "Dunder Mifflin");
people.add(jennifer); people.add(michael); people.add(david);
for (int i = 0; i < people.size(); i++) { Person p = people.get(i); System.out.println(p.toString()); }
/* Look at and run the code above, then take a moment to think about the * following questions. Write down your answers somewhere. * * 1. How is it that we were able to store an Employee or a Manager in a * variable that has a type Person? * * 2. When we called the toString() method on each of the people in the * list, it resulted in something totally different. Why? They're all * stored in variables of type Person, so why would they not be the * same? * * 3. We wrote three different versions of toString() across three * different classes. When we call p.toString(), which one of these * versions does Java use, and why? */
// Consider the following code: //michael.setSalary(800000);
/* * 4. Will this code cause errors? Uncomment it and see. * * 5. You should have found that the above code results in a * compilation error. Why can't Java figure out what to do? Employee * has a perfectly valid setSalary method, doesn't it? */
/* Now consider this code: */ Employee anotherMichael = new Employee("Michael", 56, 370000, "Dunder Mifflin");
/* This is the exact same person as above, the only difference is that * we've stored it in a variable of type Employee instead of a variable * of type Person. If we try */
//anotherMichael.setSalary(800000);
/* Will it work? Make a guess, then uncomment the line and see. * * 6. You should have found that this code DOES NOT produce a * compilation error. Why? */
/* 7. What you have just seen in action from the previous few questions * is known as dynamic binding. Describe what dynamic binding is, in * your own words. */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
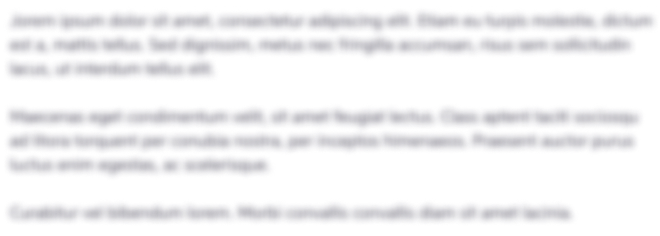
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started