Answered step by step
Verified Expert Solution
Question
1 Approved Answer
public class Point { /** The location of the point in the X direction. */ private double x; /** The location of the point in
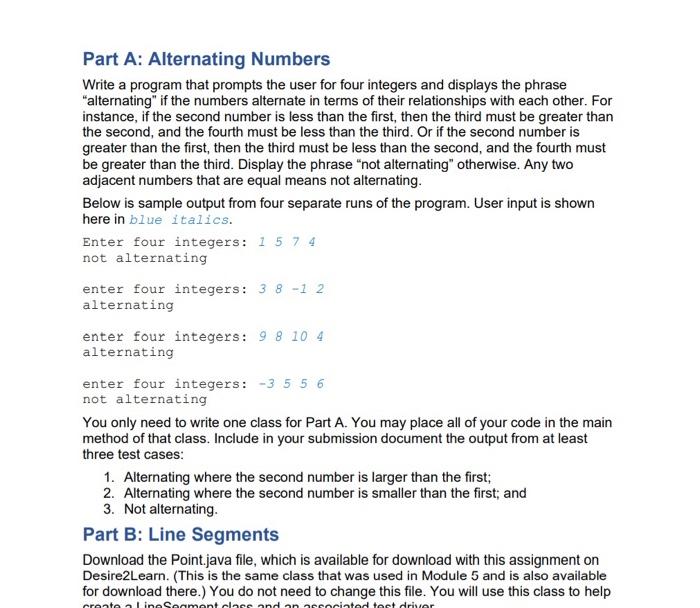
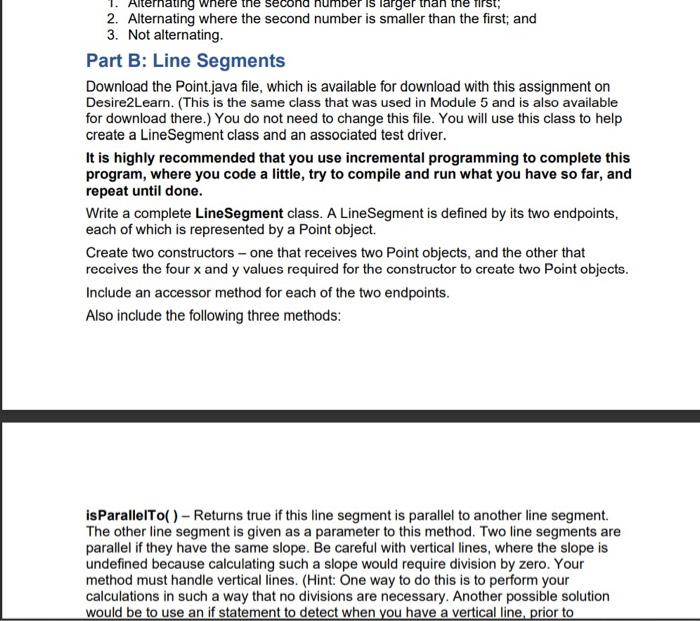
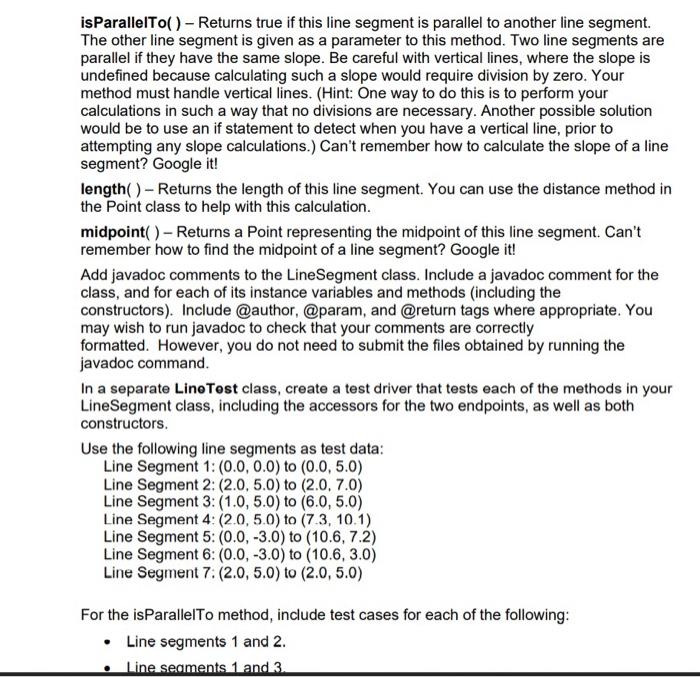
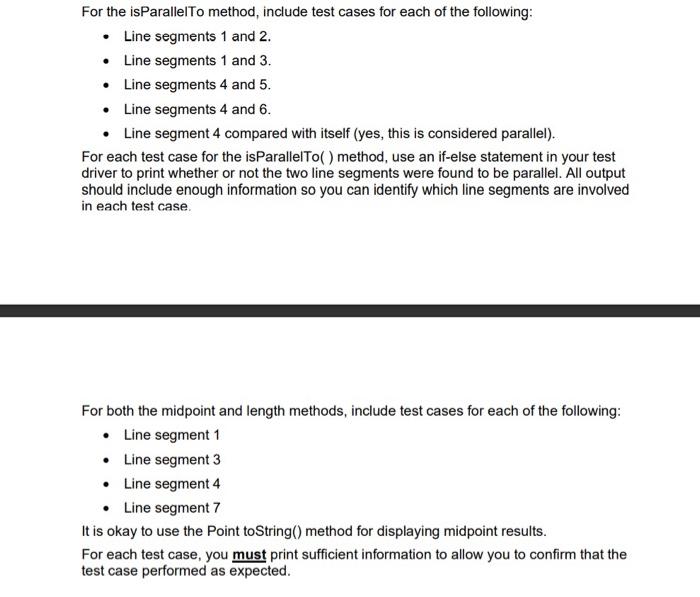
public class Point
{
/** The location of the point in the X direction. */
private double x;
/** The location of the point in the Y direction. */
private double y;
/** Constructs a Point from given x and y values.
@param xIn The location of the Point in the X direction.
@param yIn The location of the Point in the Y direction.
*/
public Point(double xIn, double yIn)
{ x = xIn;
y = yIn;
}
/** Returns the x value for this Point.
@return The x value for this Point.
*/
public double getX()
{ return x;
}
/** Returns the y value for this Point.
@return The y value for this Point.
*/
public double getY()
{ return y;
}
/** Calculates the distance between this Point and
another Point using the pythagorean theorem
@param other The other Point.
@return The distance between this Point and
another Point.
*/
public double distance (Point other)
{ double dx = x - other.getX();
double dy = y - other.getY();
return Math.sqrt(dx*dx + dy*dy);
}
// What is the Math class?
// Math.sqrt is a static method
// Find & use the Java API documentation
// ==> Use 'javac -version'
// ==> Then Google: jdk (your version number) api
// Bookmark that page!
/** Returns a String representation of the x and y values
for this Point.
@return A String representation of the x and y values
for this Point.
*/
public String toString()
{ return "Point[x=" + x + ", y=" + y + "]";
}
/** Changes the x value for this Point.
@param xIn The new x value.
*/
public void setX(double xIn)
{ x = xIn;
}
/** Changes the y value for this Point.
@param yIn The new y value.
*/
public void setY(double yIn)
{ y = yIn;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
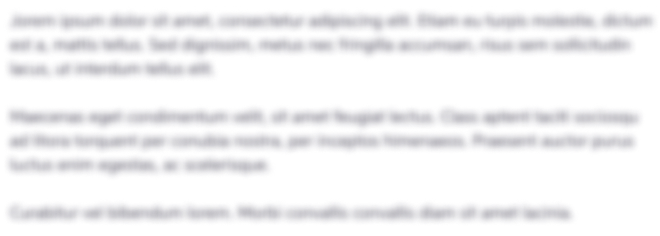
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started