Question
public class RemoveLinkedList { private static LinearNode head; public static void main(String[] args) { // create a linked list that holds 1, 2, ...,5 //
public class RemoveLinkedList {
private static LinearNode
public static void main(String[] args) {
// create a linked list that holds 1, 2, ...,5 // by starting at 5 and adding each node at head of list
head = null; // create empty linked list LinearNode
for (int i = 5; i >= 1; i--) { // create a new node for i intNode = new LinearNode
printElements(); // remove the node storing the value 5 from the list remove(3); printElements(); }
// This method removes from the linked list pointed by head the node // storing the value specified by the second parameter private static void remove(int value) { LinearNode
// Find the node storing value while (current != null) { if (current.getElement().intValue() == value) { // node storing value was found current = null; // Node deleted break; // Exit loop } else { previous = current; current = current.getNext(); } }
}
// Prints the values stored in the list pointed by head private static void printElements() { System.out.print("List of elements: "); LinearNode
------------------------------------------
public class LinearNode
Step by Step Solution
There are 3 Steps involved in it
Step: 1
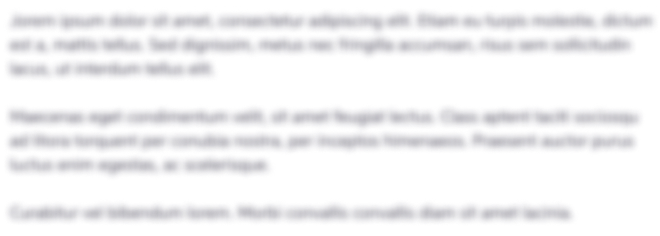
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started