Question
public class SavingAccount { private double annualInterestRate, balance, accumulativeInterest, accumulativeDeposits = 0, accumulativeWithdrawals = 0; public SavingAccount() { balance = 0.0; } public SavingAccount(double balance)
public class SavingAccount
{
private double annualInterestRate, balance, accumulativeInterest,
accumulativeDeposits = 0, accumulativeWithdrawals = 0;
public SavingAccount()
{
balance = 0.0;
}
public SavingAccount(double balance)
{
this.balance = balance;
}
public void addMonthlyInterest()
{
accumulativeInterest += (balance * annualInterestRate / 12);
balance += balance * (annualInterestRate / 12);
}
public double getAnnualInterestRate()
{
return annualInterestRate;
}
public double getBalance()
{
return balance;
}
public double getAccumulativeInterest()
{
return accumulativeInterest;
}
public double getAccumulativeDeposits()
{
return accumulativeDeposits;
}
public double getAccumulativeWithdrawals()
{
return accumulativeWithdrawals;
}
public void setAnnualInterestRate(double annualInterestRate)
{
this.annualInterestRate = annualInterestRate;
}
public void setBalance(double balance)
{
this.balance = balance;
}
public void setAccumulativeInterest(double accumulativeInterest)
{
this.accumulativeInterest = accumulativeInterest;
}
public void setAccumulativeDeposits(double accumulativeDeposits)
{
this.accumulativeDeposits = accumulativeDeposits;
}
public void setAccumulativeWithdrawals(double accumulativeWithdrawals)
{
this.accumulativeWithdrawals = accumulativeWithdrawals;
}
public void withdraw(double w)
{
balance -= w;
accumulativeWithdrawals += w;
}
public void deposit(double d)
{
balance += d;
accumulativeDeposits += d;
}
public double getMonthlyInterest()
{
return balance * (annualInterestRate / 12);
}
}
import java.io.*;
public class Assignment8
{
public static void main(String[] args)
{
final int MONTH_PERIOD = 3;
try
{
// create an InputStreamReader object to read from keyboard
InputStreamReader ir = new InputStreamReader(System.in);
// create BufferedReader object br
BufferedReader br = new BufferedReader(ir);
// create printwriter object to write data to the file result.txt
PrintWriter pw = new PrintWriter(new File("result.txt"));
double startingBalance, interestRate;
System.out.print("Enter the starting balance on the account: $");
startingBalance = Double.parseDouble(br.readLine());
SavingAccount savingAccount = new SavingAccount(startingBalance);
System.out.print("Enter the anual interest rate on the account: $");
interestRate = Double.parseDouble(br.readLine());
savingAccount.setAnnualInterestRate(interestRate);
for (int count = 1; count <= MONTH_PERIOD; count++) {
System.out.println(" MONTH " + count + "");
// read the amount to deposit to balance
System.out.printf("Total deposits for this month: $");
savingAccount.deposit(Double.parseDouble(br.readLine()));
// read the amount to withdraw from balance from the console
System.out.printf("Total withdrawals for this month: $");
savingAccount.withdraw(Double.parseDouble(br.readLine()));
savingAccount.addMonthlyInterest();
}
System.out.println(" Quaterly Savings Account Statement");
System.out.printf(" Starting balance:\t$ %.2f", startingBalance);
System.out.printf(" Total deposits:\t\t+ $%.2f",
savingAccount.getAccumulativeDeposits());
System.out.printf(" Total withdrawals:\t- $%.2f",
savingAccount.getAccumulativeWithdrawals());
System.out.printf(" Total interest:\t\t+ $%.2f",
savingAccount.getAccumulativeInterest());
System.out.println(" \t\t\t----------");
System.out.printf(" Ending balance:\t\t $%.2f",
savingAccount.getBalance());
//writing data to file
pw.write(" Starting balance:\t$ " + startingBalance);
pw.write(" Total deposits:\t\t+ $"
+ savingAccount.getAccumulativeDeposits());
pw.write(" Total withdrawals:\t- $"
+ savingAccount.getAccumulativeWithdrawals());
pw.write(" Total interest:\t\t+ $"
+ savingAccount.getAccumulativeInterest());
pw.write(" \t\t\t----------");
pw.write(" Ending balance:\t\t $" + savingAccount.getBalance());
pw.flush();
}
catch (Exception e)
{
}
}
}
Using the test case:
5000 0.035 780.5 472.75 550.25 510 729 350.65
//////////////////////////////////////////////////////////////////////////////
The output is supposed to be:
Quarterly Savings Account Statement Starting balance: $ 5000.00 Total deposits: + $ 2059.75 Total withdrawals: - $ 1333.40 Total interest: + $ 46.86 ---------- Ending balance: $ 5773.21
////////////////////////////////////////////////////////////////////////////
But Im getting
///////////////////////////////////////////////////////////////////////////
Quaterly Savings Account Statement Starting balance: $ 5000.00 Total deposits: + $2059.75 Total withdrawals: - $1333.40 Total interest: + $47.92 ---------- Ending balance: $5774.27
//////////////////////////////////////////////////////////////////////
Can you help me fix the Total interest?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
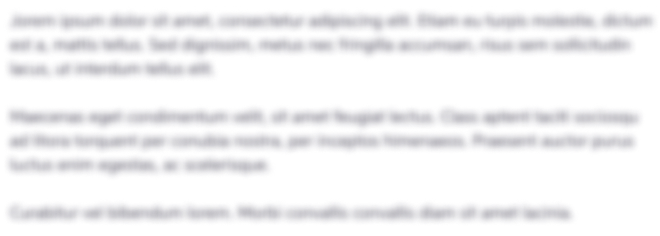
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started