Question
public class SetDictionary > implements SetInterface , Iterable { private TreeMap items; public SetDictionary() { // TODO Project 1 } // end default constructor public
public class SetDictionary
{ private TreeMapitems; public SetDictionary() { // TODO Project 1 } // end default constructor public boolean add(K newEntry) { // TODO Project 1 return false; } // end add public boolean remove(K anEntry) { // TODO Project 1 return false; } // end remove public void clear() { // TODO Project 1 } // end clear public boolean contains(K anEntry) { // TODO Project 1 return false; } // end contains public int getCurrentSize() { // TODO Project 1 return 0; } // end getCurrentSize public boolean isEmpty() { // TODO Project 1 return false; } // end isEmpty public boolean equals(Object o) { // TODO Project 1 return false; } // equals public Iterator getIterator() { // TODO Project 1 return null; } // end getIterator public Iterator iterator() { // TODO Project 1 return null; } // end iterator public K[] toArray() { // TODO Project 1 // the cast is safe because the new array contains null entries @SuppressWarnings("unchecked") K[] result = (K[]) new Comparable[getCurrentSize()]; // unchecked cast //MUST BE IMPLEMENTED WITH ITERATOR return result; } // end toArray public SetInterface union(SetInterface otherSet) { // TODO Project 1 SetInterface result = new SetDictionary(); //MUST BE IMPLEMENTED WITH ITERATORS USING forEach lambda CONSTRUCT // AS SHOWN IN LectureDictionary EXAMPLES return result; } // end union public SetInterface intersection(SetInterface otherSet) { // TODO Project 1 SetInterface result = new SetDictionary(); //MUST BE IMPLEMENTED WITH ITERATORS // UTILIZE TRY_CATCH BLOCK // try // { // while (true) // { // // // // // // } // end while // } catch (NoSuchElementException nsee) // { // System.out.println("Finished creating intersection."); // } return result; } // end intersection public static void main(String args[]) { System.out.println("CREATING set1"); SetInterface set1 = new SetDictionary(); set1.add(1); set1.add(3); set1.add(2); set1.add(0); set1.add(-1); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> contains for -1 yields " + set1.contains(-1)); System.out.println("--> contains for -2 yields " + set1.contains(-2)); System.out.println("--> contains for 3 yields " + set1.contains(3)); System.out.println("--> contains for 4 yields " + set1.contains(4)); set1.add(1); System.out.println(" --> Added 1 again to the set1, should be the same"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> Iterating over set1 utilizing getIterator method"); Iterator kIter = set1.getIterator(); while (kIter.hasNext()) { System.out.println("\t" + kIter.next()); } // end while System.out.println("--> Iterating over set1 utilizing iterator method"); kIter = ((SetDictionary ) set1).iterator(); while (kIter.hasNext()) { System.out.println("\t" + kIter.next()); } // end while System.out.println("--> Iterating over set1 utilizing forEach lambda"); ((SetDictionary ) set1).items.forEach((k,v) -> System.out.println("\t " + k)); System.out.println(" --> Removing -1 20 3 from set1:"); boolean result = set1.remove(-1); if (result) System.out.println("---> -1 was removed - CORRECT"); else System.out.println("---> -1 was not removed - INCORRECT"); result = set1.remove(20); if (result) System.out.println("---> 20 was removed - INCORRECT"); else System.out.println("---> 20 was not removed - CORRECT"); result = set1.remove(3); if (result) System.out.println("---> 3 was removed - CORRECT"); else System.out.println("---> 3 was not removed - INCORRECT"); System.out.println("--> Should just have 0 1 and 2 now"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("CREATING set2"); SetInterface set2 = new SetDictionary(); set2.add(1); set2.add(3); set2.add(2); set2.add(-1); set2.add(5); set2.add(8); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); if (set1.equals(set2)) System.out.println("set1 and set2 are the same - INCORRECT"); else System.out.println("set1 and set2 are NOT the same - CORRECT"); System.out.println(); System.out.println("CREATING UNION OF set1 and set2"); SetInterface testUnion = set1.union(set2); System.out.print("--> The union of set1 and set2 has " + testUnion.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testUnion.toArray())); System.out.println(); System.out.println("--> set1 should be unchanged"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> set2 should be unchanged"); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); System.out.println("CREATING UNION OF set1 and set1"); testUnion = set1.union(set1); System.out.print("--> The union of set1 and set1 has " + testUnion.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testUnion.toArray())); System.out.println(); if (set1.equals(testUnion)) System.out.println("set1 and testUnion are the same - CORRECT"); else System.out.println("set1 and testUnion are NOT the same - INCORRECT"); System.out.println(); System.out.println("CREATING INTERSECTION OF set1 and set2"); SetInterface testIntersection = set1.intersection(set2); System.out.print("--> The intersection of set1 and set2 has " + testIntersection.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testIntersection.toArray())); System.out.println(); System.out.println("--> set1 should be unchanged"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> set2 should be unchanged"); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); System.out.println("CREATING INTERSECTION OF set2 and set1"); testIntersection = set2.intersection(set1); System.out.print("--> The intersection of set2 and set1 has " + testIntersection.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testIntersection.toArray())); System.out.println(); System.out.println("--> set1 should be unchanged"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> set2 should be unchanged"); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); System.out.println("CREATING INTERSECTION OF set2 and set2"); testIntersection = set2.intersection(set2); System.out.print("--> The intersection of set2 and set2 has " + testIntersection.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testIntersection.toArray())); System.out.println(); System.out.println("--> set1 should be unchanged"); System.out.println("--> set1 has " + set1.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set1.toArray())); System.out.println(); System.out.println("--> set2 should be unchanged"); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); if (set2.equals(testIntersection)) System.out.println("set2 and testIntersection are the same - CORRECT"); else System.out.println("set2 and testIntersection are NOT the same - INCORRECT"); System.out.println(); System.out.println("CREATING INTERSECTION OF testUnion and set2"); testIntersection = testUnion.intersection(set2); System.out.print("--> The intersection of testUnion and set2 has " + testIntersection.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testIntersection.toArray())); System.out.println(); System.out.println("--> testUnion should be unchanged"); System.out.println("--> testUnion has " + testUnion.getCurrentSize() + " items: "); System.out.println(Arrays.toString(testUnion.toArray())); System.out.println(); System.out.println("--> set2 should be unchanged"); System.out.println("--> set2 has " + set2.getCurrentSize() + " items: "); System.out.println(Arrays.toString(set2.toArray())); System.out.println(); if (set2.equals(testIntersection)) System.out.println("set2 and testIntersection are the same - INCORRECT"); else System.out.println("set2 and testIntersection are NOT the same - CORRECT"); System.out.println(); } // end main }
Sample run of the program:
CREATING set1
--> set1 has 5 items:
[-1, 0, 1, 2, 3]
--> contains for -1 yields true
--> contains for -2 yields false
--> contains for 3 yields true
--> contains for 4 yields false
--> Added 1 again to the set1, should be the same
--> set1 has 5 items:
[-1, 0, 1, 2, 3]
--> Iterating over set1 utilizing getIterator method
-1
0
1
2
3
--> Iterating over set1 utilizing iterator method
-1
0
1
2
3
--> Iterating over set1 utilizing forEach lambda
-1
0
1
2
3
--> Removing -1 20 3 from set1:
---> -1 was removed - CORRECT
---> 20 was not removed - CORRECT
---> 3 was removed - CORRECT
--> Should just have 0 1 and 2 now
--> set1 has 3 items:
[0, 1, 2]
CREATING set2
--> set2 has 6 items:
[-1, 1, 2, 3, 5, 8]
set1 and set2 are NOT the same - CORRECT
CREATING UNION OF set1 and set2
--> The union of set1 and set2 has 7 items: [-1, 0, 1, 2, 3, 5, 8]
--> set1 should be unchanged
--> set1 has 3 items:
[0, 1, 2]
--> set2 should be unchanged
--> set2 has 6 items:
[-1, 1, 2, 3, 5, 8]
CREATING UNION OF set1 and set1
--> The union of set1 and set1 has 3 items: [0, 1, 2]
set1 and testUnion are the same - CORRECT
CREATING INTERSECTION OF set1 and set2
Finished creating intersection.
--> The intersection of set1 and set2 has 2 items: [1, 2]
--> set1 should be unchanged
--> set1 has 3 items:
[0, 1, 2]
--> set2 should be unchanged
--> set2 has 6 items:
[-1, 1, 2, 3, 5, 8]
I. Implement ADT set Problem Description We want to implement the ADT set. Recall from Chapter 1 that a set is an unordered collection of objects where duplicates are not allowed. The operations that a set should support are: Add a given object to the set (will always return true) Remove a given object from the set . See whether the set contains a given object Clear all objects from the set Get the number of objects in the set Return an iterator to the set Return a set that combines the items in two sets (the union) Return a set of those items that occur in both of two sets (the intersection). The algorithm must take advantage of the fact that both lists are sorted These operations are defined in the provided SetInterface. Implement the class SetDictionary.java that uses a dictionary internally (instance of class TreeMap) to implement the SetInterface (the skeleton of the SetDictionary.java class is provided, and it contains main that you can use to test your methods). The key is the item, the value is a boolean set to true. I. Implement ADT set Problem Description We want to implement the ADT set. Recall from Chapter 1 that a set is an unordered collection of objects where duplicates are not allowed. The operations that a set should support are: Add a given object to the set (will always return true) Remove a given object from the set . See whether the set contains a given object Clear all objects from the set Get the number of objects in the set Return an iterator to the set Return a set that combines the items in two sets (the union) Return a set of those items that occur in both of two sets (the intersection). The algorithm must take advantage of the fact that both lists are sorted These operations are defined in the provided SetInterface. Implement the class SetDictionary.java that uses a dictionary internally (instance of class TreeMap) to implement the SetInterface (the skeleton of the SetDictionary.java class is provided, and it contains main that you can use to test your methods). The key is the item, the value is a boolean set to trueStep by Step Solution
There are 3 Steps involved in it
Step: 1
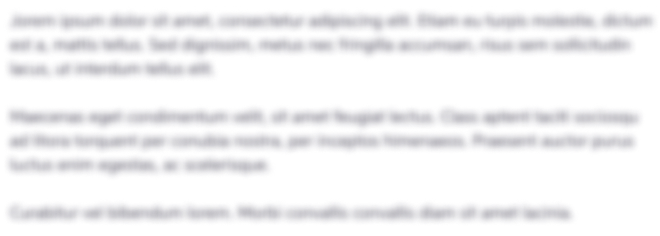
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started