Question
public class SweetMillionGame { // constants specific to current game - BUT NOT ALL GAMES public final static int DEFAULT_GAME_COUNT = 1; private final static
public class SweetMillionGame {
// constants specific to current game - BUT NOT ALL GAMES
public final static int DEFAULT_GAME_COUNT = 1;
private final static String GAME_NAME = "Sweet Million";
private final static int SELECTION_POOL_SIZE = 40;
public final static int SELECTION_COUNT = 6;
private int val_array[][] = null;
private int gameCount;
private Randomizer randomizer;
public SweetMillionGame() {
init(DEFAULT_GAME_COUNT);
}
public SweetMillionGame(int games) {
init(games);
}
private void init(int games) {
randomizer = new Randomizer();
/**
*
* Now what ... START FROM HERE What additional methods do you need?
* What additional data properties/members do you need?
*/
gameCount = games;
val_array = new int[games][SELECTION_COUNT];
for (int i = 0; i
for (int j = 0; j
val_array[i][j] = (int) randomizer.returnRandomNumber(SELECTION_POOL_SIZE);
}
Arrays.sort(val_array[i]);
}
}
/**
*
*/
public void displayTicket() {
/**
* display heading
*
* for i in gameCount generate selectionCount number of unique random
* selections in ascending order
*
* display footer
*/
// display ticket heading
displayHeading();
/**
* Display selected numbers
*/
for (int i = 0; i
System.out.printf("(%2d)\t", i + 1);
for (int j = 0; j
System.out.printf("%02d ", val_array[i][j]);
}
System.out.println("");
}
System.out.println("");
// display ticket footer
displayFooter();
return;
}
protected void displayHeading() {
System.out.println("---------------------------------------");
System.out.println("");
System.out.println("------------ " + GAME_NAME + " ------------");
System.out.println("");
Format formatter = new SimpleDateFormat(" EEE MMM dd HH:mm:ss z yyyy");
System.out.println(formatter.format(new Date()));
System.out.println("");
}
protected void displayFooter() {
System.out.println("-------- (c) S.I. Corner Deli ---------");
}
/**
* @param args
*/
public static void main(String[] args) {
// takes an optional command line parameter specifying number of QP games to be generated
// By default, generate 1
int numberOfGames = 1;
if (args.length > 0) {
numberOfGames = Integer.parseInt(args[0]);
}
SweetMillionGame game = new SweetMillionGame(numberOfGames);
// now what
game.displayTicket();
System.out.println("Leaving ...");
}
}
home / study / engineering / computer science / computer science questions and answers / assignment: making our sweetmillion solution much more flexible (100 pts) focus custom ...
Question: Assignment: Making our SweetMillion Solution much more flexible (100 pts) Focus Custom Exce...
Assignment: Making our SweetMillion Solution much more flexible (100 pts)
Focus Custom Exceptions and Configurability.
This programming assignment revolves around embellishing the design and implementation of Lab 3. Our requirement for Lab 3 drove us to implement a Class called SweetMillion that represented the popular NYS lotto game of the same name. Your solution leveraged the services of another Class Randomizer and was hopefully as modular as possible, but our solution was intended to be fairly fixed. That is, it modeled the rules of only 1 game.
|
Imagine that NYS was very thrilled with your SweetMillion implementation, and offered you a chance to build a Quick Picker Solution for all games both present and future. How would you go about re-factoring your SweetMillion solution into one that covered our new requirements?
One possible approach might look like this.
|
That is, you might have an abstract base Class of sorts that allows you to share common data and methods with game specific sub-classes.
This is a reasonable approach, but can it be better - more flexible and easier to maintain?
First, what ongoing changes would you be responsible for?
New game implementations.
Rule modifications to existing games.
NOTE: all games forces our implementations to include 2 pools of numbers rather than just one as many NYS lotto games pick N numbers from a primary pool and N supplementary numbers.
What if we could build a single Class solution that would support ALL games present and future? - one that made use of configuration files (property files) to drive the specific of each game.
What would such a solution look like?
|
The model above could rely on a collection of Java properties files. Each properties file would dictate the rules of one Lotto Game.
A properties file with the following format has all information required to cover all game behavior details.
Example 1
################################################### ## Pick10 game definition - pick 10 in 80 numbers GameName=Pick 10! Pool1=10/80 Pool2=0/0 Vendor=CSC330 Corner Store |
Example 2
################################################### ## Mega Million game definition - pick 5 in 75 and then 1 in 15 GameName=MegaMillions! Pool1=5/75 Pool2=1/15 Vendor=CSC330 Corner Store |
Your assignment:
1)(Implement a solution that would NOT require us to modify any Java code in order to support an evolving Lotto Game set. One that supports the design suggested above where:
A new game would require you to add a new .properties file to your deployment.
Updated rules to an existing game (or a Name Change) would require you to modify an existing .properties file.And thus, unless something very fundamental changes about the way NYS Lotto works, you would never need to modify, recompile and re-test your code base.
This can clearly be called a Configurable solution!
(2) Create a custom Exception called QuickPickerException that will be throw by your QuickPicker Class if one of the conditions occur.
The specified game does not exist (missing .properties file)
A required property is missing from the specified .properties file.
You need to implement the following Classes:
QuickPicker implements the behavior of a generic QuickPicker solution that depends on the details found in a game specific .properties file everything needed to provide the same functionality that your SweetMillion solution provided.
QuickPickerException needs to extend the Exception Class. Use the edu.cuny.csi.csc330.newradio.RadioException Class as a solid point of reference / template.
Program Arguments: Your QuickPicker Class will require 1 argument and accept an second optional argument:
The name of the Game to be played. Name must match the name of the properties file e.g., a file called MegaMillions.properties must exist to support a 1st argument of MegaMillions
The number of bets/games to be generated. Like your SweetMillion solution, the default number of games should be 1.
SweetMillion RandomizerStep by Step Solution
There are 3 Steps involved in it
Step: 1
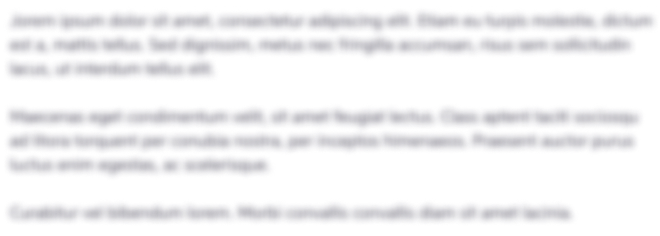
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started