Question
{ public enum Suit { Clubs, Diamonds, Hearts, Spades }; public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack,
{ public enum Suit { Clubs, Diamonds, Hearts, Spades }; public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King }; // ----------------------------------------------------------------------------------------------------- public class Card { public Rank Rank { get; private set; } public Suit Suit { get; private set; } public Card(Suit suit, Rank rank) { this.Suit = suit; this.Rank = rank; } public override string ToString() { return ("[" + Rank + " of " + Suit + "]"); } } // ----------------------------------------------------------------------------------------------------- public class Deck { private Card[] cards; private static Random rng = new Random(); // static helps prevent duplicate rng's public Deck() { Array suits = Enum.GetValues(typeof(Suit)); Array ranks = Enum.GetValues(typeof(Rank)); int size = suits.Length * ranks.Length; cards = new Card[size]; int i = 0; foreach (Suit suit in suits) { foreach (Rank rank in ranks) { Card card = new Card(suit, rank); cards[i++] = card; } } } public int Size() { return cards.Length; } public void Shuffle() { if (Size() == 0) return; // Cannot shuffle an empty deck // Fisher-Yates Shuffle (modern algorithm) // - http://en.wikipedia.org/wiki/Fisher%E2%80%93Yates_shuffle for (int i = 0; i < Size(); i++) { int j = rng.Next(i, Size()); Card c = cards[i]; cards[i] = cards[j]; cards[j] = c; } } public void Cut() { if (Size() == 0) return; // Cannot cut an empty deck int cutPoint = rng.Next(1, Size()); // Cannot cut at zero Card[] newDeck = new Card[Size()]; int i; int j = 0; // Copy the cards at or below the cutpoint into the top of the new deck for (i = cutPoint; i < Size(); i++) { newDeck[j++] = cards[i]; } // Copy the cards above the cutpoint into the bottom of the new deck for (i = 0; i < cutPoint; i++) { newDeck[j++] = cards[i]; } cards = newDeck; } public Card DealCard() { if (Size() == 0) return null; Card card = cards[Size() - 1]; // Deal from bottom of deck (makes Resizing easier) Array.Resize(ref cards, Size() - 1); return card; } public override string ToString() { string s = "["; string comma = ""; foreach (Card c in cards) { s += comma + c.ToString(); comma = ", "; } s += "]"; s += " " + Size() + " cards in deck. "; return s; } } 1.) Use the Card and Deck classes from here to get started:
2.) You Main() method will be where the game simulation takes place. It will need to have a loop that continues until the game is over. Each time through the loop represents another player's turn.
3.) Each time a player does anything, a message needs to be printed out on the console describing what the player did. After the program finishes, the console should have a complete record of everything that happened during the game.
4.) Different players will be using different rank-picking strategies during the simulation. That means we need to use polymorphism to implement all the different types of players. Start by adding the following abstract Player class in your program:
public abstract class Player { public string Name { get; private set; } public Hand Hand { get; private set; } // Other Properties/member variables go here public Player(string name) { this.Name = name; this.Hand = new Hand(); } public abstract Player ChoosePlayerToAsk(Player[] players); public abstract Rank ChooseRankToAskFor(); // Other Player methods go here public override string ToString() { string s = Name + "'s Hand: "; s += Hand.ToString(); return s; } }
5) Do not modify the code above. You can (and should) add more methods and member variables into that class, but you do not need to modify any of the code above.
6.) First, create a subclass of the Player class for players that choose things randomly.
7.) Next this first version of the game working using four of your "random strategy" players.
8.) Now, in addition to the "random strategy" player, create three additional types (i.e., subclasses) of players that use the following different strategies:
A player that always chooses the first card in their hand and the first player on their right.
A player that always chooses the last card in their hand and the first player on their left.
A player that always chooses the last card in their hand but asks a random player.
9.) Run your program lots of times and see which strategy works best.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
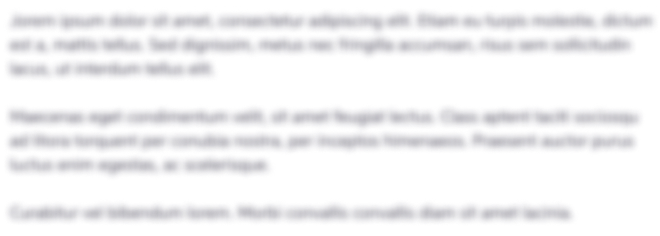
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started