Question
public interface Polynomial { /** * Returns coefficient of the term with 'power' * @param power * @return */ public double coefficient(int power); /** *
public interface Polynomial {
/**
* Returns coefficient of the term with 'power'
* @param power
* @return
*/
public double coefficient(int power);
/**
* Returns the degree of polynomial (i.e. largest power of a non-zero term)
* @return
*/
public int degree();
/**
* Add a term to the polynomial
* @param power
* @param coefficient
* @throws Exception when the power is invalid (e.g. negative)
*/
public void addTerm(int power, double coefficient) throws Exception;
/**
* Remove the term corresponding to the power from the polynomial
* @param power
*/
public void removeTerm(int power);
/**
* Returns evaluation of the polynomial at 'point'
* @param point
* @return
*/
public double evaluate(double point);
/**
* Returns a new polynomial that is the result of addition of polynomial p to
this polynomial
* @param p
* @return
*/
public Polynomial add(Polynomial p);
/**
* Returns a new polynomial that is the result of subtraction of polynomial p
to this polynomial
* @param p
* @return
*/
public Polynomial subtract(Polynomial p);
/**
* Returns a new polynomial that is the result of multiplication of polynomial
p with this polynomial
* @param p
* @return
*/
public Polynomial multiply(Polynomial p);
}
Polynomial ADT In this assignment, you will be implementing an ADT for performing polynomial operations. Recall a polynomial p(x) is given by sum of terms where each term is a product of a non-zero real number coefficient and a non-negative integer power of x. For example, -5x5 + 2.5x4 3x2 + 2 is a polynomial; it is of degree 5 as it is the highest power in the polynomial. You are given a Java interface for the ADT called Polynomial.java. Java interfaces contain only method declarations. They are implemented by specific classes that implement the interfaces. Note that in any polynomial implementation you just need to keep information regarding the coefficients and the powers of the polynomial. You need to write a class called ArrayPolynomial.java which provides one specific implementation of the Polynomial interface. In this implementation you will be using an array to store the coefficients where the k-th position in the array contains coefficient of xk in the polynomial. It will be zero if xk term does not exist in the polynomial. We will be providing a starter file for ArrayPolynomial.java for your lab session later this week when you will be asked to implement some specific things. You can start working on this assignment prior to the lab but you should merge your implementation file with this file prior to working on the lab assignmentStep by Step Solution
There are 3 Steps involved in it
Step: 1
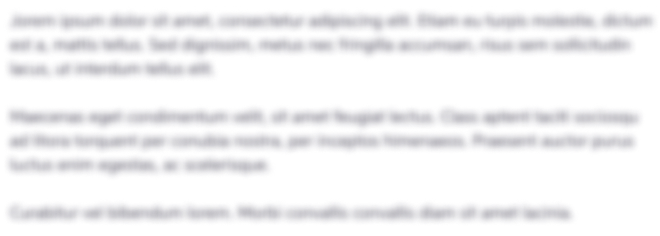
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started